Populating Spring @Value during Unit Test
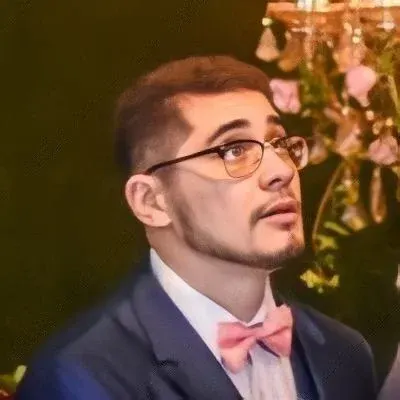
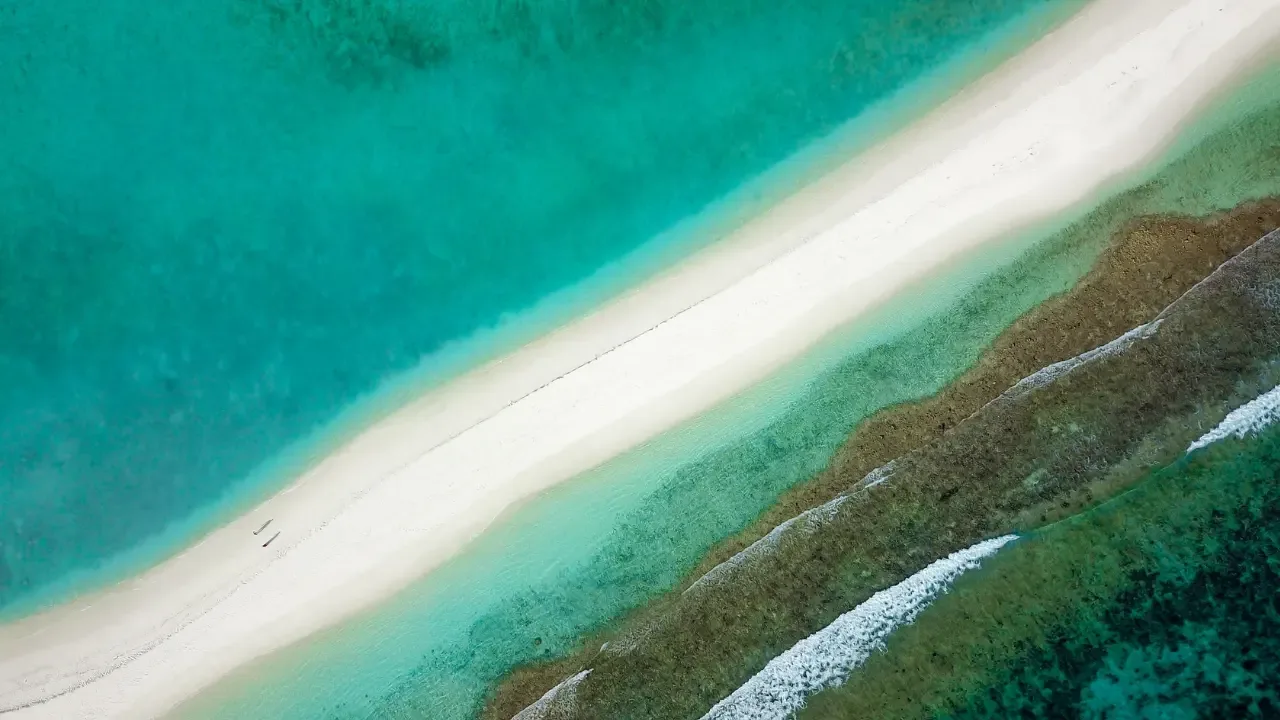
Populating Spring @Value during Unit Test: A Simple Guide
š Problem: You want to write unit tests for a bean in your Spring program that uses the @Value
annotation to initialize its properties. However, you'd prefer to avoid using a properties file in your unit tests to prevent any changes in the file from affecting the test cases.
