Jackson with JSON: Unrecognized field, not marked as ignorable
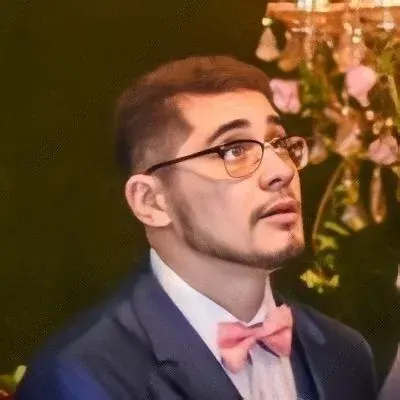
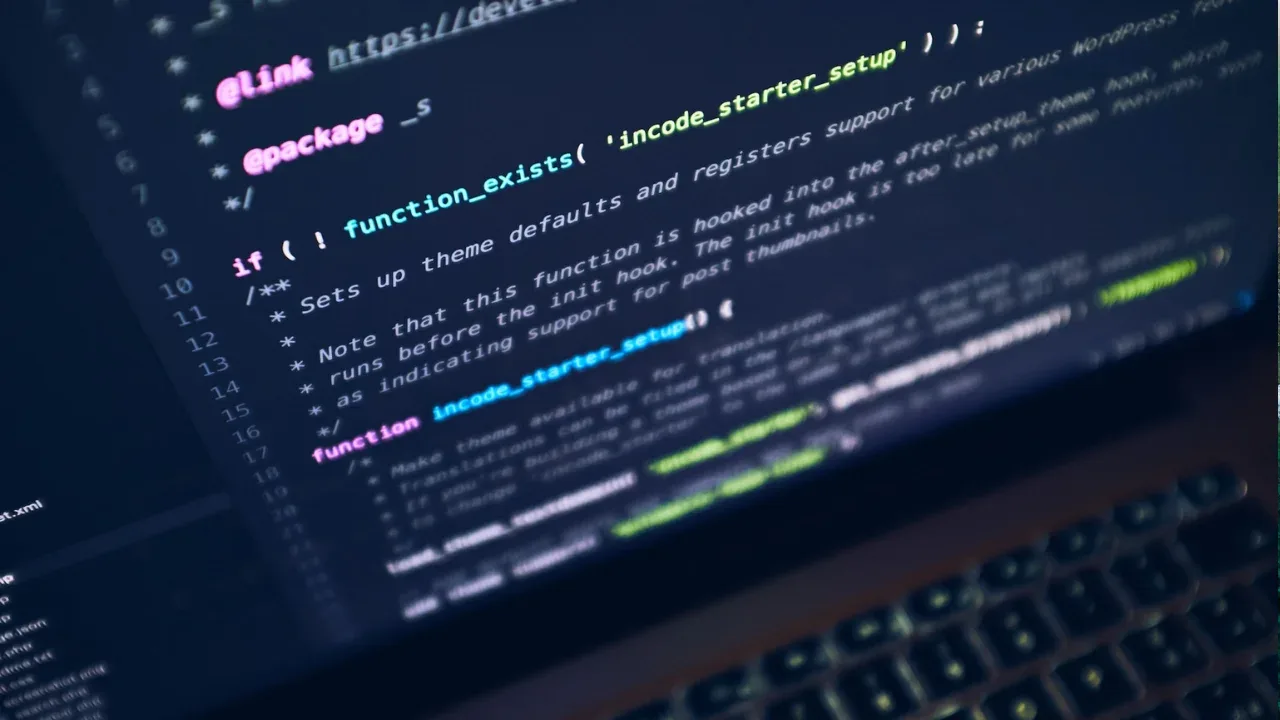
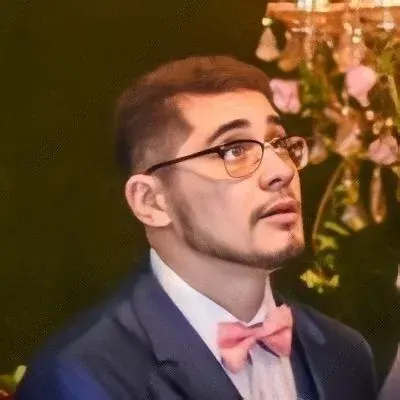
Jackson with JSON: Unrecognized field, not marked as ignorable 樂
If you're working with JSON in Java using Jackson and you encounter the error "Unrecognized field, not marked as ignorable", fear not! This blog post will guide you through common issues and provide easy solutions to help you overcome this problem. 🔥
Understanding the problem
The error message suggests that there is an unrecognized field in the input JSON that is not marked as ignorable. In other words, Jackson doesn't know how to map the JSON field to your Java object because it doesn't exist in your class definition or isn't properly annotated.
In the provided code snippet, you're trying to map the JSON string to a Wrapper
object. The Wrapper
class contains a list of Student
objects. However, the error message indicates that Jackson doesn't recognize the field "wrapper" in the JSON.
Finding the solution
To solve this problem, you need to make sure that the field names in your Java class match the names in the JSON string exactly. In your case, the issue lies with the field name "students" in the Wrapper
class.
To fix this, you can either change the field name in the Wrapper
class to "wrapper" or use Jackson annotations to map the JSON field to the Java field.
Option 1: Change the field name
Here's how you can modify your Wrapper
class:
public class Wrapper {
private List<Student> wrapper;
// getters & setters here
}
By changing the field name to "wrapper", Jackson will be able to map the JSON field correctly.
Option 2: Use Jackson annotations
If you prefer to keep the field name as "students" in your Java class, you can use Jackson annotations to specify the mapping:
public class Wrapper {
@JsonProperty("wrapper")
private List<Student> students;
// getters & setters here
}
By adding the @JsonProperty("wrapper")
annotation, Jackson will recognize the JSON field as "wrapper" and map it to the students
field in your Java class.
Putting it all together
With the necessary changes made, let's update the code snippet:
private void tryReading() {
String jsonStr = "{\"wrapper\":[{\"id\":\"13\",\"name\":\"Fred\"}]}";
ObjectMapper mapper = new ObjectMapper();
Wrapper wrapper = null;
try {
wrapper = mapper.readValue(jsonStr, Wrapper.class);
} catch (Exception e) {
e.printStackTrace();
}
System.out.println("wrapper = " + wrapper);
}
Now, when you run the code, it should successfully parse the JSON string into a Wrapper
object without throwing the "Unrecognized field, not marked as ignorable" error.
Share your success story and engage with the community!
We hope this guide helped you overcome the "Unrecognized field, not marked as ignorable" issue when working with Jackson and JSON in Java. If you found this blog post useful, don't forget to share it with your developer friends who might encounter similar problems. Together, let's make coding easier for everyone! ✨
Have you encountered any other issues with Jackson and JSON? Share your experiences or ask questions in the comments section below. Let's learn from each other and grow together as a developer community! 🌟