Only using @JsonIgnore during serialization, but not deserialization
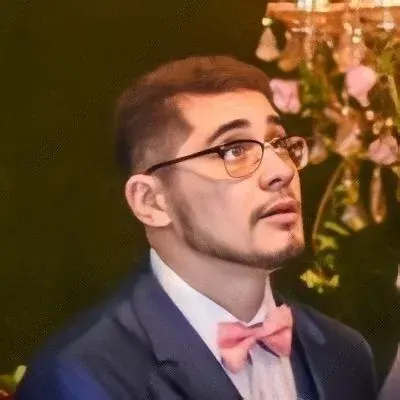
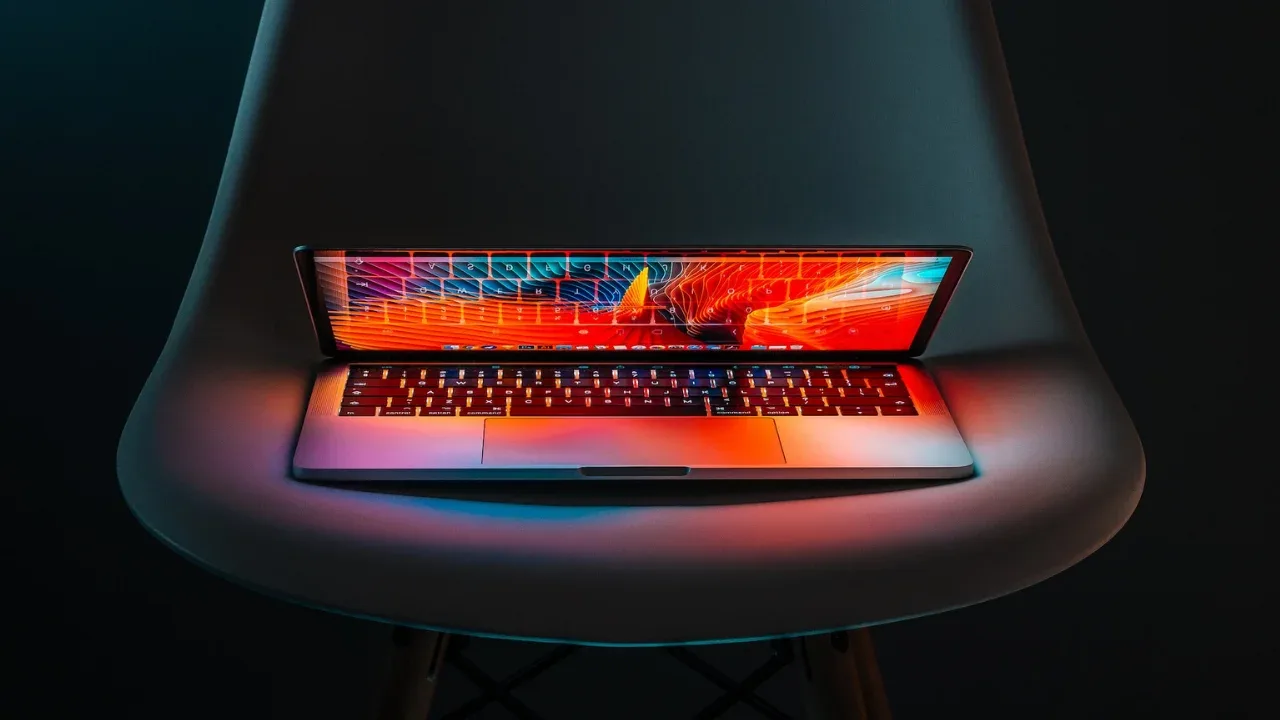
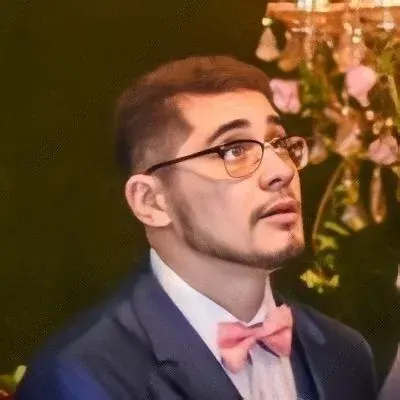
Understanding the Issue with @JsonIgnore during Serialization and Deserialization 🤔
So, you're facing a common issue with the @JsonIgnore
annotation. You want to exclude a certain property, like the hashed password, from being sent to the client during serialization. However, you also need the ability to deserialize the property when needed, like during user sign up. The problem is that @JsonIgnore
blocks the property from both serialization and deserialization. 🚫
The Challenge: Keeping @JsonIgnore for Serialization Only 😕
You mentioned that you're using Spring JSONView and have limited control over the ObjectMapper
. This means you need to find a solution without altering the ObjectMapper
directly. Don't worry, we've got you covered! 💪
Easy Solutions: A Two-Step Approach 🚀
To achieve serialization-only exclusion with @JsonIgnore
, you'll need to take a two-step approach. Let's go through the solutions you've tried and discuss a workaround that combines both for the desired result. 😉
Solution 1: Using @JsonIgnore on the Property
Your first attempt was to use @JsonIgnore
directly on the password property. While this works for serialization, it also prevents deserialization, making it impossible to sign up users with passwords. 😣
Solution 2: Using @JsonIgnore on the Getter Method
You then tried annotating only the getter method with @JsonIgnore
. While this allows deserialization to work properly, it fails to exclude the property during serialization. That's not the behavior you were looking for either. 😕
The Combined Solution: Property and Getter Method Annotation 🙌
By employing both annotation approaches, you can achieve serialization-only exclusion! Here's what you need to do:
Remove the
@JsonIgnore
annotation from the password property.Annotate the getter method of the password property with
@JsonIgnore
.
By annotating the getter method with @JsonIgnore
, the password property will be excluded during serialization. On the other hand, deserialization will still work, allowing users to sign up with passwords.
@JsonIgnore // Remove this annotation from the password property
public String getPassword() {
return this.password;
}
That's it! With this combined approach, you can serialize the user object without sending the hashed password to the client, while still being able to deserialize it when necessary during user sign up.
The Call-to-Action: Share Your Thoughts and Experiences! 💬
Have you encountered this issue before? Was the combined solution helpful to you? We'd love to hear from you! Share your thoughts, experiences, and any additional insights or alternative approaches you've found. Let's engage in a meaningful discussion and help each other solve tech challenges. 😊
Leave a comment below and let's get the conversation started! 👇