šÆ Solution: You can utilize Java code within your test class to initialize the bean and populate the Spring @Value
property without relying on a properties file.
āļø Here's how you can do it:
Create a Test Configuration
In your test class, create a nested static class and annotate it with @Configuration
. This nested class will serve as the configuration for your unit tests.
@Configuration
public static class TestConfig {
@Bean
public YourBean yourBean() {
YourBean bean = new YourBean();
bean.setThisProperty("your-test-value");
return bean;
}
}
Set up the Test Environment
In your test class, annotate it with @RunWith(SpringRunner.class)
and specify the test configuration class using @ContextConfiguration
. Also, autowire the bean you want to test.
@RunWith(SpringRunner.class)
@ContextConfiguration(classes = {TestConfig.class})
public class YourBeanTest {
@Autowired
private YourBean yourBean;
// Your test methods...
}
Write Your Test Cases
Now, you can write your test cases and call the methods inside your yourBean
instance.
@Test
public void yourTestMethod() {
// Example test case
String result = yourBean.yourMethodToTest();
assertEquals("expectedResult", result);
}
š Call-to-Action: Start using this convenient approach to populate Spring @Value
during your unit tests without relying on a properties file. Simplify your test setup and keep your test cases separate from any changes in the properties file.
If you found this guide helpful, share it with your fellow developers to streamline their Spring unit testing process! Feel free to comment below and share your thoughts or any other clever ways you've solved this problem. Happy coding! š©āš»šØāš»
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
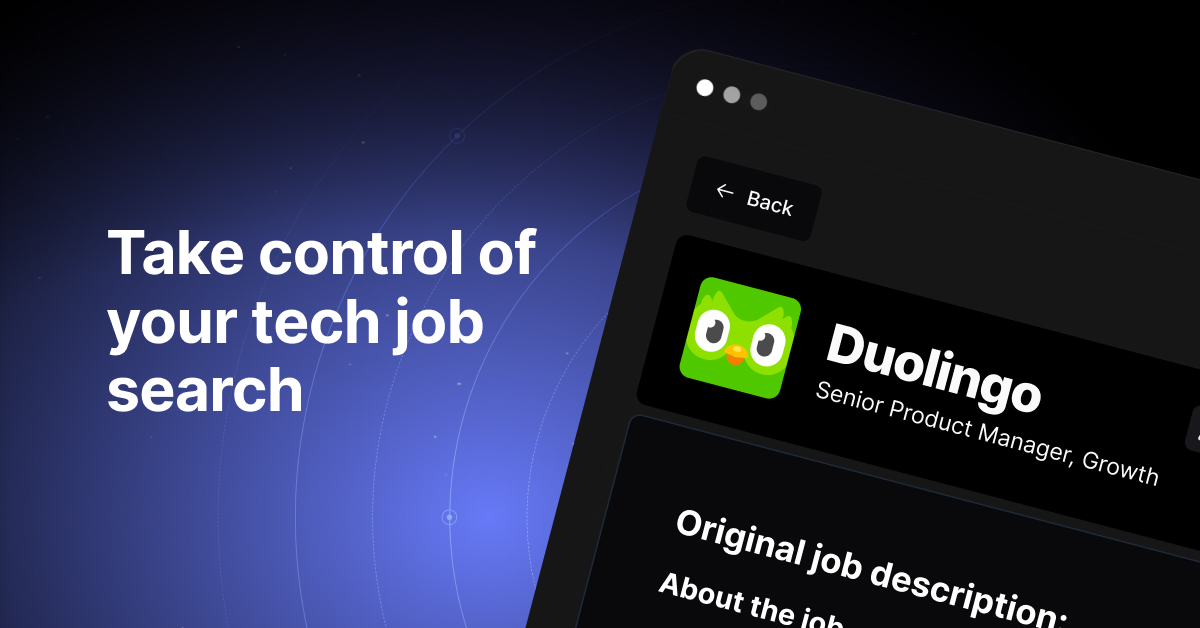