Regex for matching something if it is not preceded by something else
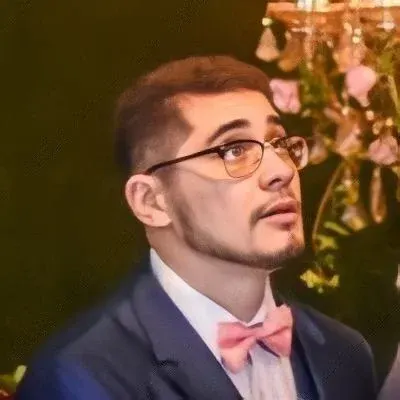
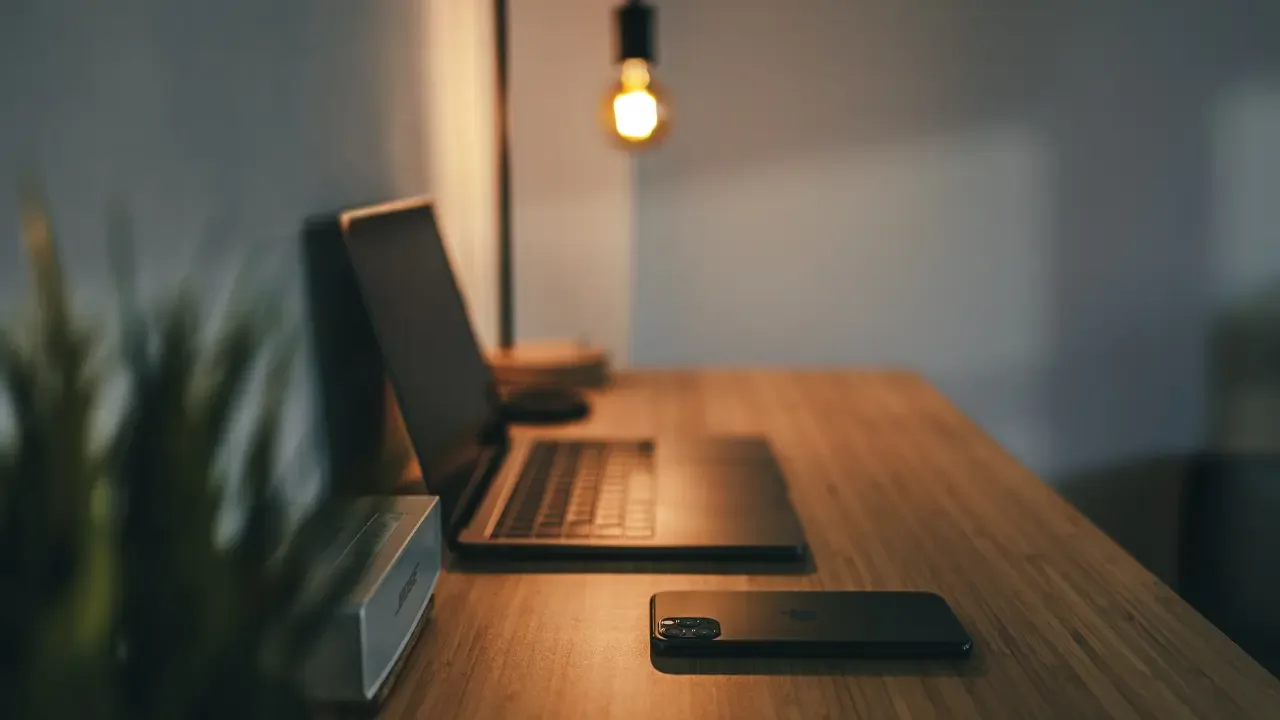
Regex for matching something if it is not preceded by something else
<!-- Introduction -->
Are you struggling to write a regex pattern that matches something only if it is not preceded by specific characters? 🤔 Don't worry, we've got you covered! In this blog post, we'll dive into the world of regular expressions and show you how to tackle this common issue. 💪
<!-- The Problem -->
The Problem: Matching if and only if not preceded by
Let's say you have a string in Java like this:
String s = "foobar barbar beachbar crowbar bar ";
You want to match instances of the word "bar", but only if it's not preceded by the word "foo". In this case, the desired output would be:
barbar
beachbar
crowbar
bar
This can be quite tricky to achieve using regular expressions alone. But fear not, we'll walk you through step by step!
