Remove HTML tags from a String
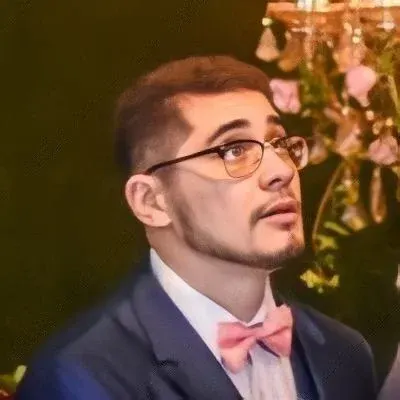
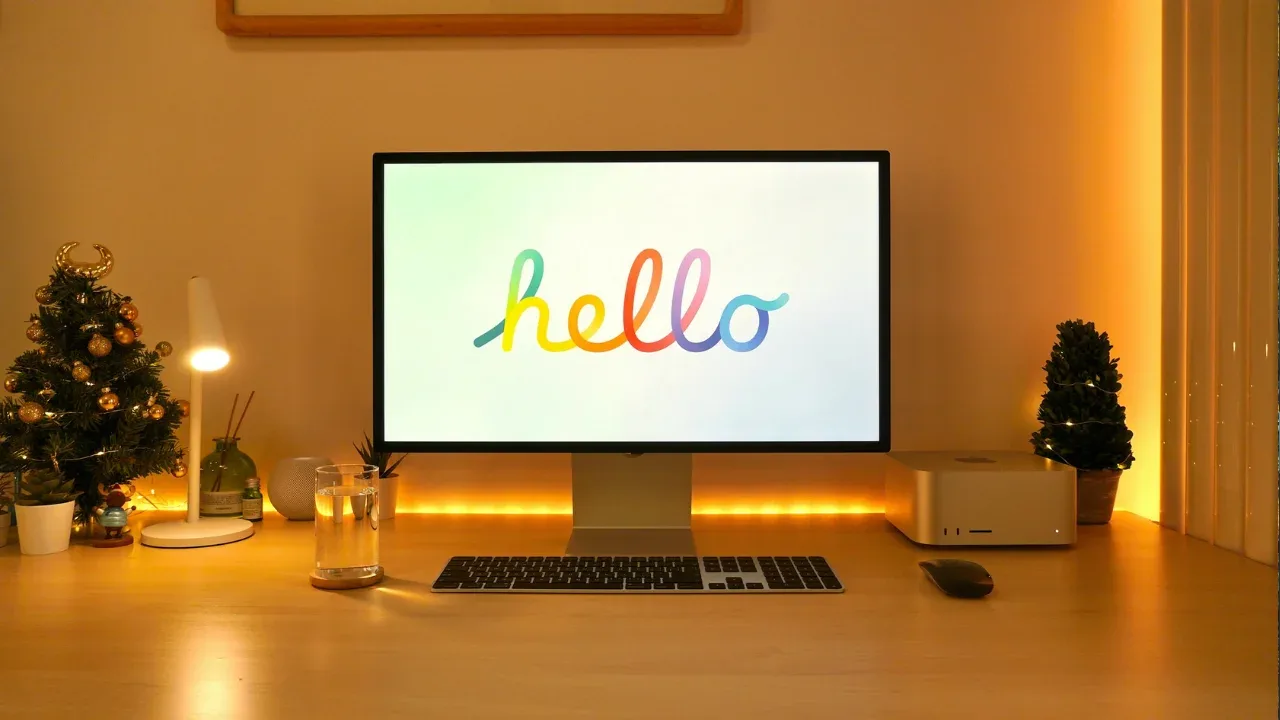
Removing HTML Tags from a String: The Ultimate Guide! 💪
So, you want to remove those pesky HTML tags from a string in Java, huh? You're in luck! In this blog post, we'll dive into this common issue and provide you with easy solutions to tackle it like a pro. 🚀
The Problem: Removing HTML Tags
Let's start by understanding the problem at hand. You have a Java string that contains HTML tags, and you need to get rid of them while preserving the content within the tags. Seems simple, right? Well, not quite.
The straightforward approach is to use a regular expression, like the one you mentioned:
String htmlString = "<p>Hello, <strong>world</strong>!</p>";
String cleanString = htmlString.replaceAll("\\<.*?\\>", "");
🔍 The regex explained:
\\<
: Matches the opening angle bracket<
..*?
: Matches any character (excluding line breaks) between the opening and closing angle brackets non-greedily.\\>
: Matches the closing angle bracket>
.
The Caveats: The Devil is in the Details 👿
While the above regex might do the trick for some cases, it has its limitations. Here are a couple of issues you might encounter:
Incorrect conversion of special characters: The regex won't properly convert characters like
&
, which should be converted to&
. This could result in incorrect rendering of your text.Non-HTML content removal: Using
.*?
in the regex pattern can inadvertently remove non-HTML content located between angle brackets. This means that tags like<a href="example.com">Click here!</a>
will becomeClick here!
