Is there anything like .NET"s NotImplementedException in Java?
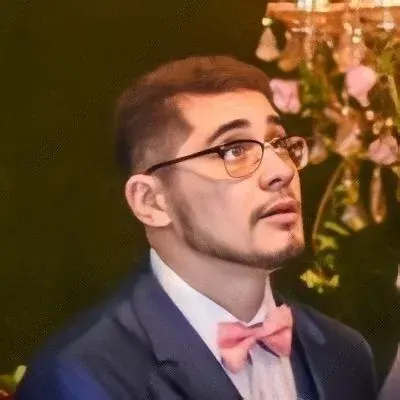
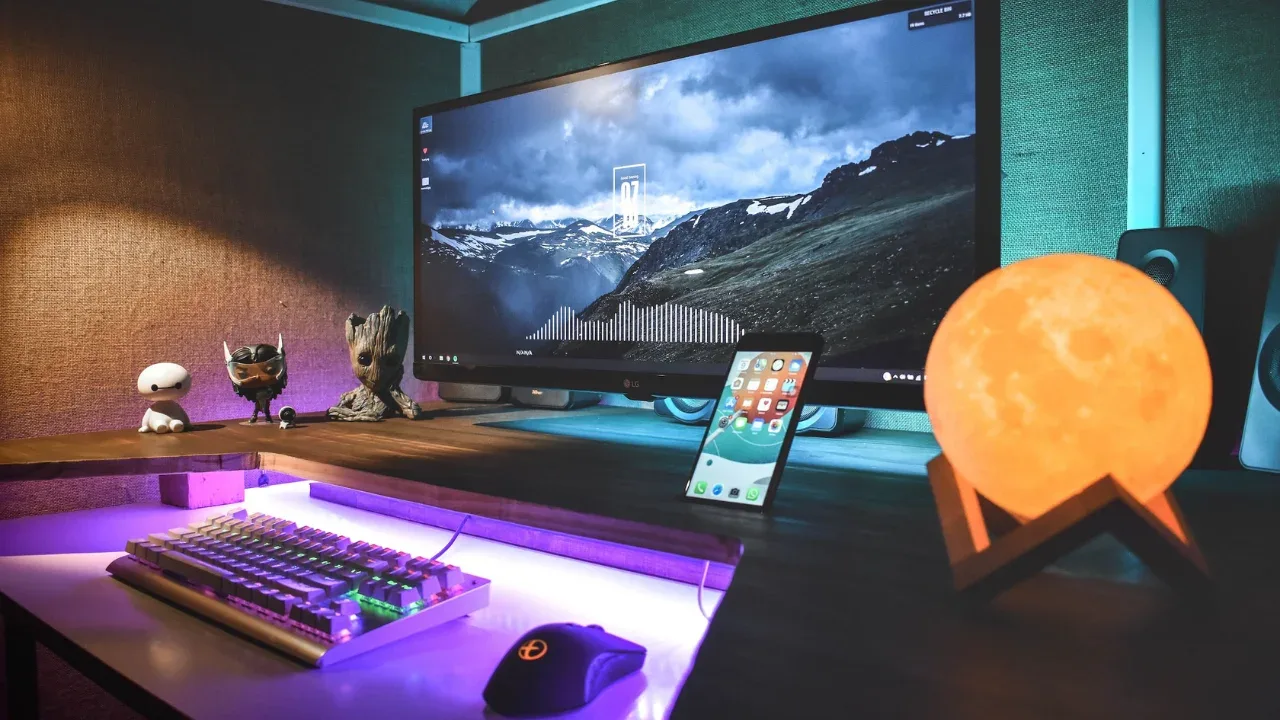
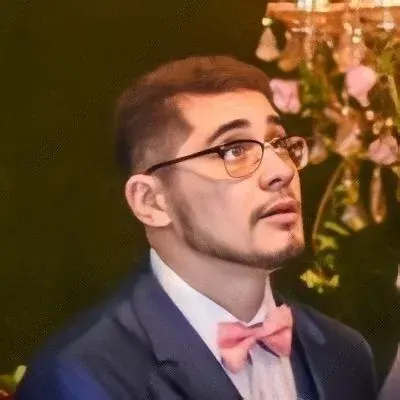
Is there anything like .NET's NotImplementedException in Java? 🤔
So, you've come across the concept of NotImplementedException
in .NET and wondering if there's something similar in Java? Well, you're in luck! While Java doesn't have a built-in NotImplementedException
class like .NET, you can still achieve similar functionality in Java with a few workarounds. Let's dive into this topic and explore some options! 💡
The Why behind NotImplementedException
Before we explore the solutions in Java, let's quickly understand the purpose of NotImplementedException
. In .NET, NotImplementedException
is an exception class that developers can explicitly throw when they encounter a feature or functionality that is not yet implemented. It serves as a way to signal that a particular code block or method needs to be implemented in the future. This helps in identifying incomplete or unfinished code during development or testing phases. 😉
Solution 1: UnsupportedOperationException
In Java, you can leverage the UnsupportedOperationException
class to achieve similar behavior. This exception is typically used to indicate that the requested operation is not supported by the given object or class. You can throw this exception to denote that a particular method or feature is not yet implemented. 🚫🧰
Let's see an example:
// Example class with a not yet implemented method
public class MyClass {
public void myMethod() {
throw new UnsupportedOperationException();
}
}
By throwing UnsupportedOperationException
, you can indicate that myMethod()
is not yet implemented. Developers who encounter this exception will know that they need to implement the method before using it.
Solution 2: Custom Exceptions
If you prefer a more explicit approach, you can create your own custom exception class specifically for situations where code is not implemented. This allows you to provide more context or additional functionality as needed. 📝🚀
Here's an example of a custom exception:
public class NotImplementedException extends RuntimeException {
public NotImplementedException() {
super("This method is not yet implemented.");
}
}
With this custom exception class, you can throw it wherever you need to indicate that a particular method or feature is not yet implemented:
public class MyCustomClass {
public void myMethod() {
throw new NotImplementedException();
}
}
Choose What Works Best for You
Now that you have two different approaches to handle the NotImplementedException
scenario in Java, it's time to choose what best suits your needs. Both solutions effectively convey that certain features are not yet implemented, allowing you to avoid confusion and clearly communicate your intentions as a developer. 🤝
Road to Completion! ✅
Remember, throwing UnsupportedOperationException
or using a custom exception class like NotImplementedException
is not the final destination - it's just a roadmap placeholder! Whenever you use these approaches, make sure to go back and implement the missing code as soon as possible. This way, you can move towards a complete and functional application! 🚀💪
Your Opinion Counts! 📣
What's your take on implementing unfinished code? Do you prefer using UnsupportedOperationException
or creating a custom exception class like NotImplementedException
in Java? Or maybe you have other ideas? Share your thoughts and experiences in the comments below! Let's level up our programming skills together! 🌟💬
Remember, embracing incomplete code is just one step towards creating amazing software. Keep coding, keep exploring, and never stop learning! Happy coding! 👩💻👨💻
[Your Call to Action]
If you found this article helpful, share it with fellow Java developers and spread the knowledge! Let's help others tackle the challenges of implementing unfinished code in Java. Also, don't forget to subscribe to our blog to stay updated with more useful tech guides like this. Together, we can simplify complex programming concepts! 🎉💌