Iterating through a Collection, avoiding ConcurrentModificationException when removing objects in a loop
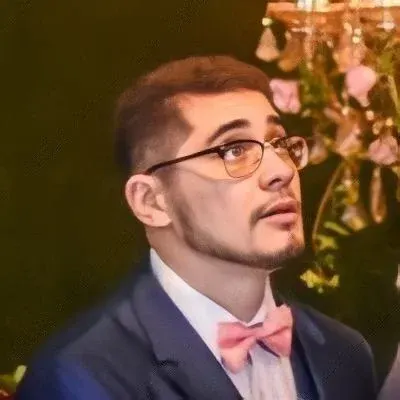

Iterating through a Collection without getting a ConcurrentModificationException
π©Hey there tech enthusiasts! Are you tired of encountering the dreaded ConcurrentModificationException
when trying to remove objects from a collection while iterating through it? Well, fret no more! In this blog post, we will tackle this common issue and provide you with easy solutions to overcome it. So, buckle up and let's dive in! πββοΈ
Understanding the Problem
We all know that the straightforward approach of removing objects from a collection while iterating through it causes a ConcurrentModificationException
. This exception is thrown to prevent concurrent access to the collection by multiple threads, but it can also occur when you modify the collection inside a loop without using the proper techniques.
Consider this example:
for (Object i : l) {
if (condition(i)) {
l.remove(i);
}
}
π€ At first glance, this seems like a reasonable way to remove objects from the collection. However, it's a big NO-NO, my friend! This code will lead to a ConcurrentModificationException
because you're modifying the collection while iterating through it, and the collection is not prepared for it.
Approaches to Avoid ConcurrentModificationException
1. Using Iterator
To successfully remove objects from a collection while iterating, one common solution is to use an Iterator
. Here's the modified code using an Iterator
:
Iterator<Object> iterator = l.iterator();
while (iterator.hasNext()) {
Object i = iterator.next();
if (condition(i)) {
iterator.remove();
}
}
π By using an Iterator
, we ensure that we're making modifications to the collection in a way that the iterator can handle. Calling iterator.remove()
removes the current object, eliminating the possibility of a ConcurrentModificationException
.
2. Collecting Items to Remove
Another approach is to collect the items that need to be removed into a separate collection and then remove them outside the loop. Here's an example:
List<Object> itemsToRemove = new ArrayList<>();
for (Object i : l) {
if (condition(i)) {
itemsToRemove.add(i);
}
}
l.removeAll(itemsToRemove);
π By storing the objects that need to be removed in a separate collection, we avoid modifying the original collection while iterating through it. After iterating, we can simply remove all the items using l.removeAll(itemsToRemove)
.
3. Using Java 8 Stream API
If you're using Java 8 or above, you can leverage the Stream API to filter out the unwanted items and collect the resulting collection. Here's an example:
l = l.stream()
.filter(i -> !condition(i))
.collect(Collectors.toList());
π Using the powerful Stream API, we can apply a filter to exclude the items that don't meet our condition. The collect(Collectors.toList())
method collects the filtered items into a new list, effectively removing the unwanted items from the collection.
Wrap-up and Your Turn to Shine! π‘
Congratulations! You've now learned three effective ways to remove objects from a collection without encountering the ConcurrentModificationException
. Whether you choose to use an Iterator
, collect items to remove, or utilize the Stream API, you now have the knowledge to handle this notorious problem like a pro.
β¨ Your mission, if you choose to accept it, is to experiment with these techniques, try them out in your code, and let us know which one worked best for you! Share your experiences, thoughts, or alternate solutions in the comments below. Let's learn and grow together! π±
Remember, the path to becoming a skilled developer is all about embracing challenges and continuously expanding your knowledge. Stay curious, keep coding, and until next time, happy programming! βοΈπ
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
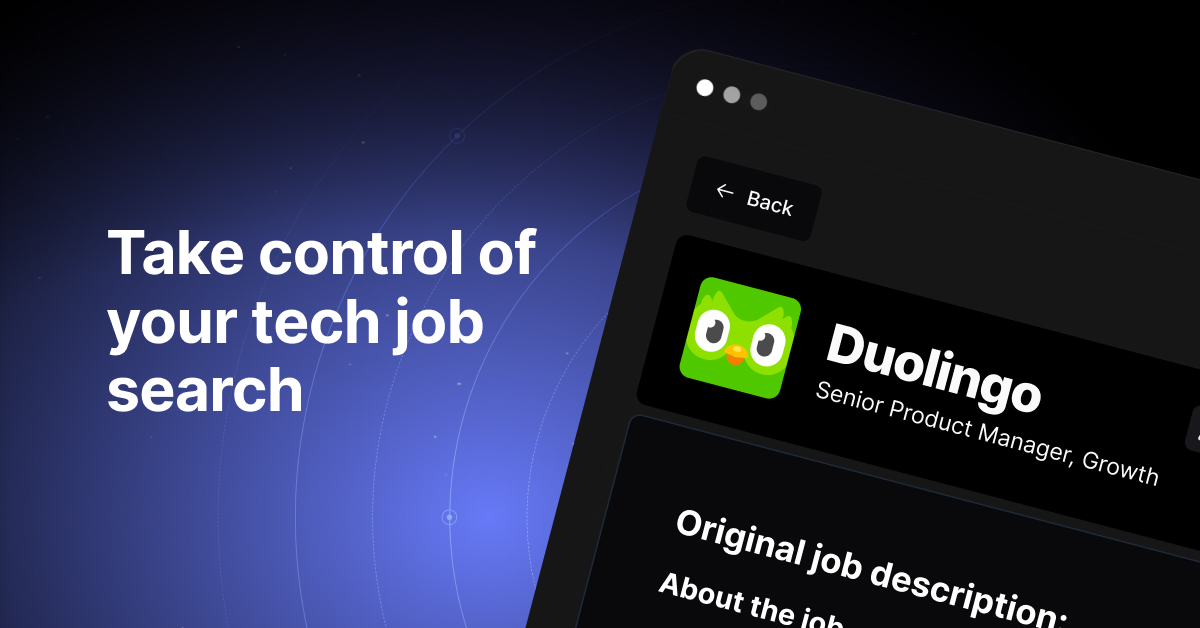