Pretty-Print JSON in Java
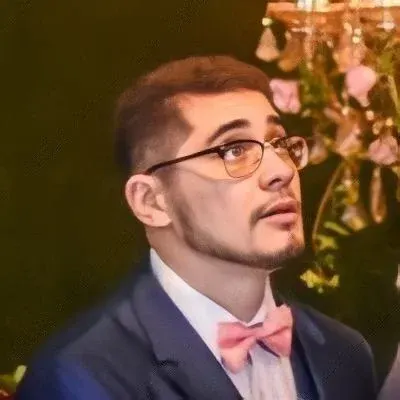
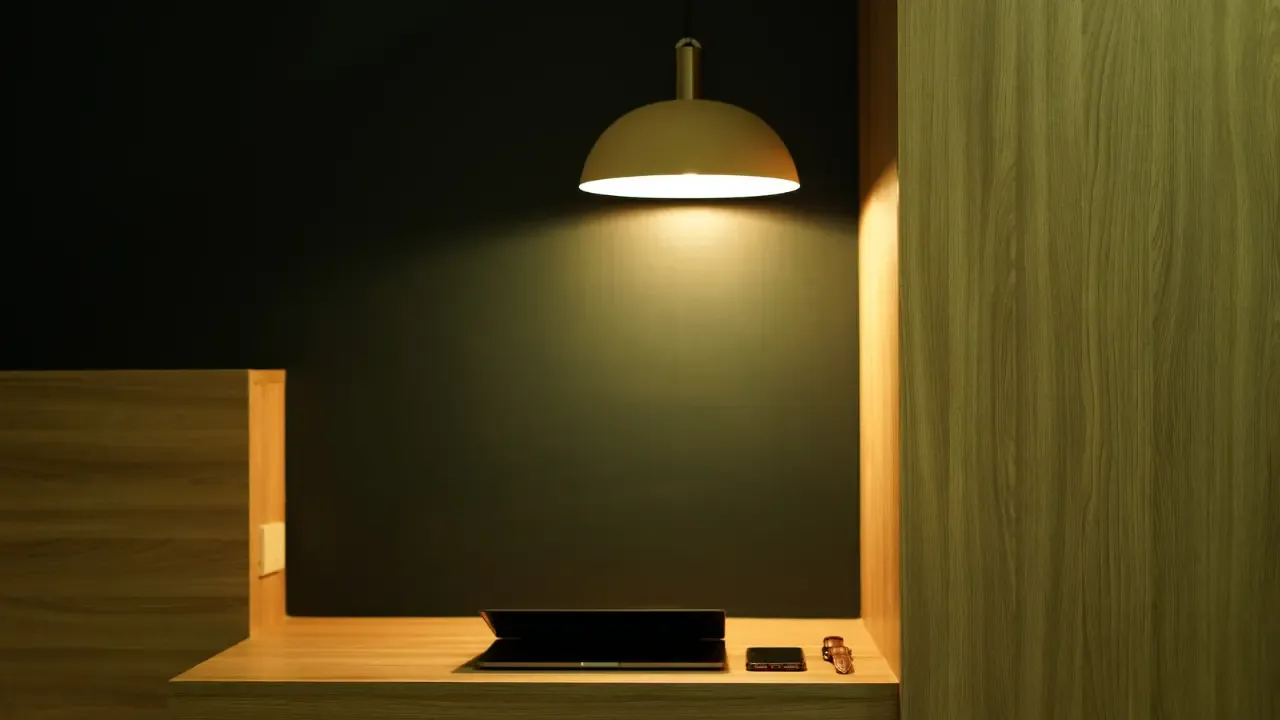
Pretty-Print JSON in Java like a Pro! π
Do you ever find yourself in a situation where you need to make your JSON data more human-readable? Don't worry, you're not alone! π In this blog post, we'll explore how to pretty-print JSON in Java, even if your current library doesn't provide this functionality. Let's dive right in! π
The Challenge: Making JSON Data Pretty
The original question mentioned using the json-simple
library, which unfortunately doesn't have built-in support for pretty-printing JSON. But fret not, because we have some neat tricks up our sleeves! πͺ
Solution 1: The Gson Library to the Rescue π¦ΈββοΈ
One popular solution to pretty-print JSON is by using the Gson library. Gson is a fantastic library for working with JSON in Java, and luckily, it has a built-in feature for making JSON output readable.
