Is null check needed before calling instanceof?
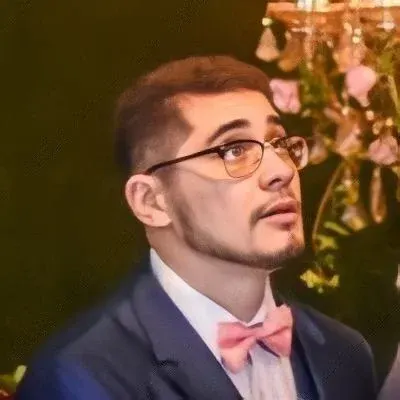
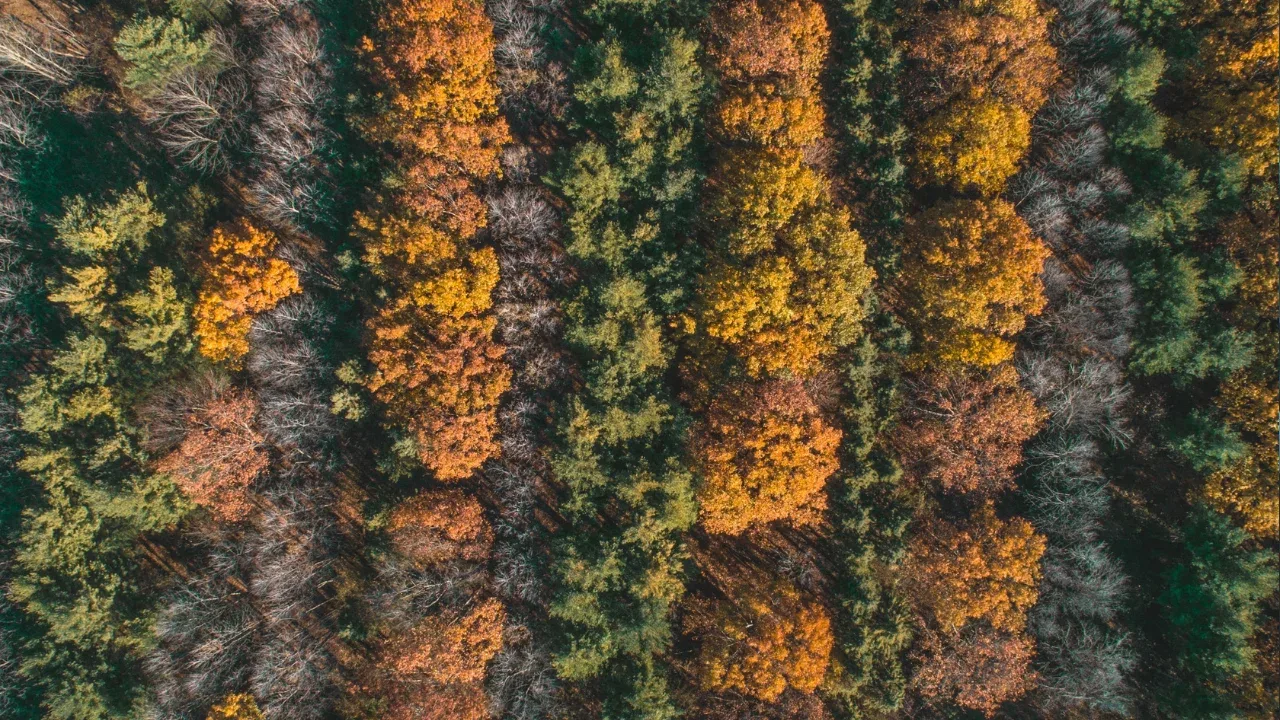
🤔 Is null check needed before calling instanceof
?
