Reading a plain text file in Java
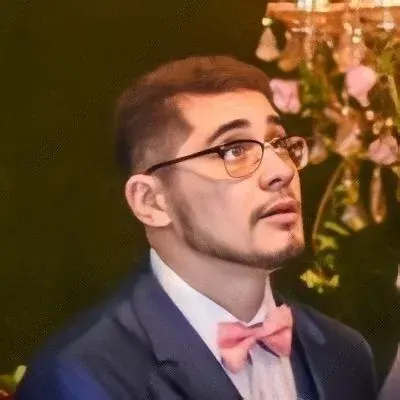
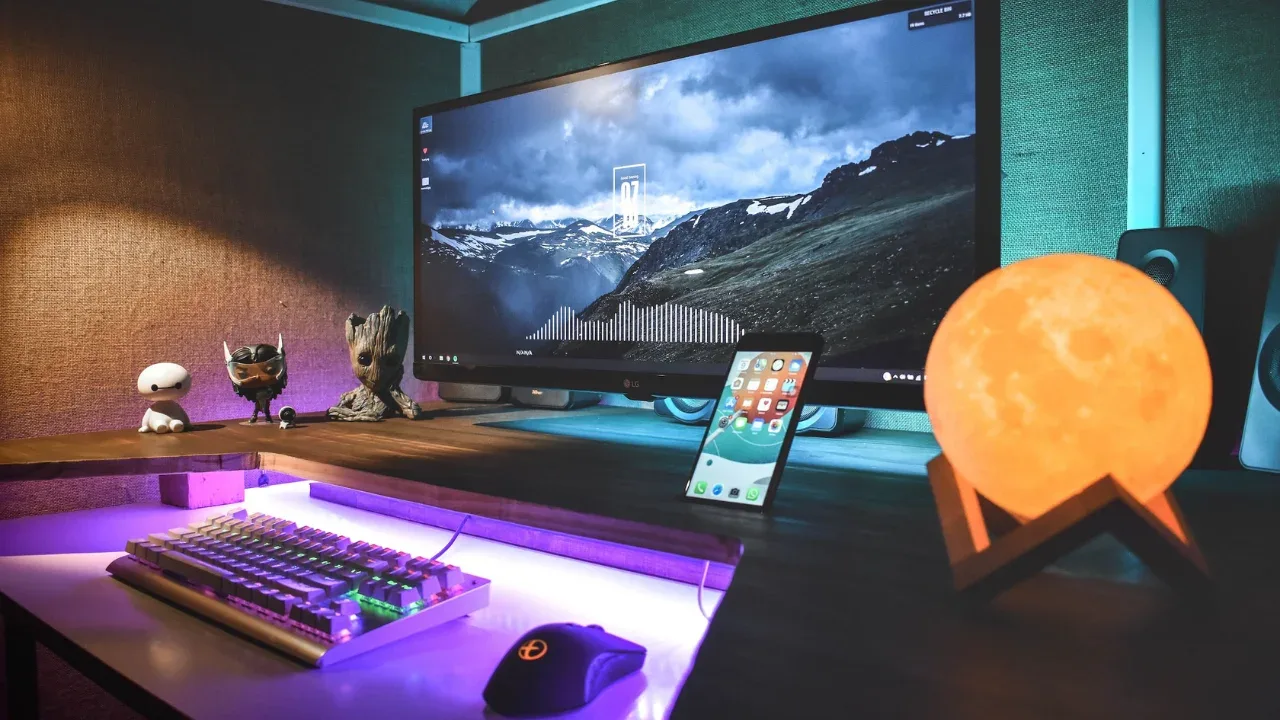
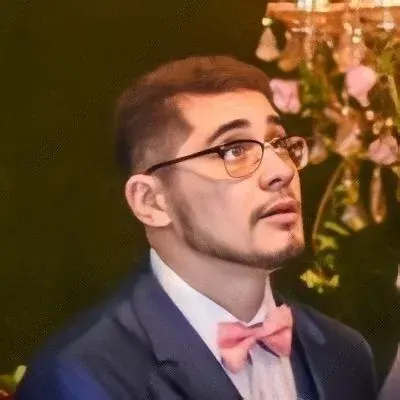
πTitle: "Reading a Plain Text File in Java: Demystifying the Different Approaches"
Introduction: π Hey Java enthusiasts! So, you've stumbled upon a task that many developers face - reading ASCII data from a plain text file in Java. π Fear not! In this guide, we'll explore the various ways to tackle this challenge, highlighting their differences and providing easy solutions. Let's dive right in! π»
BufferedReader and FileReader Approach:
Sometimes, the simplest solution is the best. π§ The BufferedReader and FileReader classes in Java can help us accomplish our goal effortlessly.
try (BufferedReader br = new BufferedReader(new FileReader("path/to/your/file.txt"))) {
String line;
while ((line = br.readLine()) != null) {
// Process each line of the file
}
} catch (IOException e) {
// Handle the exception
}
π With this approach, we read the file line by line using the readLine()
method from BufferedReader. It's efficient and memory-friendly!
Files.lines Approach:
Java 8 introduced the powerful Files
class, which provides several utility methods to manipulate files. One such method, lines()
, simplifies file reading.
try (Stream<String> lines = Files.lines(Paths.get("path/to/your/file.txt"))) {
lines.forEach(line -> {
// Process each line of the file
});
} catch (IOException e) {
// Handle the exception
}
π By leveraging the lines()
method, we can obtain a Stream of lines from the file, which allows for a concise and elegant approach. Plus, it automatically handles the closure of the file.
Scanner Approach:
For more advanced file reading needs, the Scanner class can come to the rescue. It not only allows us to read plain text files but also supports parsing different data types.
try (Scanner scanner = new Scanner(new File("path/to/your/file.txt"))) {
while (scanner.hasNextLine()) {
String line = scanner.nextLine();
// Process each line of the file
}
} catch (FileNotFoundException e) {
// Handle the exception
}
π Utilizing the Scanner class, we can read each line using nextLine()
and process them accordingly. It's flexible and handy for specific use cases!
Apache Commons IO Approach:
If you're already using Apache Commons IO library in your project, it provides a convenient alternative for file reading.
try {
List<String> lines = FileUtils.readLines(new File("path/to/your/file.txt"), StandardCharsets.UTF_8);
for (String line : lines) {
// Process each line of the file
}
} catch (IOException e) {
// Handle the exception
}
π Apache Commons IO can be a lifesaver, abstracting away complex file operations and providing a reliable way to read plain text files.
π£ Call-to-Action: There you have it! A comprehensive overview of how to read ASCII data from a plain text file in Java. π‘ Don't be shy, try out these different approaches and pick the one that suits your needs! If you have any questions or want to share your experiences, let's connect in the comments section below. Happy coding! ππ»