<!-- Solution -->
The Solution: Negative Lookbehind
To accomplish this task, we can utilize a concept known as negative lookbehind in regular expressions. 🧐
The negative lookbehind assertion allows us to specify a pattern that must not be present before the desired match. In our case, we would use the following regex pattern:
(?<!foo)bar
Let's break this down:
(?<!foo)
is the negative lookbehind assertion. It states that the pattern should not be preceded by the characters "foo".bar
is the actual pattern we want to match.
By combining these two, we effectively match instances of "bar" that are not preceded by "foo".
Here's how you can apply this regex pattern in Java:
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class RegexDemo {
public static void main(String[] args) {
String s = "foobar barbar beachbar crowbar bar ";
Pattern pattern = Pattern.compile("(?<!foo)bar");
Matcher matcher = pattern.matcher(s);
while (matcher.find()) {
System.out.println(matcher.group());
}
}
}
This code will output the desired result:
barbar
beachbar
crowbar
bar
🎉 Congratulations! You've successfully solved the problem! 🎊
<!-- Conclusion -->
Conclusion
Regex can be a powerful tool, but it can also be quite daunting, especially when dealing with complex patterns. In this blog post, we focused on solving the specific problem of matching something only if it's not preceded by something else.
We explored the concept of negative lookbehind and provided a clear example in Java to help you grasp the solution. Remember to adjust the regex pattern according to your specific use case.
Now that you've mastered this regex challenge, go ahead and apply it to your own projects! Don't hesitate to experiment and explore other regex features to level up your pattern matching skills. And remember, practice makes perfect! 💪
If you have any questions or would like to share your experience, feel free to leave a comment below. We'd love to hear from you! 👇
Happy coding! 🚀✨
<!-- Call-to-Action -->
Ready for more regex challenges?
If you enjoyed solving the problem in this blog post, why not check out our other regex-related articles? From validating email addresses to extracting URLs, we cover a wide range of regex challenges that can help sharpen your skills.
Click here to browse through our collection of regex tutorials and take your regex game to the next level! 📚🔍🤓
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
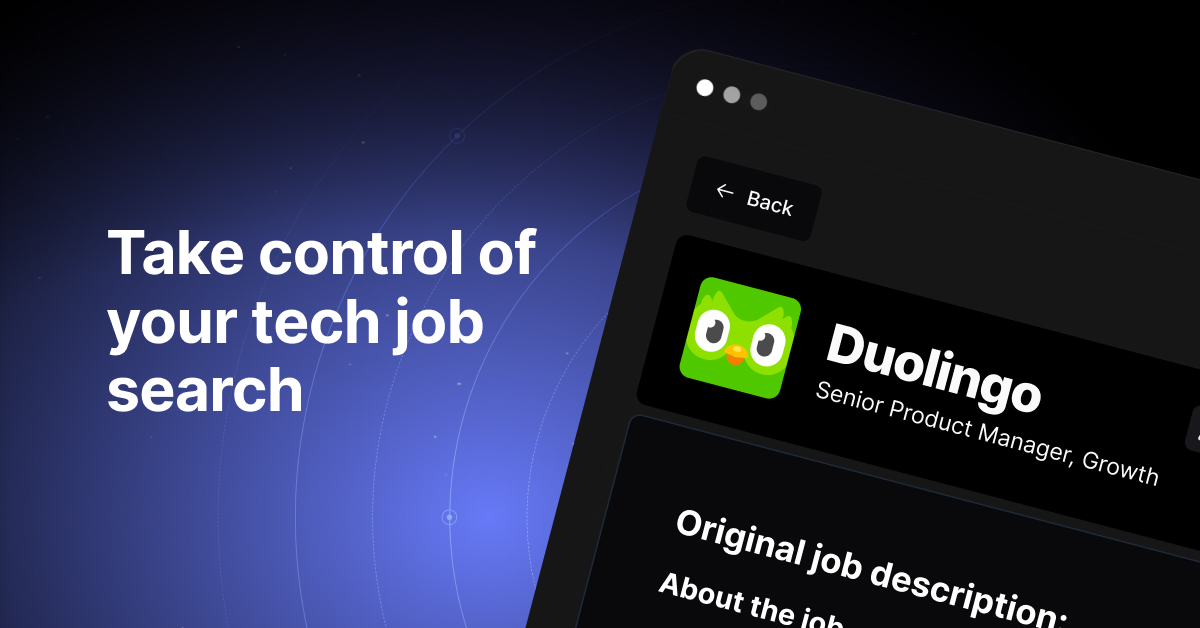