Programmatically shut down Spring Boot application
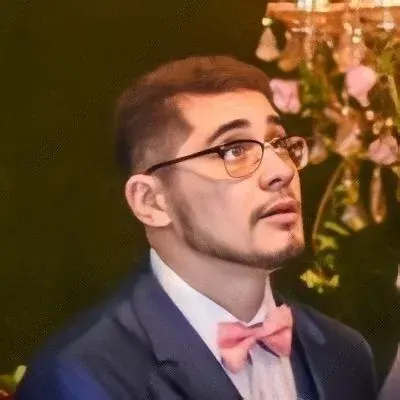
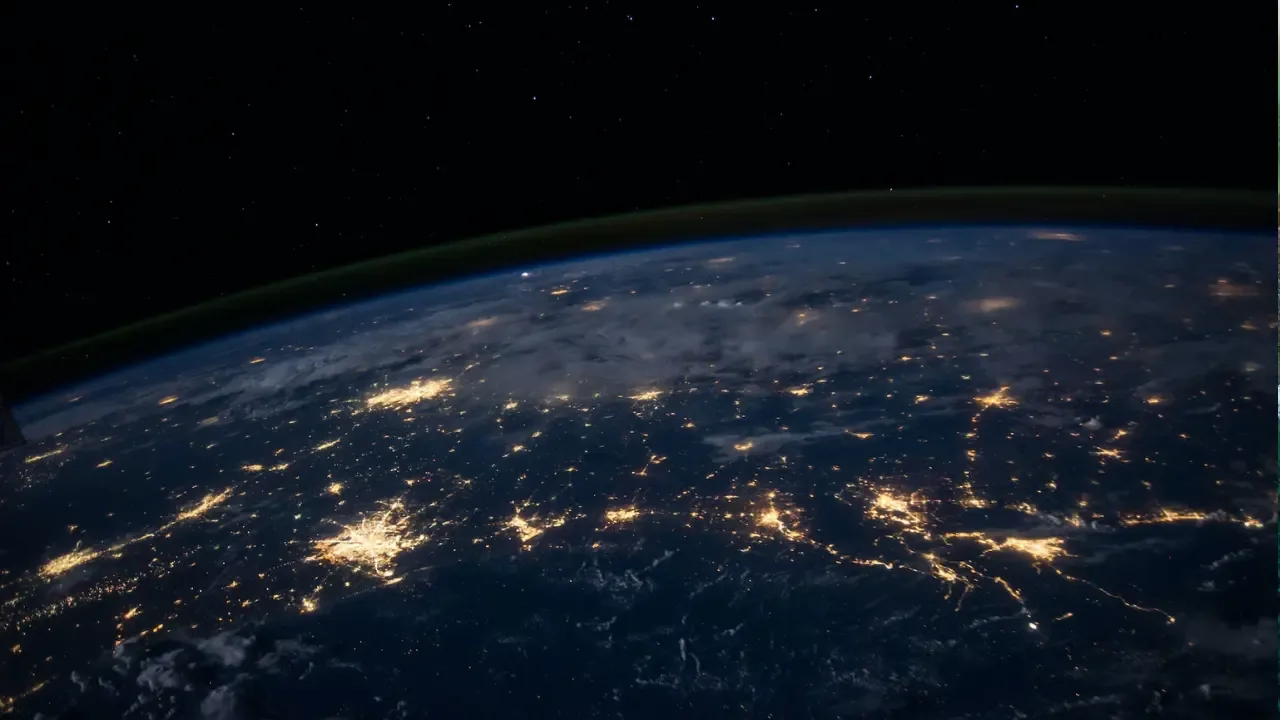
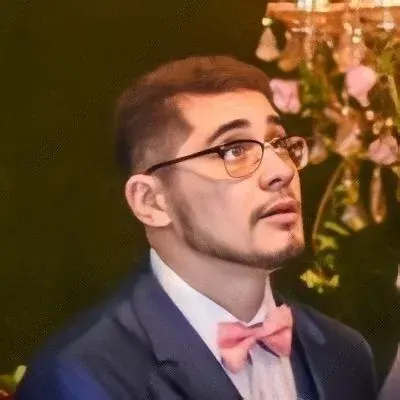
🛑 Shutting Down a Spring Boot Application Programmatically 🛑
So, you've built an awesome Spring Boot application and now you want to gracefully shut it down programmatically, without terminating the VM? Well, you've come to the right place! In this blog post, we'll explore the answer to this common question and provide you with some easy solutions. Let's dive right in! 💪
The Opposite of new SpringApplication(Main.class).run(args);
To understand how to programmatically shut down a Spring Boot application, let's first discuss the opposite. When you start your Spring Boot application using the SpringApplication.run()
method, it spins up a web server and begins listening for incoming requests.
But how can we stop this gracefully without forcefully terminating the VM? 🤔
Solution 1: Using the SpringApplication.exit()
Method
One simple and straightforward way to shut down a Spring Boot application programmatically is by using the SpringApplication.exit()
method. This method allows you to gracefully stop the application by triggering the shutdown process.
Here's how you can do it:
SpringApplication application = new SpringApplication(Main.class);
ConfigurableApplicationContext context = application.run(args);
// ... Your awesome application logic ...
// Now, let's gracefully shut down the application
int exitCode = SpringApplication.exit(context);
System.exit(exitCode);
In this approach, we first start the application using SpringApplication.run()
, perform any necessary tasks, and then trigger the shutdown process by calling SpringApplication.exit()
with the application context. Finally, we use System.exit()
to terminate the VM gracefully.
Solution 2: Using a Custom Endpoint
Another way to shut down a Spring Boot application programmatically is by exposing a custom management endpoint. This approach allows you to trigger the shutdown process by sending an HTTP request to a specific endpoint.
Here's how you can implement it:
Add the Spring Boot Actuator dependency to your
pom.xml
file:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
Create a custom controller that listens for shutdown requests:
@RestController
public class ShutdownController {
@Autowired
private ApplicationContext context;
@PostMapping("/shutdown")
public void shutdown() {
SpringApplication.exit(context, () -> 0);
}
}
Secure the shutdown endpoint (optional):
@Configuration
public class ActuatorSecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/shutdown").hasRole("ADMIN")
.anyRequest().permitAll()
.and()
.csrf().disable();
}
}
In this solution, we create a custom controller with a shutdown()
method that calls SpringApplication.exit()
with the application context. You can secure the endpoint by using Spring Security and restrict access to authorized users only.
Conclusion
And that's it! You now have two easy solutions to programmatically shut down your Spring Boot application without terminating the VM. Feel free to choose the approach that best fits your requirements.
Whether you're gracefully shutting down your application to perform a clean restart or adding a convenient API endpoint for remote shutdown, these methods will surely come in handy. So go ahead, implement them in your code, and enjoy the ease of programmatically shutting down your Spring Boot application!
Do you have any other Spring Boot questions or interesting use cases? Share them in the comments below and let's continue the conversation! 😄👇