Mockito: Inject real objects into private @Autowired fields
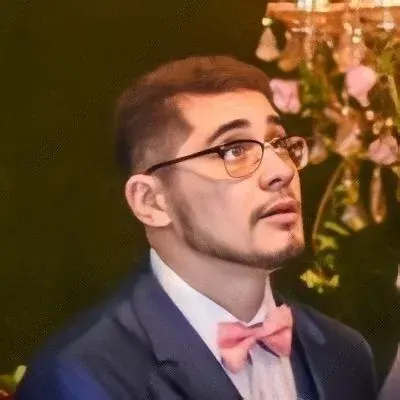
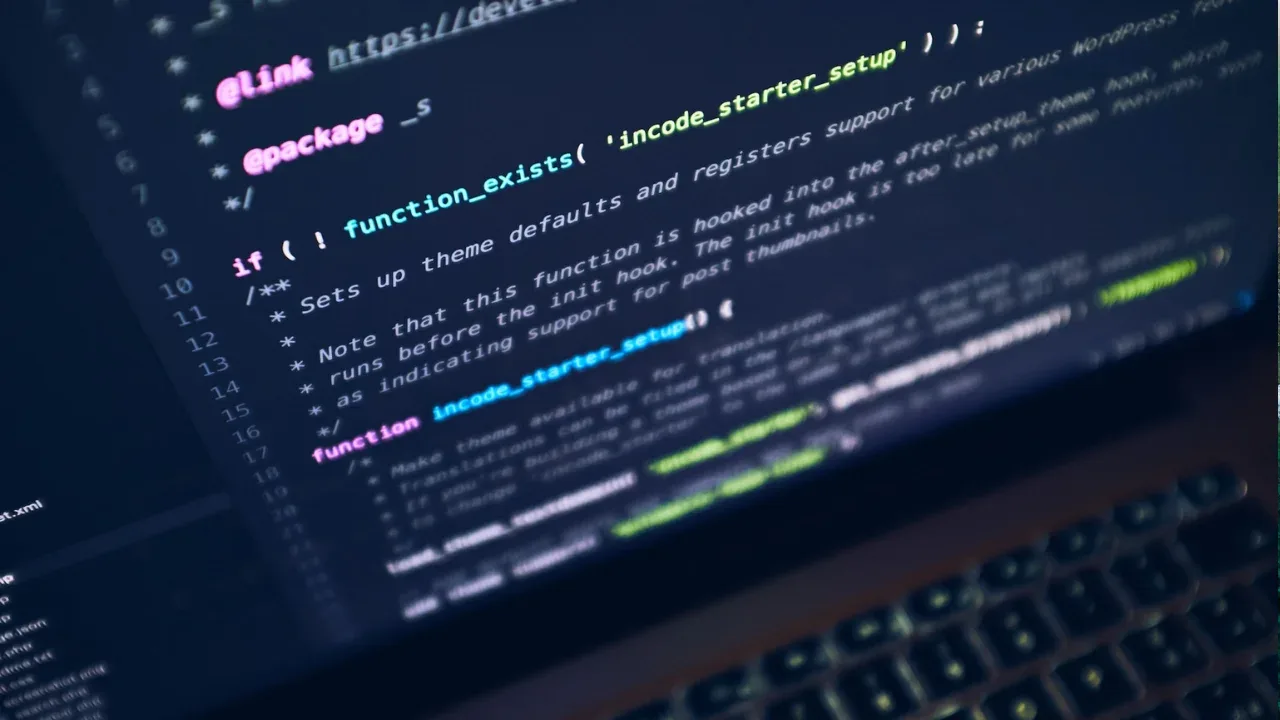
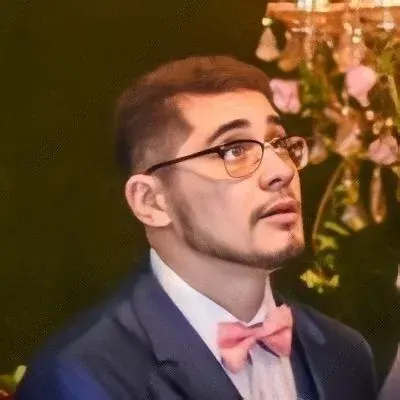
๐งช Testing Made Easy: Injecting Real Objects into Private @Autowired Fields with Mockito
Have you ever found yourself needing to inject real objects into private @Autowired
fields during testing with Mockito? Maybe you've been using the @Mock
and @InjectMocks
annotations to inject dependencies into your test class, but now you want to go a step further and inject actual objects. Good news! It's indeed possible, and I'm here to show you how. Let's dive in!
The Dilemma
The scenario is simple: you have a class, let's call it Demo
, with a private @Autowired
field, SomeService
. In your test class, DemoTest
, you are already using Mockito's @Mock
and @InjectMocks
annotations to inject mocked dependencies into Demo
. But now you're wondering if it's possible to inject real SomeService
objects into Demo
without exposing the field or creating a setter method.
@RunWith(MockitoJUnitRunner.class)
public class DemoTest {
@Mock
private SomeService service;
@InjectMocks
private Demo demo;
// ...
}
public class Demo {
@Autowired
private SomeService service;
// ...
}
The Solution
The good news is that Mockito provides a way to achieve this by using the @Spy
annotation in combination with the @InjectMocks
annotation. Here's how you can modify your code to inject real objects instead of mocks:
@RunWith(MockitoJUnitRunner.class)
public class DemoTest {
@Spy
@Autowired
private SomeService service;
@InjectMocks
private Demo demo;
// ...
}
By changing @Mock
to @Spy
in your DemoTest
class, Mockito will create a spy object that preserves the original functionality of SomeService
, allowing you to work with real instances of the class.
Putting It into Context
Let's take a closer look at what's happening here. When using @Mock
, Mockito replaces the SomeService
field in Demo
with a mock object during testing. This is handy when you want fine-grained control over the behavior of the dependency. However, if you want to work with the actual object, using the @Spy
annotation along with @Autowired
allows you to achieve just that.
Engage with the Community
Testing can be a complex topic, and sometimes it's challenging to find the best approach. That's why we encourage you to share your thoughts, experiences, and questions in the comments below. Engage with our vibrant community of tech enthusiasts, learn from their insights, and contribute to the collective knowledge.
Are there any other testing scenarios you'd like us to explore in future blog posts? Let us know! We're here to help you on your journey to becoming a testing pro.
So go ahead, put this solution to the test, and let us know how it works for you. Happy testing!
๐๐ป๐งช