Java 8 List<V> into Map<K, V>
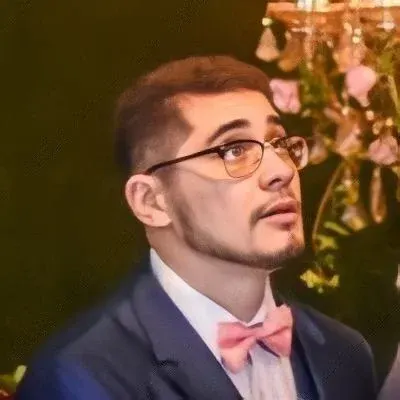
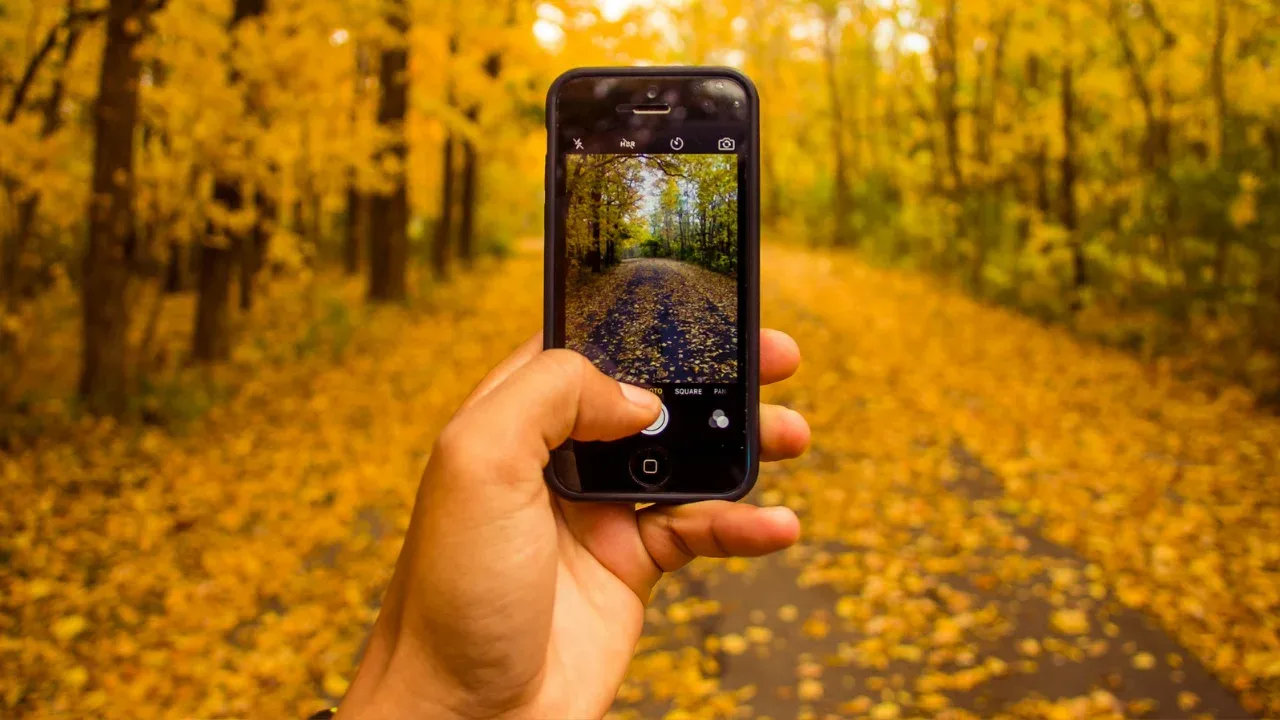
📝Easy Java 8 Solution: Converting List<V> into Map<K, V> 🚀
So, you want to translate a List of objects into a Map using Java 8's streams and lambdas? No worries, I've got you covered! Let's dive into it and explore different approaches to solve this problem.
