Quickest way to convert XML to JSON in Java
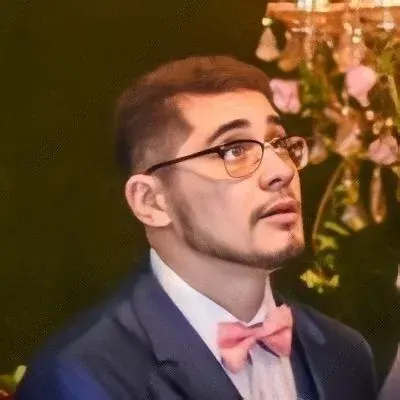
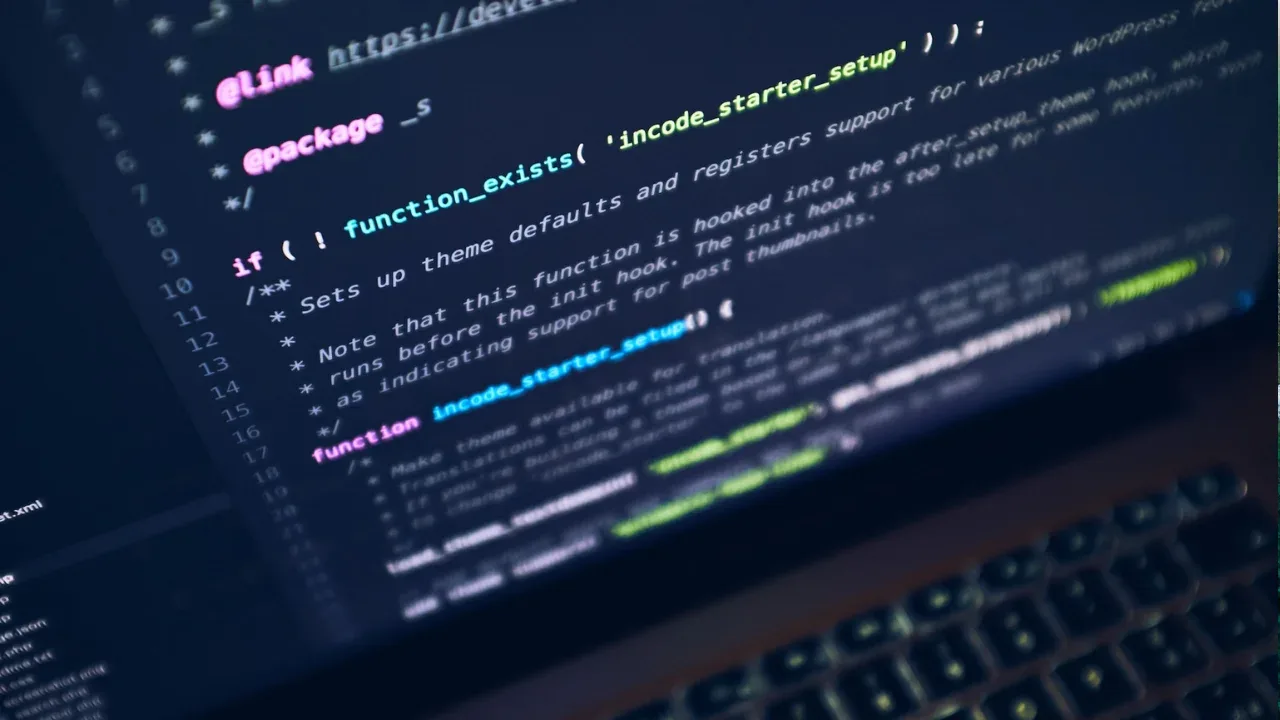
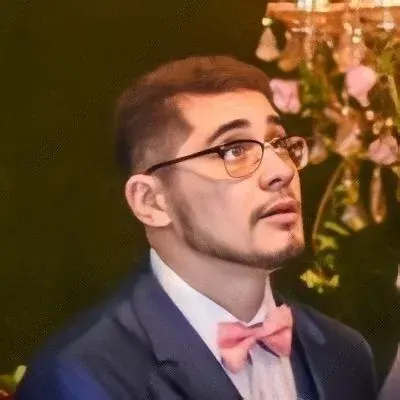
The Quickest Way to Convert XML to JSON in Java 🚀
Have you ever found yourself in a situation where you needed to convert XML to JSON in Java? Don't worry, you're not alone! Many developers face this challenge, but fear not, as we have got you covered. In this blog post, we'll walk you through the quickest and easiest way to convert XML to JSON in Java, addressing common issues and providing simple solutions.
The Challenge: Converting XML to JSON
Converting XML to JSON can be a daunting task, especially if you're not familiar with the intricacies of both formats. But fear not, there are libraries available that can simplify this process for you. One such library is the Jackson library, which provides powerful and efficient XML and JSON processing capabilities.
Common Issues
Before we dive into the solution, let's take a look at some common issues developers face when converting XML to JSON:
Complex XML Structures: XML can have complex nested structures, with attributes, namespaces, and multiple levels of elements. Converting such XML structures to JSON requires careful handling.
Mismatched Data Types: XML and JSON have different data type representations. For example, XML supports data types like date, time, and decimal, whereas JSON represents everything as strings. Mapping these types correctly during the conversion is crucial.
The Solution: Using the Jackson Library
Now that we understand the challenges, let's explore how to use the Jackson library to convert XML to JSON. Follow these steps and you'll be on your way to successful conversion:
Add the Jackson library dependencies: To get started, you need to add the Jackson libraries to your project. Simply include the following Maven dependencies:
<dependency> <groupId>com.fasterxml.jackson.dataformat</groupId> <artifactId>jackson-dataformat-xml</artifactId> <version>2.12.2</version> </dependency> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> <version>2.12.2</version> </dependency>
Don't forget to update the version numbers if needed.
Perform the conversion: With the Jackson library in place, you're ready to convert XML to JSON. Here's an example code snippet to get you started:
import com.fasterxml.jackson.databind.ObjectMapper; import com.fasterxml.jackson.dataformat.xml.XmlMapper; public class XmlToJsonConverter { public static String convert(String xml) throws Exception { XmlMapper xmlMapper = new XmlMapper(); ObjectMapper objectMapper = new ObjectMapper(); Object jsonObject = xmlMapper.readValue(xml, Object.class); return objectMapper.writeValueAsString(jsonObject); } }
In this example, we create an instance of
XmlMapper
to read the XML input, andObjectMapper
to convert the XML to JSON.Test your conversion: It's always a good idea to test your code. Here's a simple test method you can use:
public class Main { public static void main(String[] args) throws Exception { String xml = "<root><name>John Doe</name><age>30</age></root>"; String json = XmlToJsonConverter.convert(xml); System.out.println(json); } }
Run this code, and you should see the XML converted to JSON printed in the console.
Give it a Try!
Now that you know how to quickly and easily convert XML to JSON in Java using the Jackson library, give it a try yourself! Experiment with different XML structures, handle complex scenarios, and see how the library handles them effortlessly.
We hope this guide helps you overcome the challenges of converting XML to JSON in Java. If you have any questions or suggestions, feel free to leave a comment below. Happy coding! 👨💻🚀
💌 Don't forget to share this post with your fellow developers who might find it useful! Sharing is caring! 🤝