JsonMappingException: No suitable constructor found for type [simple type, class ]: can not instantiate from JSON object
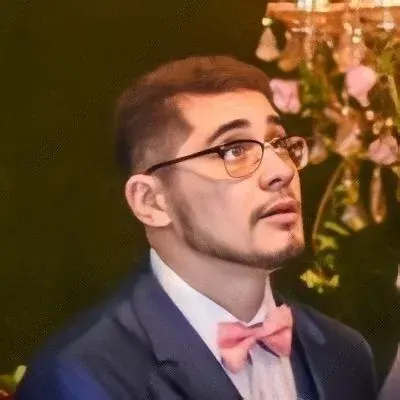
![Cover Image for JsonMappingException: No suitable constructor found for type [simple type, class ]: can not instantiate from JSON object](https://images.ctfassets.net/4jrcdh2kutbq/376eUYzJAW7m4wV7PLqKoF/686c0d07d68f4a0bd05ecf1a1ec4bd01/Untitled_design__17_.webp?w=3840&q=75)
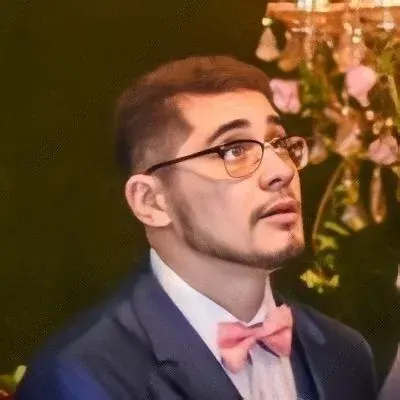
How to Fix JsonMappingException
If you're encountering the dreaded JsonMappingException: No suitable constructor found for type [simple type, class ]: can not instantiate from JSON object
error, don't fret! 🤔 I've got your back. In this blog post, I'll walk you through common issues that cause this error and provide easy solutions to fix it. Let's dive in!
The Error and its Context
Here's the error message that's causing all the trouble:
org.codehaus.jackson.map.JsonMappingException: No suitable constructor found for type [simple type, class com.myweb.ApplesDO]: can not instantiate from JSON object (need to add/enable type information?)
The error occurs when you're trying to receive and process a JSON request. Here's an example of the JSON you're sending:
{
"applesDO" : [
{
"apple" : "Green Apple"
},
{
"apple" : "Red Apple"
}
]
}
You have a controller method that is supposed to handle this request:
@RequestMapping("showApples.do")
public String getApples(@RequestBody final AllApplesDO applesRequest){
// Method Code
}
AllApplesDO
is a wrapper class for ApplesDO
, which looks like this:
public class AllApplesDO {
private List<ApplesDO> applesDO;
// Getters and setters
// Constructor
}
public class ApplesDO {
private String apple;
// Getter and setter
public ApplesDO(CustomType custom){
// Constructor Code
}
}
The Root Cause
The root cause of the JsonMappingException
error is most likely the absence of a suitable constructor in your ApplesDO
class. Jackson is unable to instantiate the class from the JSON object. This often occurs when you have a subclass that Jackson cannot convert into Java objects.
The Solution
To fix this issue, you need to add or enable type information for Jackson to properly convert JSON into Java objects. Here's what you can do:
Add a default constructor to your
ApplesDO
class:
public class ApplesDO {
private String apple;
// Getter, setter, and default constructor
public ApplesDO(){
// Default constructor code
}
public ApplesDO(CustomType custom){
// Constructor Code
}
}
By adding a default constructor, Jackson will be able to instantiate the ApplesDO
class using reflection when converting JSON into Java objects.
Enable Jackson's type information handling:
In your Spring configuration, make sure you have the following properties set:
spring.jackson.deserialization.fail-on-unknown-properties=false
spring.jackson.mapper.enable-default-typing=true
The enable-default-typing
property enables Jackson's type information handling, which allows the conversion of JSON objects into Java objects, even if the class is a subclass.
Conclusion
Congratulations! You've successfully fixed the JsonMappingException
error. 🎉 By adding a default constructor to your class and enabling Jackson's type information handling, you've ensured that JSON objects can be seamlessly converted into Java objects.
If you found this article helpful, why not share it with your fellow developers who might be facing the same issue? 🌟 And if you have any questions or additional tips, feel free to leave a comment below. Let's keep the conversation going!
Happy coding! 👩💻👨💻