.
The Solutions: Handling HTML Tags With Care ✨
To overcome these caveats, we need to take a more sophisticated approach. Luckily, the Java library jsoup
comes to the rescue! It provides a robust and reliable way to handle HTML parsing.
Solution 1: Using the jsoup Library
Start by including the
jsoup
library in your project. You can download the latest JAR file from their official website.Once you have the library added to your project, you can remove HTML tags from a string as follows:
import org.jsoup.Jsoup;
import org.jsoup.safety.Whitelist;
// ...
String htmlString = "<p>Hello, <strong>world</strong>!</p>";
String cleanString = Jsoup.clean(htmlString, Whitelist.none());
🔍 The explanation:
Jsoup.clean()
takes the HTML string as input and cleans it based on the providedWhitelist
. In this case, we useWhitelist.none()
to allow no tags, effectively removing all HTML tags.
With this approach, you can bid farewell to the issues we encountered earlier and enjoy a clean and tag-free string!
Solution 2: Regular Expressions with HtmlUtils (Spring Framework)
If you're using the Spring Framework in your Java project, you can leverage the HtmlUtils
class to deal with HTML tags.
Ensure that you have the Spring Framework included in your project. You can add the necessary dependencies to your
pom.xml
file if you're using Maven.Here's how you can remove HTML tags using
HtmlUtils
:
import org.springframework.web.util.HtmlUtils;
// ...
String htmlString = "<p>Hello, <strong>world</strong>!</p>";
String cleanString = HtmlUtils.htmlUnescape(HtmlUtils.htmlEscape(htmlString));
🔍 The explanation:
HtmlUtils.htmlEscape()
sanitizes the HTML string by escaping special characters and replacing the HTML tags with their corresponding entities.HtmlUtils.htmlUnescape()
reverses the process by converting the escaped entities back to their original form.
The Call-to-Action: Share Your Thoughts! 💬
There you have it! Two easy solutions to remove HTML tags from a string in Java, each with its own benefits. Now, it's your turn to give them a try. Which approach do you prefer? Have you encountered any other challenges when handling HTML tags? Let us know in the comments below! 👇
And remember, sharing is caring! If you found this post helpful, don't hesitate to share it with your fellow developers. Happy coding! 😄👩💻👨💻
*Disclaimer: Always use caution when modifying HTML content, and be aware of potential security vulnerabilities.
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
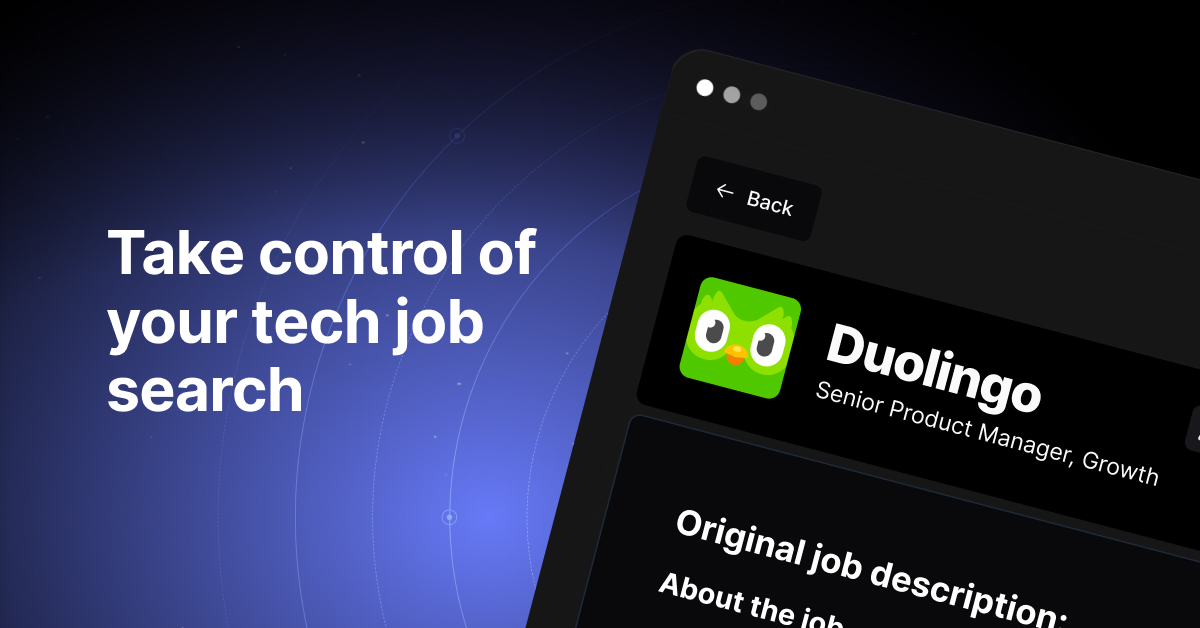