Hey tech enthusiasts! 👋 Today, we're diving into a common question that often puzzles developers: Should we perform a null check before calling instanceof
? 🤔 Let's explore this topic and find some easy solutions together! 🚀
The Problem:
Imagine this scenario: You have a code snippet with the expression null instanceof SomeClass
. What would be the result? Will it return false
or throw a NullPointerException
? 🤷♀️
The Explanation:
Surprisingly, null instanceof SomeClass
will always return false
. It will never throw a NullPointerException
. 🙅♂️
Why is that? Well, instanceof
checks if an object is an instance of a particular class or interface. When null
is used with instanceof
, Java understands that null
is not an instance of any class, so it returns false
. 😮
The Easy Solution:
Given that null instanceof SomeClass
always returns false
, there is no need to perform a null check before calling instanceof
. It doesn't change the outcome. ✅
Instead of writing code like this:
if (object != null && object instanceof SomeClass) {
// Some code here
}
You can simply write:
if (object instanceof SomeClass) {
// Some code here
}
The Benefits:
By skipping the unnecessary null check, your code becomes more concise and easier to read. You can get rid of those redundant lines, making your logic more straightforward. Plus, fewer lines of code means less potential for bugs to creep in! 💪
The Call-to-Action:
Now that you know that a null check is not needed before calling instanceof
, go ahead and refactor that extra code. You'll save time and improve the quality of your code. 🎉
If you found this blog post helpful, don't forget to share it with your fellow developers. And feel free to leave your thoughts and questions in the comments section below. Let's learn and grow together! 🌱💡
Keep coding! 💻
References:
Official Java documentation on The instanceof Operator
(Disclaimer: This blog post assumes knowledge of Java programming language and its basic constructs. If you're new to Java, start by going through some introductory Java resources.)
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
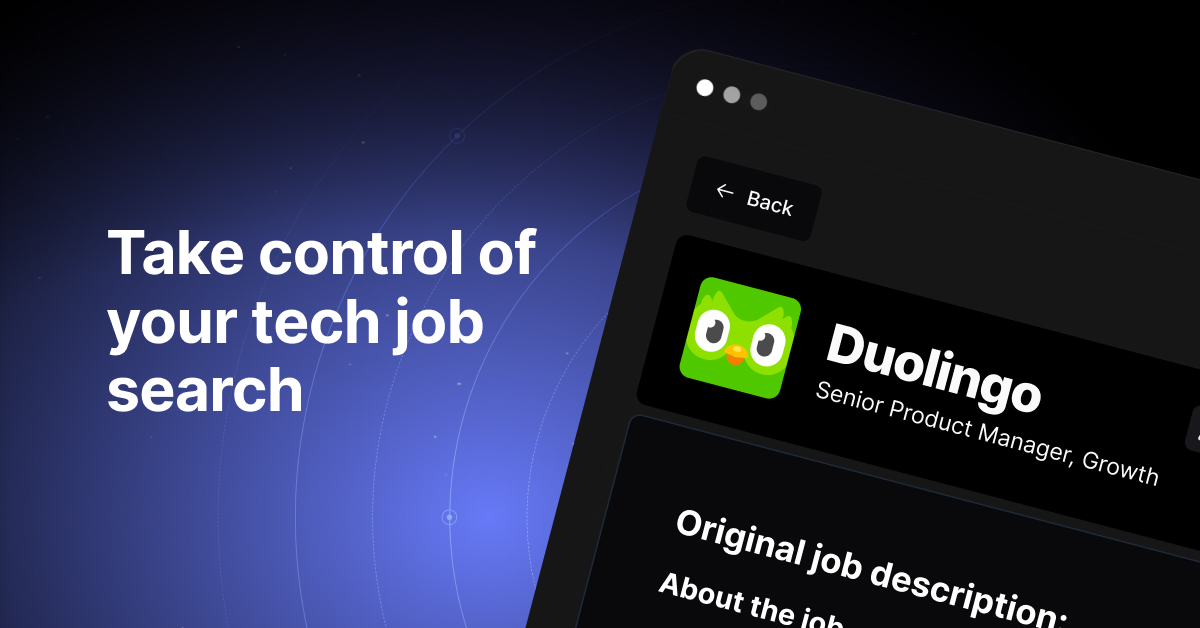