Map enum in JPA with fixed values?
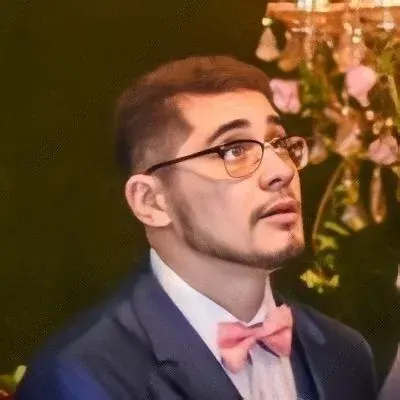
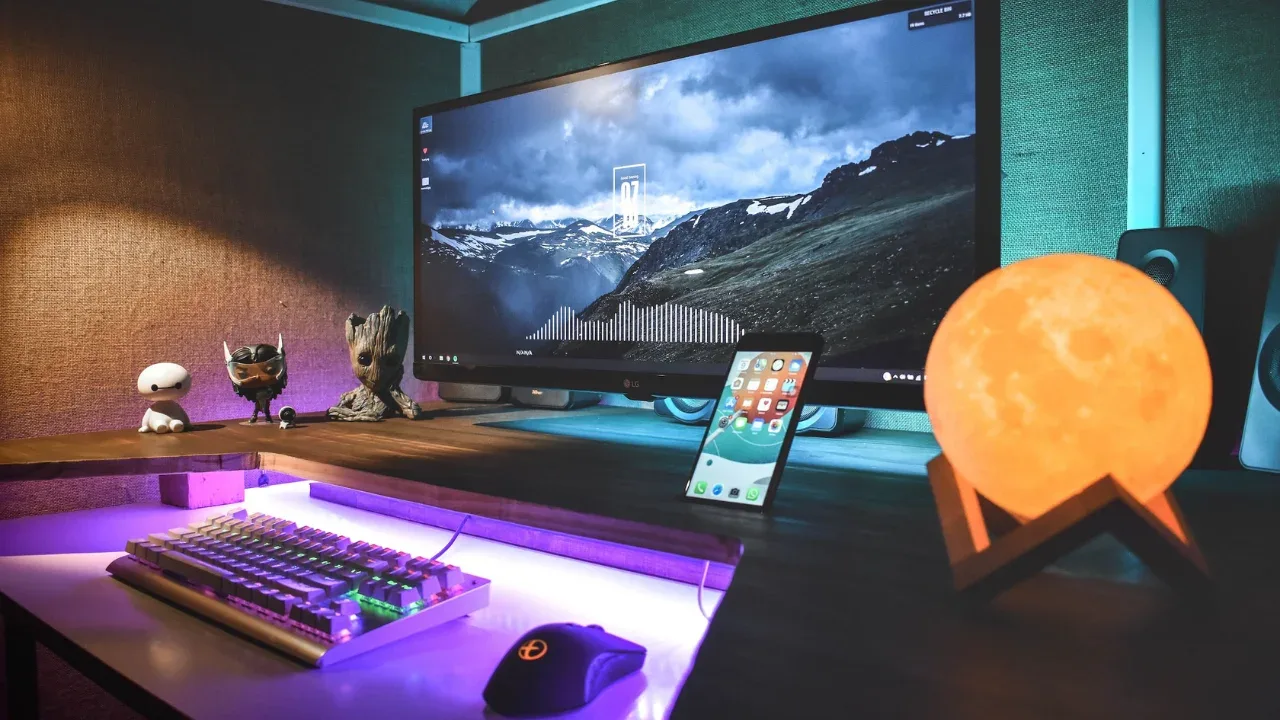
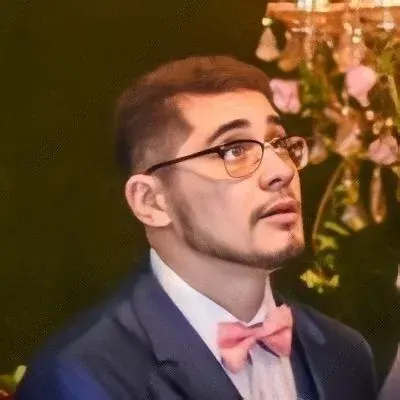
Mapping enums in JPA with fixed values πΊοΈ
Are you struggling with how to map enums with fixed values in JPA? Want to save only the integer value of each enum entry? Look no further, we've got you covered! In this blog post, we will explore different solutions to this common issue and provide easy-to-implement solutions. Let's dive in! π»π₯
The problem at hand π
A user on our platform was looking for different ways to map an enum using JPA. Specifically, they wanted to set the integer value of each enum entry and save only the integer value. Here's the context they provided:
@Entity
@Table(name = "AUTHORITY_")
public class Authority implements Serializable {
public enum Right {
READ(100), WRITE(200), EDITOR (300);
private int value;
Right(int value) { this.value = value; }
public int getValue() { return value; }
};
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
@Column(name = "AUTHORITY_ID")
private Long id;
// the enum to map :
private Right right;
}
As you can see, the user wants to map the Right
enum, but JPA maps the enum index (0, 1, 2) instead of the desired value (100, 200, 300). Let's move on to the solutions!
Solution 1: @Enumerated(EnumType.ORDINAL) - Not what we want π
A simple solution is to use the Enumerated
annotation with EnumType.ORDINAL
:
@Column(name = "RIGHT")
@Enumerated(EnumType.ORDINAL)
private Right right;
However, this maps the enum index and not the desired value. So, this solution won't work for us.
Solution 2: A bit complex, but it does the job! π
Fortunately, we have found two solutions that will fit our requirements. Let's start with the first one.
Solution 2a: @PrePersist and @PostLoad β¨
A solution proposed on the Java.net forums suggests using @PrePersist
and @PostLoad
annotations to convert the enum to another field and mark the enum field as transient. Here's how you can implement it:
@Basic
private int intValueForAnEnum;
@PrePersist
void populateDBFields() {
intValueForAnEnum = right.getValue();
