Java string to date conversion
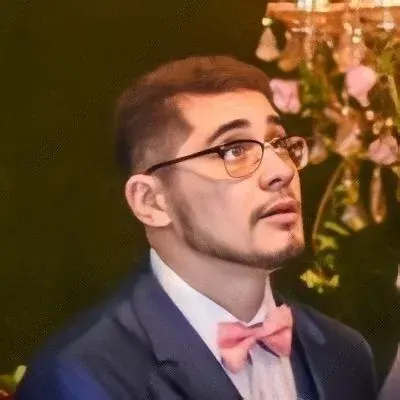
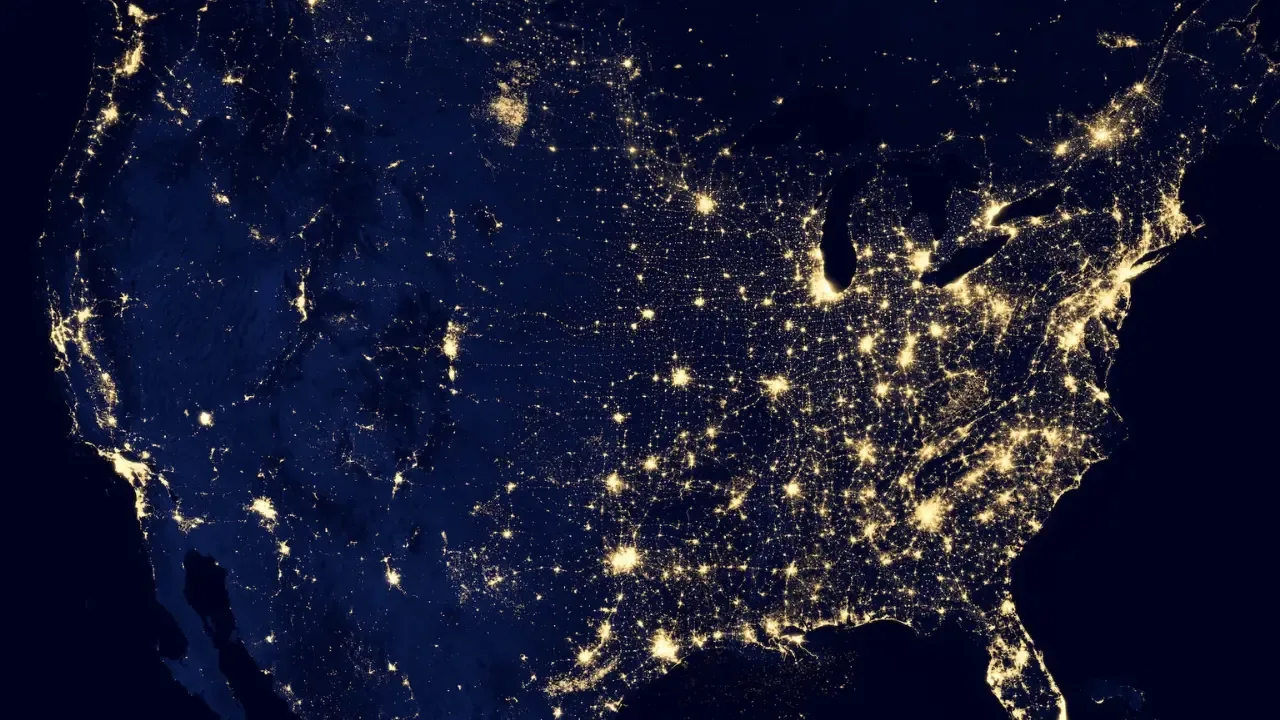
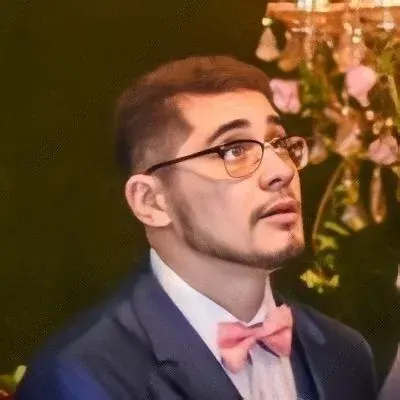
Converting a Java String to a Date: A Handy Guide 📅
So, you've stumbled upon a puzzling problem: how do you convert a Java String in the format 'January 2, 2010' to a Date type? Fear not, fellow developer! In this guide, we'll explore common pitfalls, provide easy solutions, and equip you with the knowledge you need to conquer this conversion challenge. 🚀
The Challenge: String to Date Conversion 🤷♂️
Let's dive right into the problem at hand. You have a String representing a date in the format 'January 2, 2010', and you want to convert it into a usable Date object. Additionally, you'd like to extract the individual month, day, and year values as integers for further manipulation. Sound familiar? We thought so too! 💡
Common Issues & Easy Solutions 💡
Issue 1: Parsing the String to a Date object 📝
The first step towards transforming your String into a Date object is parsing. Java provides the SimpleDateFormat
class to make the process a breeze. Let's see an example:
import java.text.SimpleDateFormat;
import java.util.Date;
String dateString = "January 2, 2010";
SimpleDateFormat formatter = new SimpleDateFormat("MMMM d, yyyy");
Date date = formatter.parse(dateString);
In this snippet, we create a SimpleDateFormat
object and specify the pattern that matches our date format. The pattern "MMMM d, yyyy" accounts for the full month name, day with or without leading zeroes, and the full year. The parse()
method is then used to convert the String into a Date object. Easy peasy! 🙌
Issue 2: Extracting the month, day, and year as integers 📆
Now that you have your Date object, extracting the month, day, and year values as integers is a breeze. Here's an example:
import java.util.Calendar;
Calendar calendar = Calendar.getInstance();
calendar.setTime(date);
int month = calendar.get(Calendar.MONTH) + 1; // Adding 1, as months are zero-based
int day = calendar.get(Calendar.DAY_OF_MONTH);
int year = calendar.get(Calendar.YEAR);
By utilizing the Calendar
class, we can easily access the desired components of our Date object. The getInstance()
method returns a Calendar instance, and setTime()
allows us to set the Date we obtained earlier. From there, we can use various predefined constants (e.g., Calendar.MONTH
, Calendar.DAY_OF_MONTH
, Calendar.YEAR
) to extract the desired values. Voila! 🎉
Your Time to Shine ⏰
Congratulations! You've successfully converted a Java String to a Date and extracted the month, day, and year as integers. Now, unleash your developer skills and integrate these newfound techniques into your projects. 🚀
But hey, before you go, we'd love to hear your thoughts! Have you encountered any roadblocks in your Date conversions? Do you have any pro tips to share with the developer community? Drop a comment below and let's spark a meaningful conversation! 👇
Happy coding! 💻🔥