Passing multiple variables in @RequestBody to a Spring MVC controller using Ajax
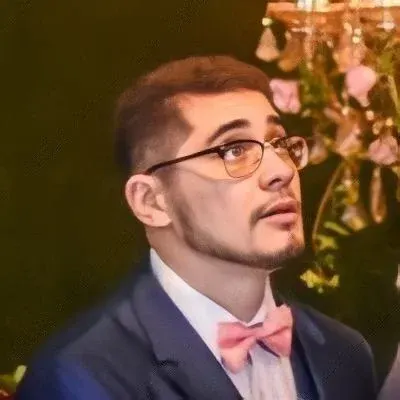
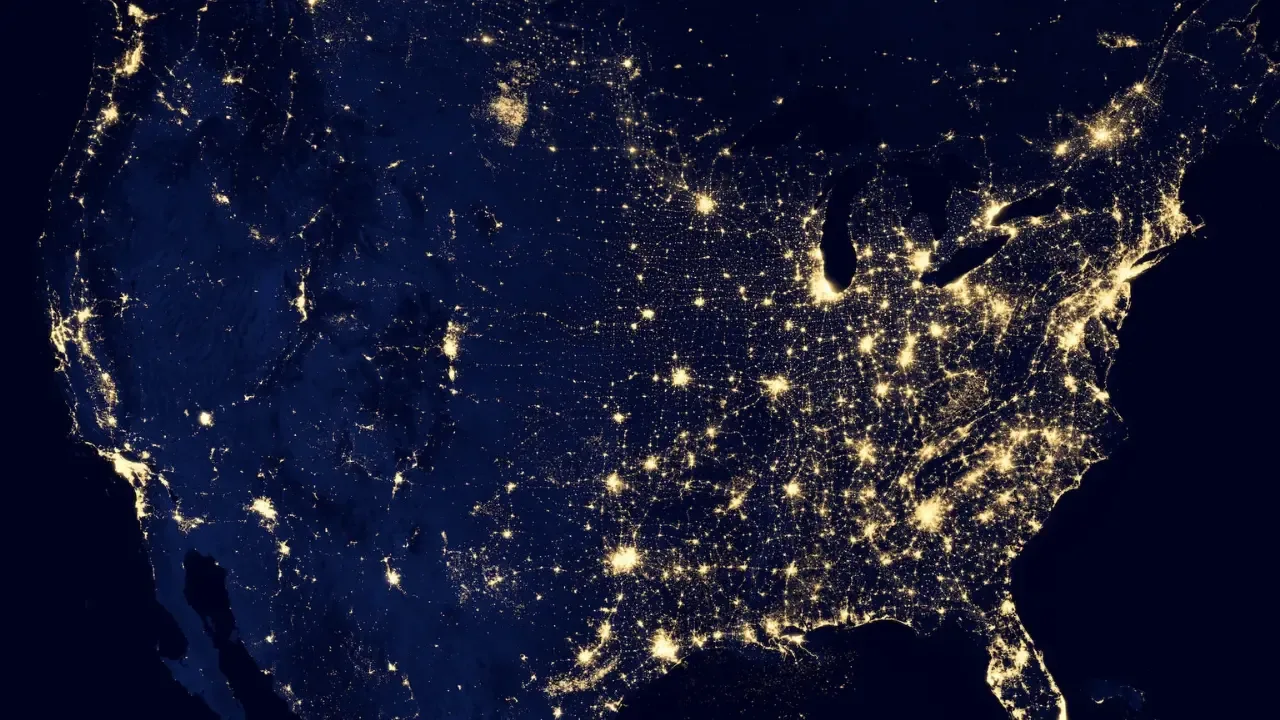
Passing Multiple Variables in @RequestBody to a Spring MVC Controller Using Ajax 😎
Are you struggling with passing multiple variables to a Spring MVC controller using @RequestBody and Ajax? 🤔 Don't worry, we've got you covered! In this blog post, we'll address this common issue and provide you with easy solutions. So, let's dive right in! 🚀
The Problem 😕
In the given context, the user wants to pass multiple variables, namely str1
and str2
, to a Spring MVC controller using @RequestBody. They initially attempted to do it like this:
@RequestMapping(value = "/Test", method = RequestMethod.POST)
@ResponseBody
public boolean getTest(@RequestBody String str1, @RequestBody String str2) {}
And they tried to use the following JSON:
{
"str1": "test one",
"str2": "two test"
}
However, this approach doesn't work as expected. 😢 Instead, they need to use a wrapper object to hold the variables in the request body.
