Reading a List from properties file and load with Spring annotation @Value
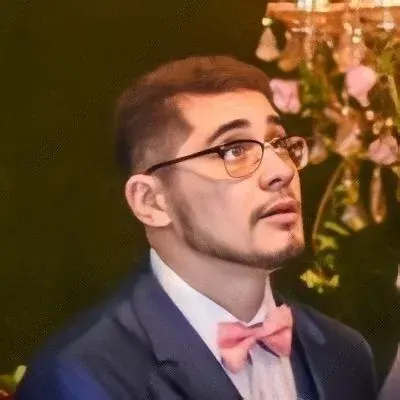
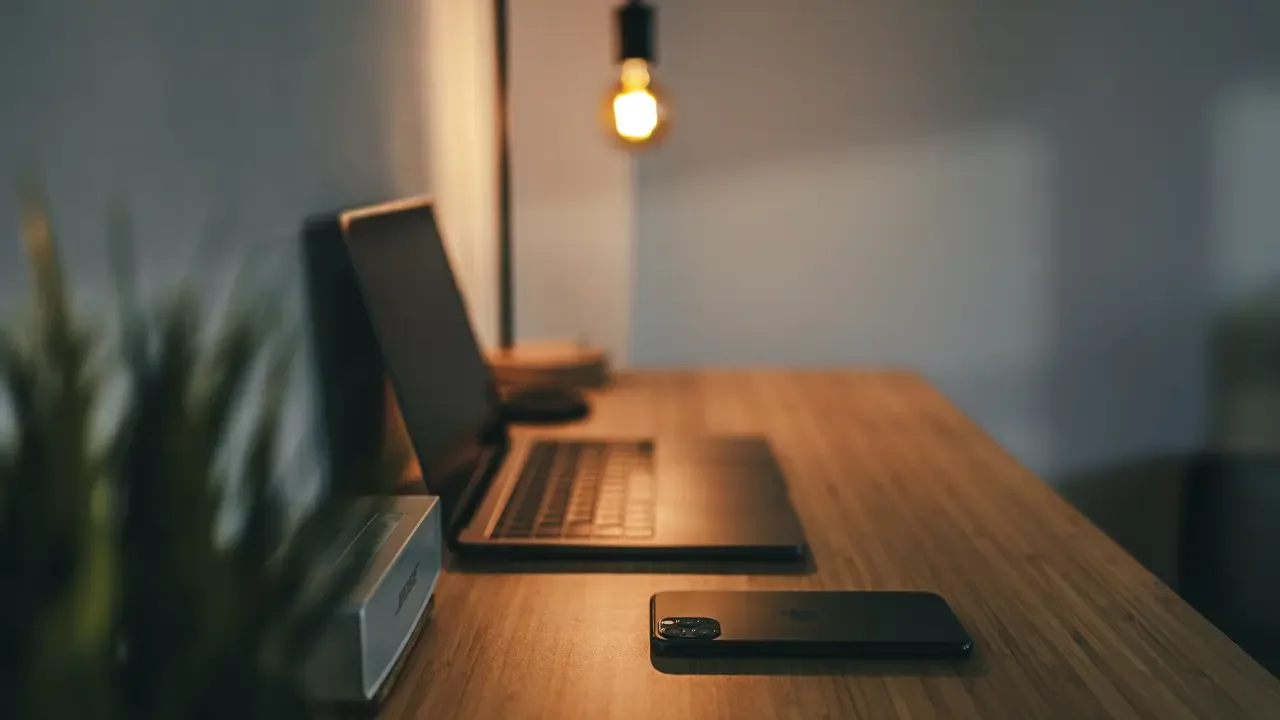
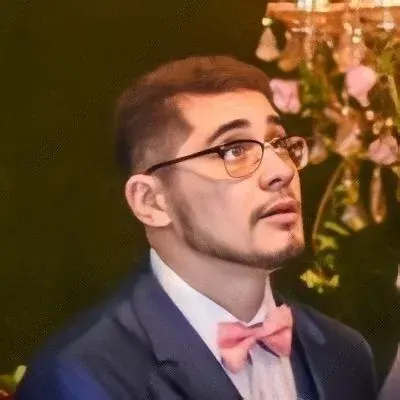
Reading a List from Properties File and Loading with Spring Annotation @Value
So you want to have a list of values in a .properties
file and load it directly into your class using Spring annotation @Value
. You're wondering if there's a way to do this without any custom code. Good news! There is a simple solution. Let's dive into it.
The Scenario
Say you have a .properties
file (application.properties
for example) with the following entry:
my.list.of.strings=ABC,CDE,EFG
And you want to load these values into a List<String>
in your Spring class using the @Value
annotation:
@Value("${my.list.of.strings}")
private List<String> myList;
The Solution: Using Spring Expression Language (SpEL)
To achieve this, we can leverage the power of Spring Expression Language (SpEL) in combination with the @Value
annotation.
To load a list of values from a property and convert them into a List<String>
, you can use the following syntax:
@Value("#{'${my.list.of.strings}'.split(',')}")
private List<String> myList;
Let's break it down:
${my.list.of.strings}
: This is the property placeholder expression. It retrieves the value from the.properties
file.#{...}
: This denotes the usage of SpEL.'${my.list.of.strings}'.split(',')
: This expression retrieves the value as a single string and then splits it using the comma delimiter.List<String>
: This specifies the type of the list to be populated.
That's it! With this simple modification, Spring will automatically read the property value, split it into individual elements, and populate your List<String>
for you.
Other Considerations
Escape Special Characters
In case your property values contain special characters that need escaping (e.g., if a value itself contains a comma), you can use the backslash \
before the special character to escape it, like this:
my.list.of.strings=ABC,CD\,E,EFG
Empty List Handling
If the property value is not present or empty, Spring will automatically assign an empty list to myList
. No additional code is needed for handling this scenario.
Alternative Approach: Using XML Configuration
As mentioned in the question, an alternative approach is to define the list directly in the Spring configuration XML file. Here's how it would look:
<bean name="list">
<list>
<value>ABC</value>
<value>CDE</value>
<value>EFG</value>
</list>
</bean>
By defining the list as a bean, you can then reference it using the @Resource
or @Autowired
annotation.
Conclusion
Reading a list from a .properties
file and loading it with the Spring annotation @Value
is easily achievable using Spring Expression Language (SpEL). By following the simple syntax @Value("#{'${my.list.of.strings}'.split(',')}")
, you can populate your List<String>
without any custom code.
Remember to escape special characters if necessary and handle the case of an empty property value. And don't forget, if XML configuration is more to your liking, you can always define the list directly in your Spring XML configuration file.
Now go ahead and give it a try! Share your thoughts, experiences, and any other creative solutions you come up with in the comments below. Happy coding! 😎🚀📝