Jaxb, Class has two properties of the same name
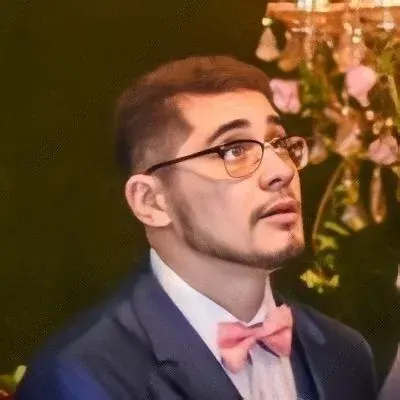
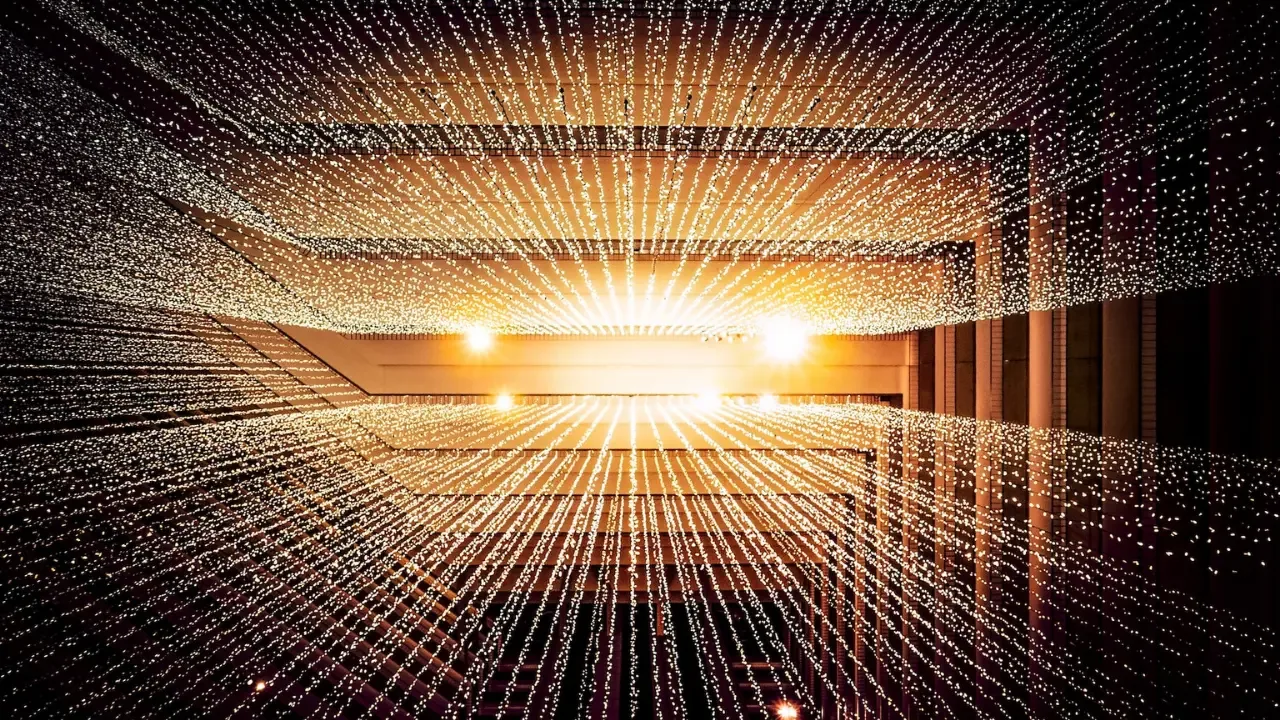
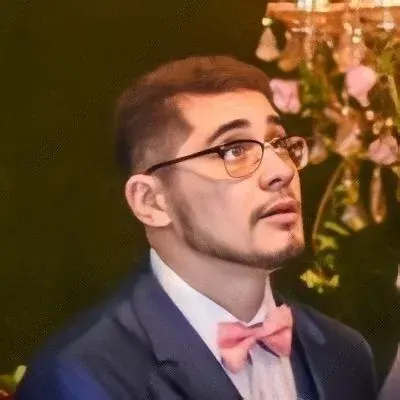
Title: Understanding JAXB: Handling properties with the same name in a class
Hey there! 😎 Have you ever encountered an error when using JAXB 📦, where a class has two properties with the same name? 🤔 It can be frustrating, especially when you're not sure how to fix it. In this blog post, we'll dive into this common issue and provide you with easy solutions to prevent it from happening. 🛠️
The Problem: Class has two properties of the same name
Let's take a look at the specific example that triggered this error. A user was trying to read an XML file using JAXB, which contained a class called ModeleREP
. This class had a property named timeSeries
. However, upon running the code, the user encountered the following error:
com.sun.xml.internal.bind.v2.runtime.IllegalAnnotationsException: 1 counts of IllegalAnnotationExceptions
Class has two properties of the same name "timeSeries"
The error message clearly states that the class ModeleREP
has two properties named timeSeries
. One is a public getter method, and the other is a protected instance variable.
Understanding the error
JAXB is a powerful library that maps XML to Java objects and vice versa. To do this, it relies on annotations to determine how to map XML elements to Java properties. In this case, when JAXB tries to map the XML element <TimeSeries>
to the timeSeries
property in the ModeleREP
class, it gets confused because there are two potential mappings with the same name.
Easy Solution: Use consistent access type annotations
To fix this error, we need to provide JAXB with unambiguous instructions on how it should map the XML elements. We can achieve this by using consistent access type annotations. 📝
In the example provided, the timeSeries
property in the ModeleREP
class has both a public getter method and a protected instance variable. To resolve the issue, we can follow these steps:
Remove the access type annotation from the
ModeleREP