The Solution ✅
To pass multiple variables to a Spring MVC controller using @RequestBody, you need to create a wrapper class. Let's call it Holder
for simplicity. Here's how you can modify your code:
@RequestMapping(value = "/Test", method = RequestMethod.POST)
@ResponseBody
public boolean getTest(@RequestBody Holder holder) {}
Now, the JSON to be sent in the request body would look like this:
{
"holder": {
"str1": "test one",
"str2": "two test"
}
}
Using this approach, you can easily access the str1
and str2
variables inside the getTest
method through the holder
object.
The Alternatives 🔄
If using a wrapper class doesn't suit your requirements, you have a couple of alternative options:
Change the
RequestMethod
toGET
and use@RequestParam
in the query string. However, keep in mind that the query string has limitations on the length and type of data that can be sent.Use
@PathVariable
with eitherRequestMethod.GET
orRequestMethod.POST
. This approach can work well when the variables are part of the URL in a RESTful manner.
Choose the alternative that best fits your scenario and requirements.
Conclusion 🎉
Passing multiple variables in @RequestBody to a Spring MVC controller using Ajax might be a little tricky at first. However, by using a wrapper class, you can easily overcome this challenge. We hope this guide has helped you understand the problem and provided you with simple and effective solutions. If you have any further questions or insights, feel free to leave a comment below. Happy coding! 😄
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
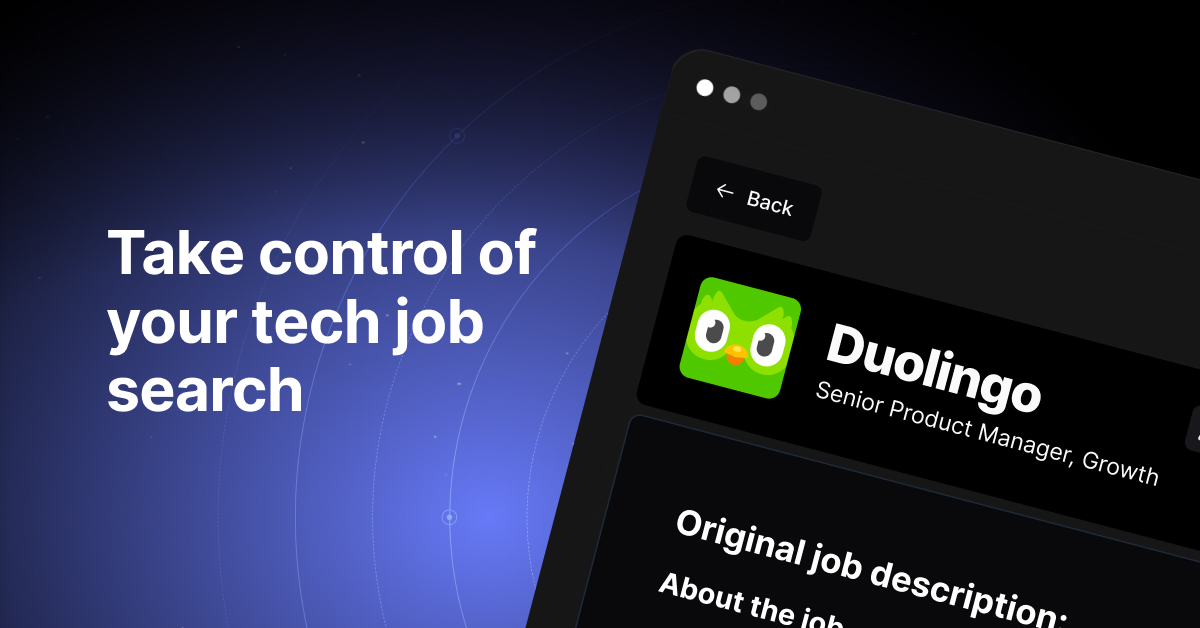