}
@PostLoad
void populateTransientFields() {
right = Right.valueOf(intValueForAnEnum);
}
This solution ensures that the integer value is stored in the database while still allowing us to use the Right
enum in our code. It's a bit more complex, but it gets the job done!
Solution 2b: Using a conversion object π
Another solution, described on the AppFuse website, proposes using a generic conversion object. However, this solution seems more focused on Hibernate and may not exist in Java EE. Anyway, here's an example of how you can implement it:
@Type(
type = "org.appfuse.tutorial.commons.hibernate.GenericEnumUserType",
parameters = {
@Parameter(
name = "enumClass",
value = "Authority$Right"),
@Parameter(
name = "identifierMethod",
value = "toInt"),
@Parameter(
name = "valueOfMethod",
value = "fromInt")
}
)
This solution might be heavier and more Hibernate-oriented, so make sure to check if it fits your project requirements.
Looking for alternative solutions π€
While the above solutions are effective, you might be wondering if there are other ways to tackle this problem. Here are a few ideas that you can explore:
Use setter and getter methods of the
right
member in theAuthority
class when loading and saving theAuthority
object.Define specific conversion methods within the
Right
enum itself to convert between the enum and integer values.Leverage Spring's capabilities to define a custom converter (e.g.,
RightEditor
) and instruct JPA to use it.
These ideas are worth exploring, but make sure to consider the implications and compatibility with your project's tech stack.
Conclusion π
Mapping enums with fixed values in JPA can be a bit tricky, but with the right solutions, it becomes a piece of cake! In this blog post, we explored two effective solutions to address this problem. Additionally, we discussed alternative ideas that you can explore.
Now, it's your turn! Give these solutions a try and let us know in the comments which one worked best for you. Have any other suggestions or questions? We'd love to hear from you! Let's engage in a meaningful discussion and share our knowledge. Happy coding! π»π