Redirect to an external URL from controller action in Spring MVC
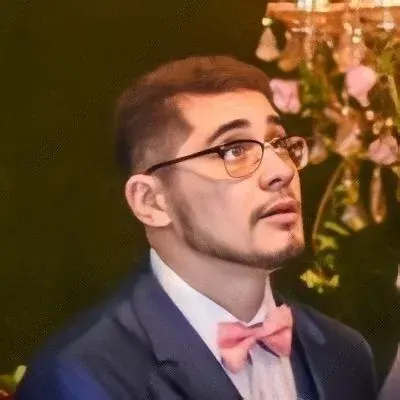
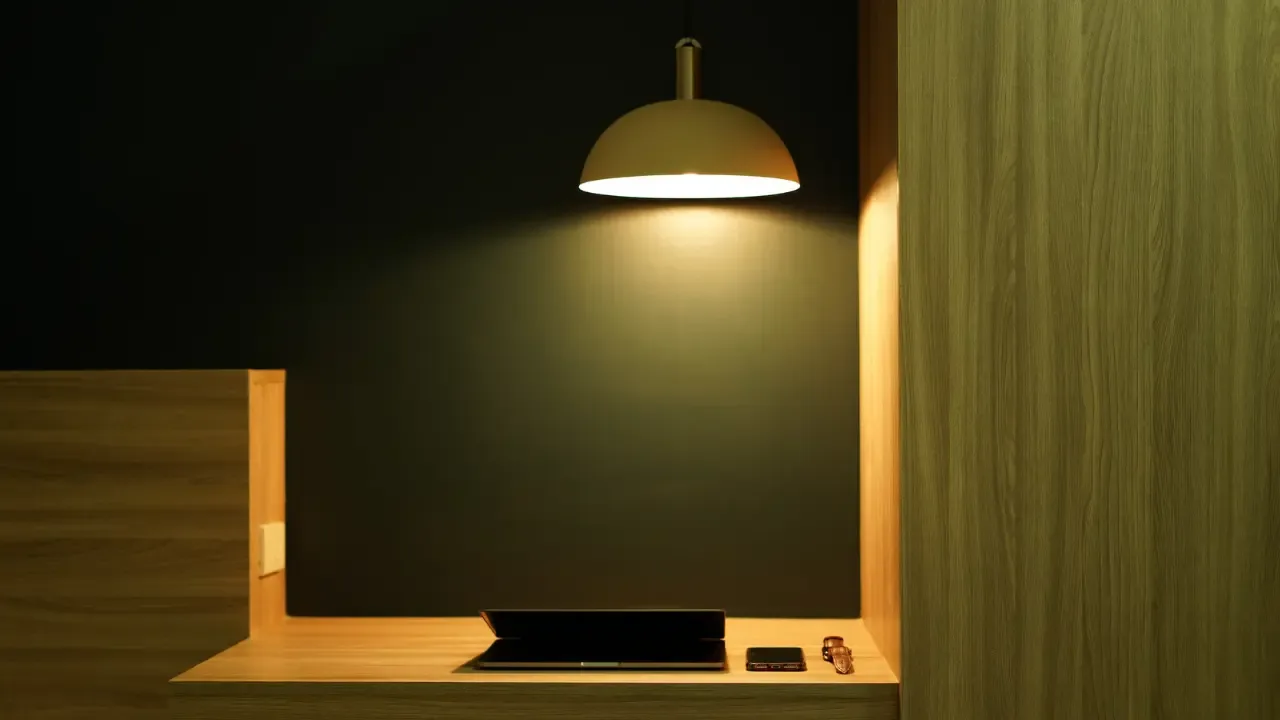
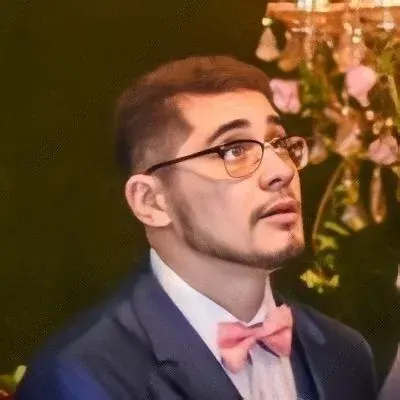
๐ Redirect to an External URL from Controller Action in Spring MVC
Have you ever tried redirecting to an external URL from a controller action in Spring MVC, only to find yourself encountering some issues? Don't worry, we've got your back! In this blog post, we'll address this common problem and provide you with easy solutions to ensure a smooth redirect. So, let's dive right in! ๐ป๐
The Problem
Let's take a look at the code snippet you provided:
@RequestMapping(method = RequestMethod.POST)
public String processForm(HttpServletRequest request, LoginForm loginForm,
BindingResult result, ModelMap model)
{
String redirectUrl = "yahoo.com";
return "redirect:" + redirectUrl;
}
As you rightly pointed out, this code redirects the user to a URL inside the project instead of an external URL. On the other hand, the following code does redirect properly as intended but requires the protocol (http:// or https://) to be specified:
@RequestMapping(method = RequestMethod.POST)
public String processForm(HttpServletRequest request, LoginForm loginForm,
BindingResult result, ModelMap model)
{
String redirectUrl = "http://www.yahoo.com";
return "redirect:" + redirectUrl;
}
What you want is a solution that redirects to the specified URL, regardless of whether it has a valid protocol or not. Additionally, you do not want to redirect to a view. So, how can you achieve that? ๐ค
Solution 1: Protocol Handling
To ensure that the redirect always works, whether the protocol is specified or not, you can modify the processForm
method as follows:
@RequestMapping(method = RequestMethod.POST)
public String processForm(HttpServletRequest request, LoginForm loginForm,
BindingResult result, ModelMap model)
{
String redirectUrl = "http://" + "yahoo.com";
return "redirect:" + redirectUrl;
}
By prefixing the URL with "http://"
, you guarantee that the redirect will always have a valid protocol. However, you may be wondering if there's a more elegant solution. Let's find out! ๐กโจ
Solution 2: URL Handling with URI Components
Another approach you can take is to leverage the UriComponentsBuilder
class provided by Spring, which simplifies URL construction and manipulation. Here's what the modified code would look like:
@RequestMapping(method = RequestMethod.POST)
public String processForm(HttpServletRequest request, LoginForm loginForm,
BindingResult result, ModelMap model)
{
UriComponents redirectUrl = UriComponentsBuilder.newInstance()
.scheme("https") // Specify your desired protocol here
.host("yahoo.com") // Specify the host/domain here
.build();
return "redirect:" + redirectUrl.toUriString();
}
This solution not only allows you to specify the protocol but also enables you to customize other parts of the URL, such as the host/domain. Pretty cool, right? ๐
The Compelling Call-to-Action
We hope this guide has provided you with the solutions you were looking for! Now it's your turn to put this knowledge into practice and redirect to external URLs effortlessly. Share this blog post with your developer friends who might face similar challenges, and let's help each other grow!
If you have any questions or other Spring MVC-related topics you want us to cover, let us know in the comments below. We always love hearing from you! ๐๐ฌ
Remember to subscribe to our newsletter for more awesome tech tips and stay updated with the latest trends in the tech world. Until next time, happy coding! ๐ฉโ๐ป๐จโ๐ป