class. This will ensure that JAXB uses the default access type, which is determined by the presence of either field or property annotations.@XmlRootElement(name = "ModeleREP", namespace = "urn:test:mod_rep.xsd") public class ModeleREP { // existing code }
Add the
@XmlElement
annotation to the public getter method of thetimeSeries
property.@XmlAccessorType(XmlAccessType.FIELD) @XmlRootElement(name = "TimeSeries") public class TimeSeries { @XmlElement(name = "ResourceObject") protected RessourceObject resourceObject; // existing code }
Remove the
@XmlElement
annotation from the protected instance variable.// existing code @XmlElement(name = "Period") protected Period period; // existing code
By making these changes, you explicitly tell JAXB to use the public getter method to map the XML element, ensuring that the naming conflict is resolved.
Preventing null values in timeSeries property
Now that we have fixed the naming conflict issue, let's address another concern mentioned by the user: sometimes the timeSeries
property is returning null.
To prevent null values in the timeSeries
property, we can add a null check and initialization code in the getTimeSeries()
method of the ModeleREP
class. Here's an example:
public List<TimeSeries> getTimeSeries() {
if (this.timeSeries == null) {
this.timeSeries = new ArrayList<TimeSeries>();
}
return this.timeSeries;
}
By initializing the timeSeries
property with an empty ArrayList
if it is null, we ensure that the property always has a non-null value. This can prevent potential null pointer exceptions and make your code more robust.
Conclusion and Call-to-Action
Congratulations! 🥳 You've successfully tackled the issue of a class having two properties with the same name when using JAXB. By using consistent access type annotations and performing a null check and initialization, you can overcome these challenges and achieve smooth XML-to-Java mapping.
I hope you found this guide helpful and that it resolved your JAXB issue. If you have any questions or need further assistance, feel free to leave a comment below. And don't forget to share this blog post with others who might find it useful! Let's spread the knowledge together. 👍✉️
Now, go ahead, update your code, and experience seamless XML processing with JAXB! 🚀