Removing whitespace from strings in Java
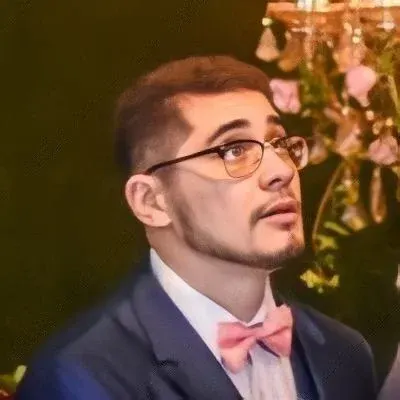

π₯πRemoving Whitespace from Strings in Javaππ₯
Hi there! Have you ever found yourself in a situation where you needed to remove whitespace from a string in Java, but the regular solutions just didn't work? Don't worry, I've got you covered! In this blog post, I am going to show you how to tackle this problem head-on and provide you with some easy and effective solutions. So, let's dive right in! πͺ
The Problem π€
You have a string like this:
String mysz = "name=john age=13 year=2001";
And you want to remove the whitespaces within the string, so it becomes:
String mysz2 = "name=johnage=13year=2001";
The Failed Attempts π
You've tried using the trim()
method, which only removes leading and trailing whitespaces, not the ones within the string. Then, you attempted using replaceAll("\\W", "")
, which almost worked, but unfortunately, it also removed the equals sign (=
) along with the whitespaces.
The Clever Solution βοΈ
Fear not! There's a clever solution to achieve your desired output.
String mysz = "name=john age=13 year=2001";
String mysz2 = mysz.replace(" ", "");
By using the replace()
method, you can replace all occurrences of a specific substring (in this case, the whitespace) with an empty string. This will result in a new string, mysz2
, without any whitespaces.
More Flexibility with Regular Expressions β¨
What if there are more types of whitespaces, such as tabs or line breaks, within the string? Or what if the whitespaces occur more than once consecutively? Don't worry, regular expressions to the rescue! π¦ΈββοΈ
You can modify the replace()
method to use a regular expression pattern instead of a simple substring:
String mysz = "name=john age=13 year=2001";
String mysz2 = mysz.replaceAll("\\s+", "");
In this case, the regular expression pattern \\s+
matches one or more whitespaces (\\s
) consecutively (+
). It's a powerful way to remove any kind of whitespace, regardless of its type or frequency within the string.
Wrap Up and Call-to-Action π
And there you have it, a simple and effective solution to remove whitespace from strings in Java! Now, you can confidently manipulate your strings without any unwanted spaces. π
If you found this blog post helpful or have any questions, feel free to leave a comment below. I'd love to hear from you! And don't forget to share this post with your fellow developers who might be struggling with this issue too! Sharing is caring! β€οΈ
Happy coding! π©βπ»π
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
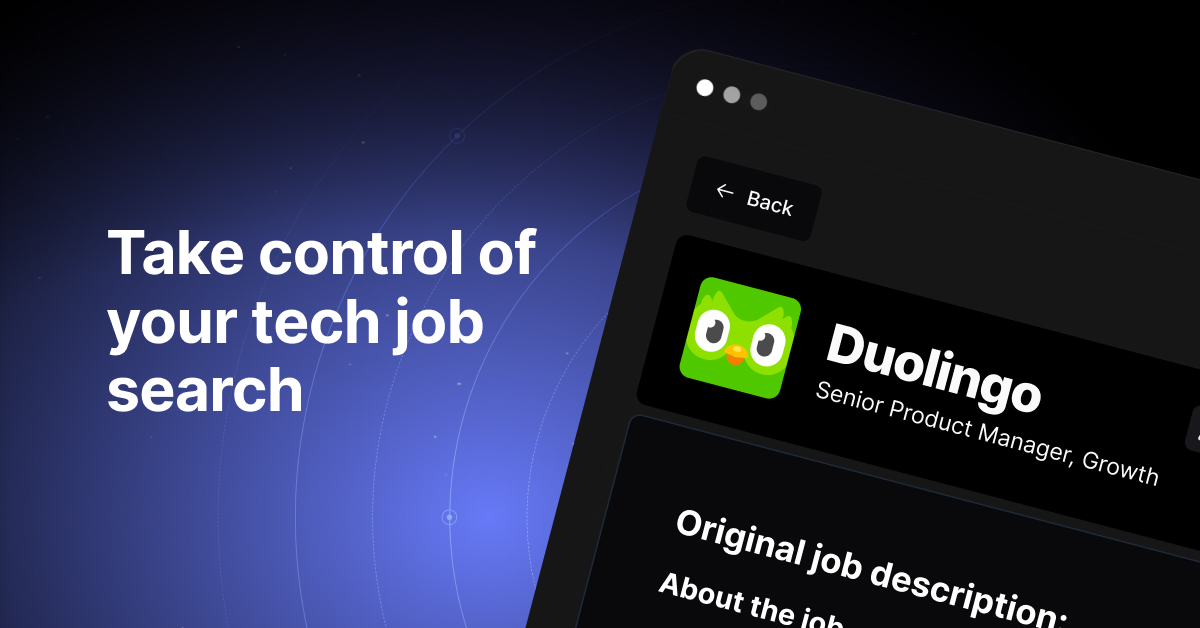