The Java 7 Solution
Before we jump into the Java 8 magic, let's take a look at how this could be achieved in Java 7 and below. Here's an example:
private Map<String, Choice> nameMap(List<Choice> choices) {
final Map<String, Choice> hashMap = new HashMap<>();
for (final Choice choice : choices) {
hashMap.put(choice.getName(), choice);
}
return hashMap;
}
The Guava Solution
Now, let's see how this could be done using Guava, a popular Java library. Guava provides a convenient method called uniqueIndex
that allows us to create a Map from a List using a provided function. Here's the Guava solution:
private Map<String, Choice> nameMap(List<Choice> choices) {
return Maps.uniqueIndex(choices, new Function<Choice, String>() {
@Override
public String apply(final Choice input) {
return input.getName();
}
});
}
The Guava Solution with Java 8 Lambdas
Finally, if you already have Java 8 in your project, you can take advantage of lambdas to simplify the Guava solution even further. Here's how it looks:
private Map<String, Choice> nameMap(List<Choice> choices) {
return Maps.uniqueIndex(choices, Choice::getName);
}
✨ And there you have it! Three different approaches to convert a List into a Map using Java 8, Guava, and Java 8 with Guava lambdas.
🌟 Do It Without Guava
But what if you want to achieve the same result without using Guava? Fear not, my friend! Java 8 streams and lambdas can come to the rescue. Here's how you can do it:
private Map<String, Choice> nameMap(List<Choice> choices) {
return choices.stream()
.collect(Collectors.toMap(Choice::getName, Function.identity()));
}
With this approach, we utilize the Collectors.toMap
method from Java 8 streams to create the desired Map by specifying the key and value functions. Super cool, right? 😎
🚀 Time to Take Action!
Now that you've learned about different ways to convert a List into a Map using Java 8, it's time to put your knowledge into action! Try out these solutions in your project and see which one fits your needs the best.
✨ Remember, Java 8 is all about convenience, readability, and making your life as a developer easier. So, embrace the power of lambdas and streams to level up your coding game! 🌟
👉 Now it's your turn! Share your thoughts and which solution you found most useful in the comments below. Let's start a conversation and learn from each other! 💬😃
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
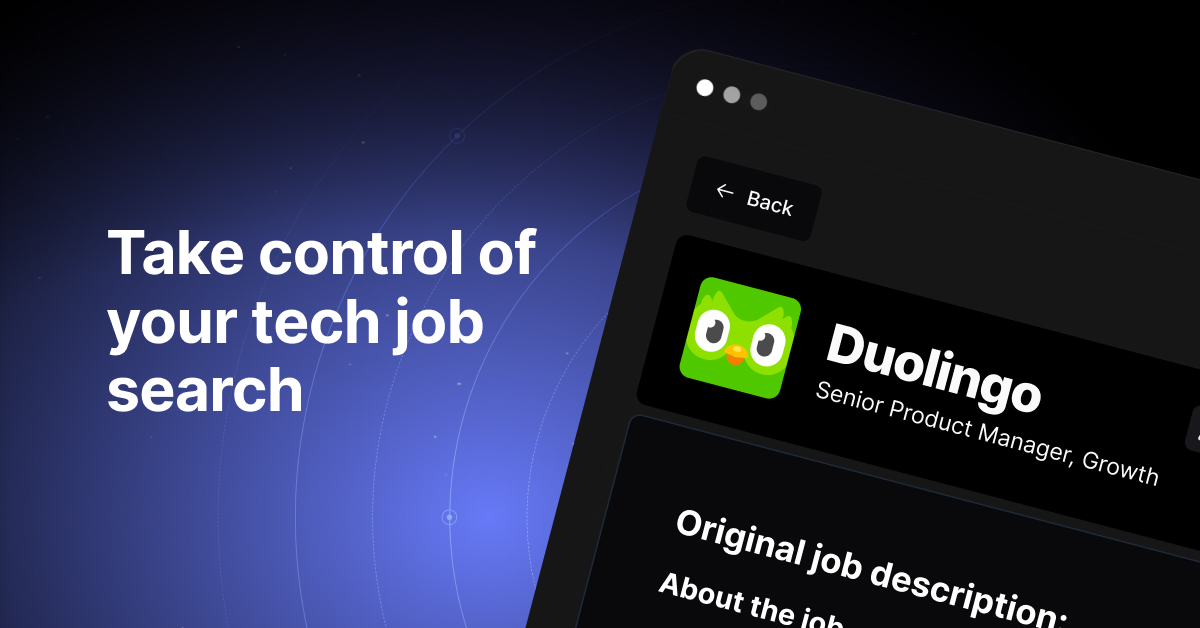