Here's a simple code snippet to get you started:
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
public class JsonPrinter {
public static void main(String[] args) {
String jsonString = "{\"name\":\"John\",\"age\":30,\"city\":\"New York\"}";
Gson gson = new GsonBuilder().setPrettyPrinting().create();
String prettyJson = gson.toJson(jsonString);
System.out.println(prettyJson);
}
}
In the above example, we create a Gson
object with the setPrettyPrinting()
option enabled. Then we simply pass our JSON string to the toJson()
method, which handles the heavy lifting for us. Finally, we print the pretty-printed JSON to the console.
Solution 2: Jackson Library to the Rescue Too π¦ΈββοΈ
Another powerful library for working with JSON in Java is Jackson. Just like Gson, Jackson also provides a way to pretty-print JSON. Let's take a look at how we can achieve this:
import com.fasterxml.jackson.databind.ObjectMapper;
public class JsonPrinter {
public static void main(String[] args) throws Exception {
String jsonString = "{\"name\":\"John\",\"age\":30,\"city\":\"New York\"}";
ObjectMapper objectMapper = new ObjectMapper();
String prettyJson = objectMapper.writerWithDefaultPrettyPrinter().writeValueAsString(jsonString);
System.out.println(prettyJson);
}
}
In this solution, we use the ObjectMapper
class from the Jackson library. We create an instance of ObjectMapper
, and then use the writerWithDefaultPrettyPrinter()
method to enable pretty-printing. Finally, we convert our JSON string to a pretty-printed format using the writeValueAsString()
method.
Conclusion: Make Your JSON Shine! β¨
No more tearing your hair out over making JSON data more readable! With libraries like Gson and Jackson, you have the power to pretty-print your JSON in a snap.
Feel free to experiment with these libraries and find the one that suits your needs best. You can explore their documentation to discover more advanced options and features.
So, go ahead and make your JSON shine like a star! β¨
Got more questions or other cool solutions? We'd love to hear from you in the comments below! Let's unleash the power of your JSON data together! πͺπ¬
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
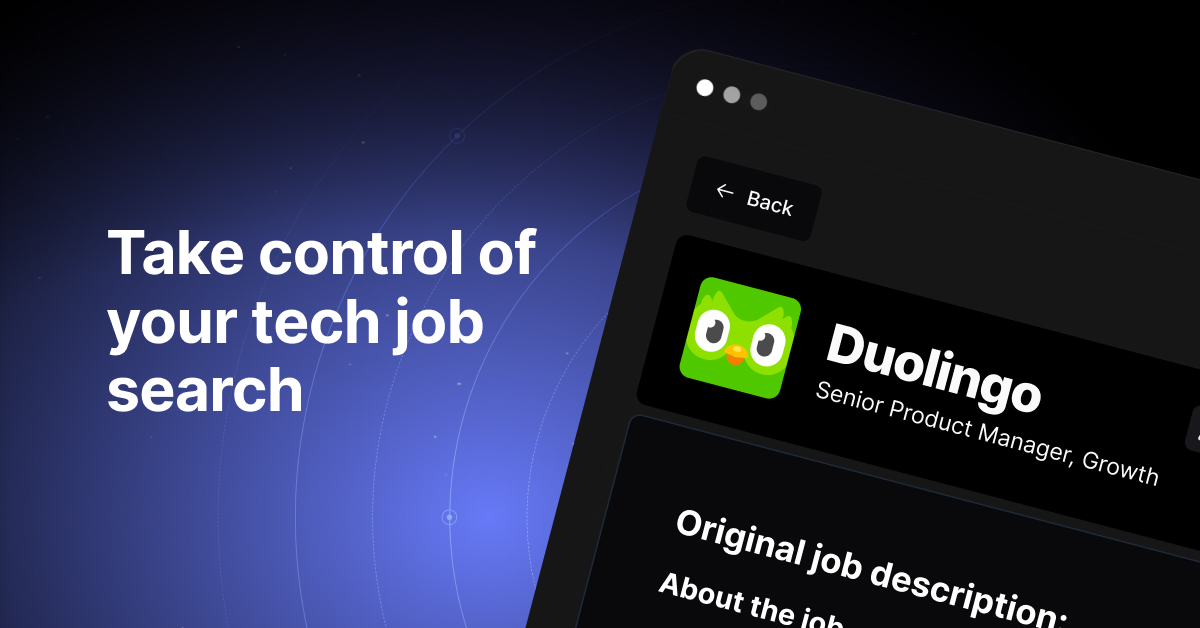