Java string split with "." (dot)
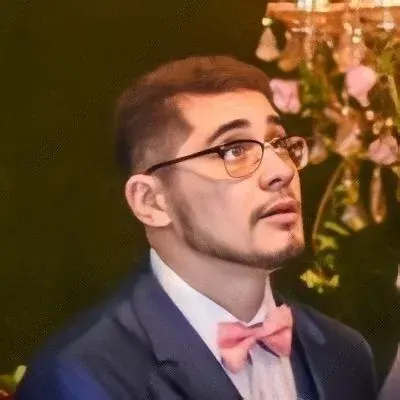
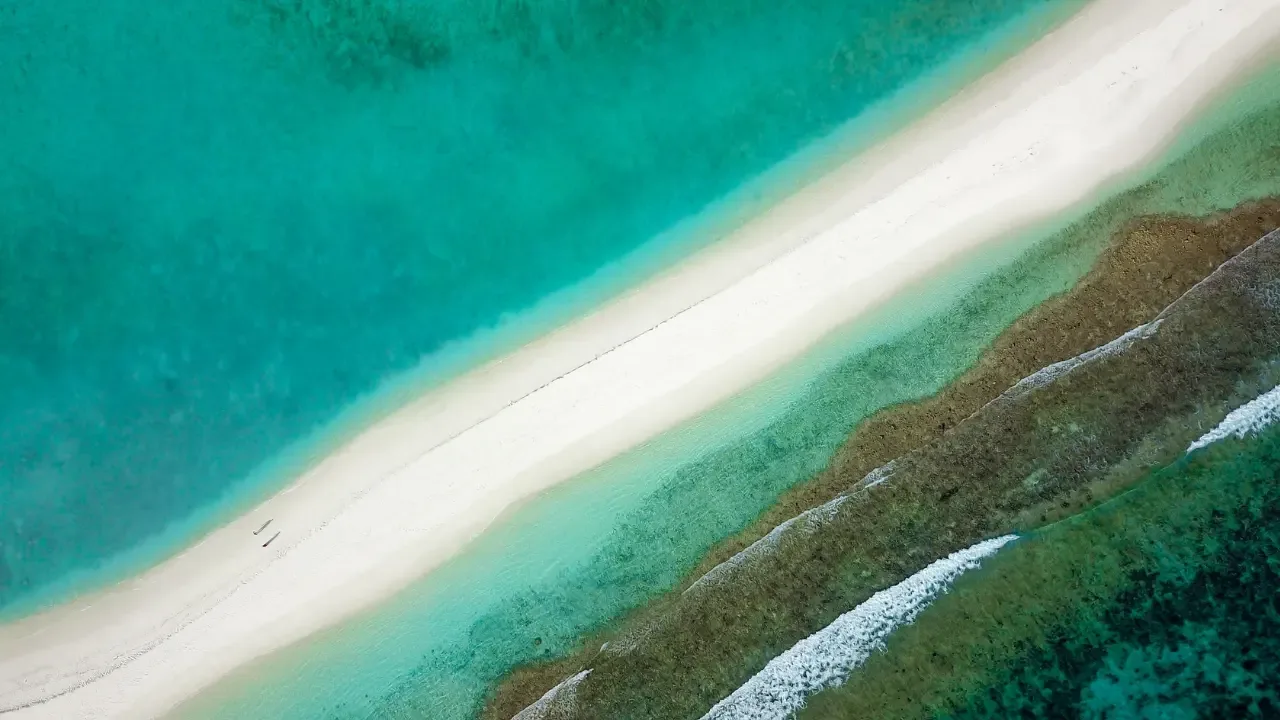
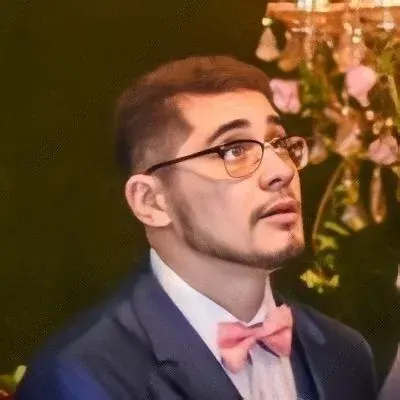
Understanding the Java String Split with "."
Hello there, tech enthusiasts! 👋 Welcome to another exciting blog post where we dive into the world of Java programming. Today, we're going to address a common issue with the Java string split method when using the dot (".") as the delimiter.
So here's the scenario: you have a file path stored in a string variable called filename
. You want to extract the name of the file without the extension. Seems pretty straightforward, right? Let's take a look at the code snippet provided and understand why it throws an ArrayIndexOutOfBoundsException
.
String filename = "D:/some folder/001.docx";
String extensionRemoved = filename.split(".")[0];
In this code, the aim is to split the filename
string using the dot as the delimiter and store the first part (with the extension removed) in the extensionRemoved
variable. However, when you run this code, it throws an ArrayIndexOutOfBoundsException
. 🚫
The reason for this exception is a bit tricky, but fear not! We're here to guide you through it.
The Issue: Regular Expression Metacharacters
The problem lies in the fact that the split
method treats the dot (".") as a regular expression metacharacter. 😱 In regular expressions, a dot matches any character, so when you try to split the string using the dot as the delimiter, it splits the string after every single character, resulting in an array with numerous elements.
To better illustrate this, let's see what happens when we print the array after splitting the string:
String[] parts = filename.split(".");
System.out.println(Arrays.toString(parts));
Output:
[, D, :, /, s, o, m, e, , f, o, l, d, e, r, /, 0, 0, 1, ., d, o, c, x]
As you can see, the string is split into individual characters, rather than splitting it at the dot. This is the reason why the subsequent [0]
index access in filename.split(".")[0]
throws an ArrayIndexOutOfBoundsException
, as the resulting array has many elements.
The Solution: Escape the Dot
To fix this issue, we need to escape the dot metacharacter by using a backslash before it. This tells Java to treat the dot as a literal dot and not a metacharacter.
Let's update our code snippet and see the magic:
String extensionRemoved = filename.split("\\.")[0];
By adding the double backslash \\
before the dot, we are effectively escaping the dot, and the split
method will now split the string at the actual dot character, as intended. Voila! 🎩✨
The Easier Approach: Using substring
If using the split
method seems a bit overkill for your specific use case, there's an even simpler approach using the substring
method. Instead of splitting the string, you can find the position of the dot and extract the substring from the beginning till that position.
Here's how you can achieve it:
int dotIndex = filename.lastIndexOf(".");
String extensionRemoved = filename.substring(0, dotIndex);
By finding the last occurrence of the dot using lastIndexOf(".")
, we get the index position of the dot. We can then extract the substring from the beginning till that position using the substring
method. Easy peasy! 🙌
Wrapping It Up
We've covered a common issue encountered while using the dot as a delimiter in the Java split
method. We explained why the exception occurs and provided easy solutions to overcome it. Now you're all set to extract filenames without extensions like a pro! 💪
If you found this blog post helpful, be sure to share it with your fellow Java developers who might be facing a similar issue. And don't hesitate to leave a comment below if you have any questions or additional insights. We love hearing from you! 😊✨
Happy coding! 🚀🎉