Read file from resources folder in Spring Boot
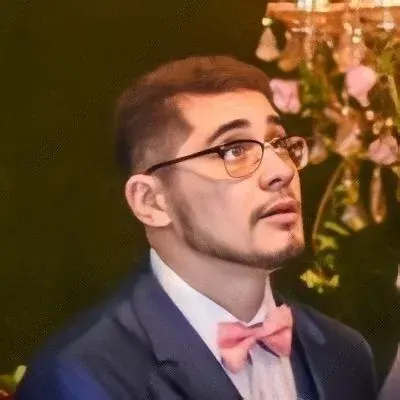
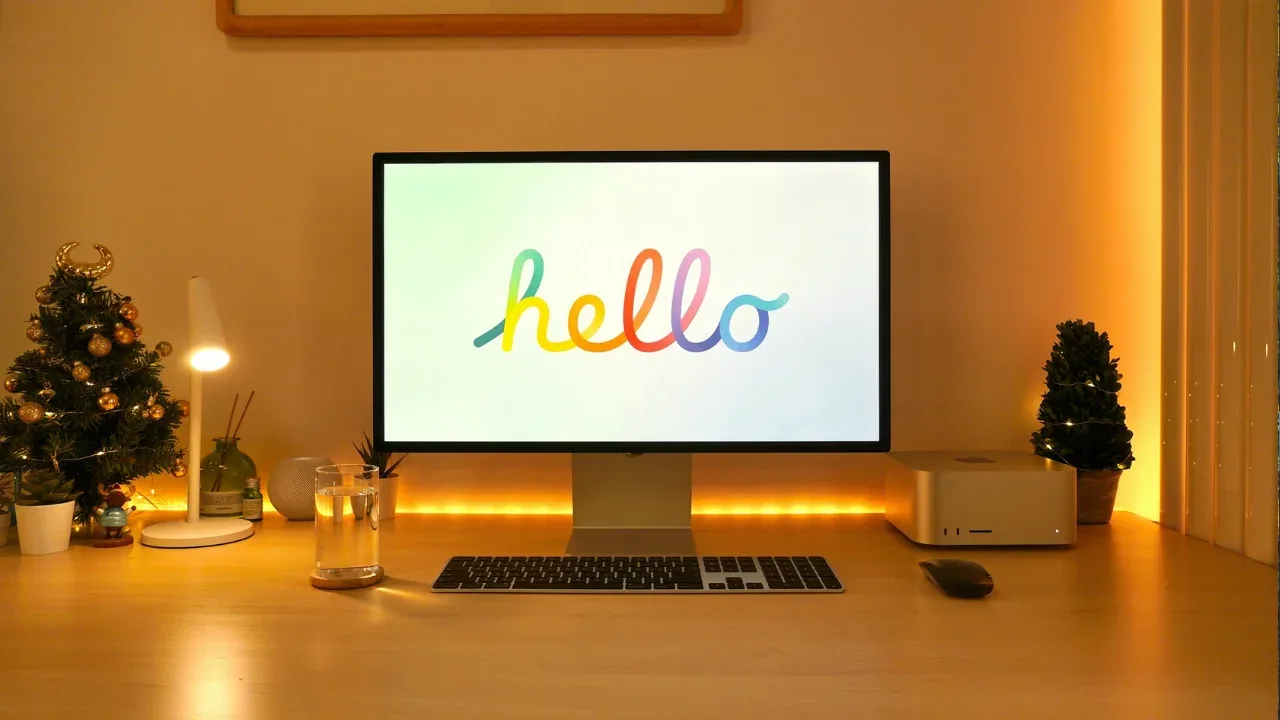
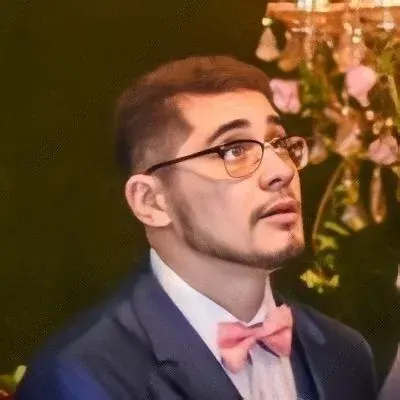
š»šš Reading Files from the Resources Folder in Spring Boot: A Guide š
Are you using Spring Boot and struggling to read a file from the resources folder? š« Don't worry, you're not alone! This common issue can be tricky to resolve, but fear not! In this guide, we'll explore a simple solution to help you navigate this challenge. Let's dive in! š
Understanding the Problem š¤
The error message mentions a "No such file or directory" issue, indicating that the file path you're providing might be incorrect. Let's explore a better approach to reading files from the resources folder in Spring Boot. š”
Solution: ClassLoader.getResource() š
To read a file from the resources folder, we can use the ClassLoader.getResource()
method. This method returns a URL representing the resource location. Here's how you can modify your code to fix the issue:
ClassLoader classLoader = getClass().getClassLoader();
URL resource = classLoader.getResource("jsonschema.json");
File file = new File(resource.toURI());
JsonNode mySchema = JsonLoader.fromFile(file);
By using the getClassLoader().getResource()
method, you can obtain the URL for the desired file, even if it's inside the resources folder. The toURI()
method is used to convert the URL to a File object, allowing you to access its contents successfully. š
Addressing File Not Found Errors ā
Sometimes, even after making the changes mentioned above, you might still encounter a "File Not Found" error. This issue typically arises if you're running your application from an IDE, as the default working directory might be different. To resolve this, you can try the following:
Clean and rebuild your project to ensure that the resources are being packaged correctly.
Verify the location of your resources folder in your project structure. It should be located inside the
src/main
directory.Try running your Spring Boot application from the command line instead of your IDE. This will ensure that the relative paths are resolved correctly.
Take It a Step Further! šŖ
Now that you've successfully resolved the issue of reading files from the resources folder, why not take it a step further? Here's a call-to-action to help you engage and explore more:
Share this post with fellow developers who might be facing the same challenge. š¬
Experiment with different file types (e.g., XML, properties) to see how you can apply this solution to various scenarios. š”
Leave a comment below, sharing your experience and any other tricks you've discovered along the way. Let's learn from each other! š
In Conclusion š
Reading files from the resources folder in Spring Boot can be a bit tricky, but with the right approach, it's definitely achievable. By using ClassLoader.getResource()
and ensuring your resource files are correctly packaged, you'll be able to access them successfully. Don't let this challenge hold you back in your development journey. Keep on coding and exploring! šš»
Happy coding! šāØ
References:
Articles you might like
š Exploring the Power of React Hooks: A Comprehensive Guide š Top 10 JavaScript Tricks Every Developer Should Know