Is it possible to have empty RequestParam values use the defaultValue?
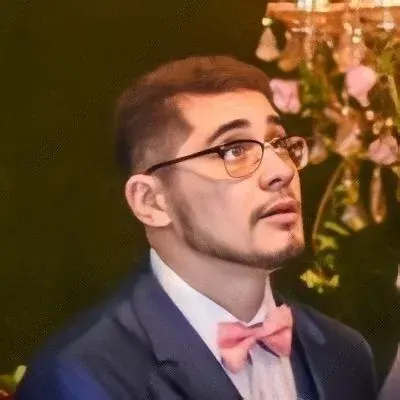
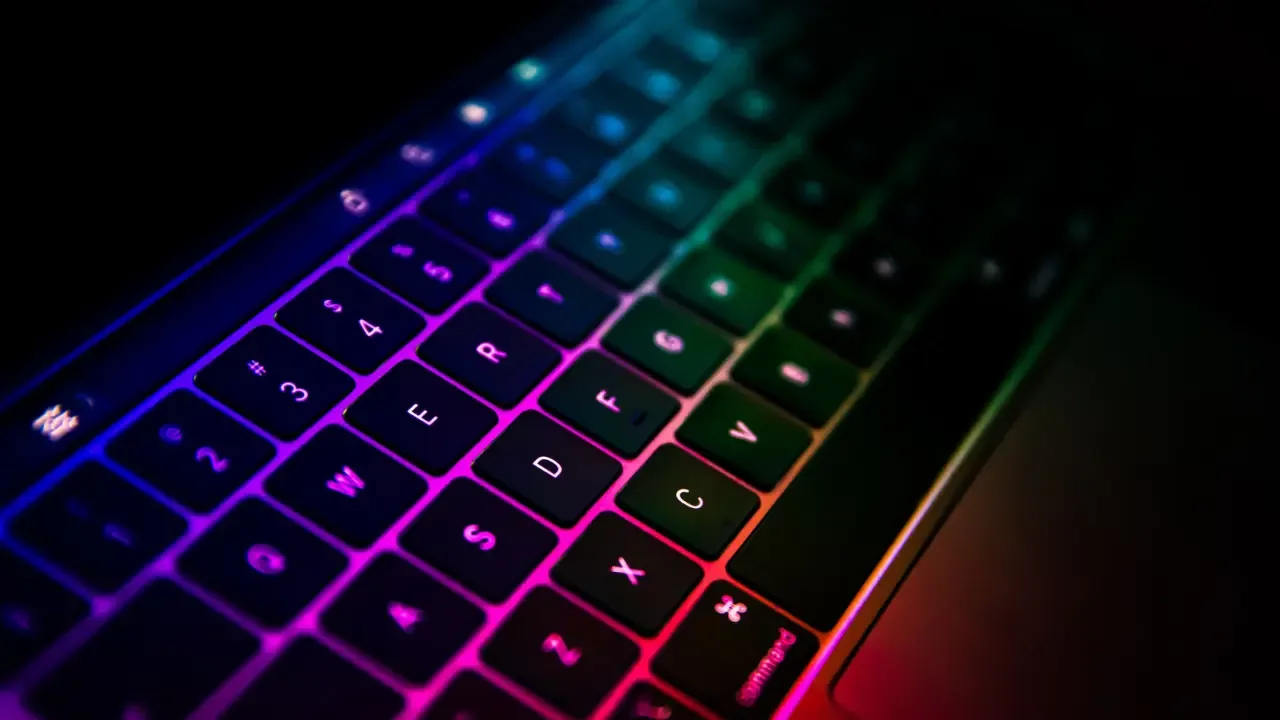
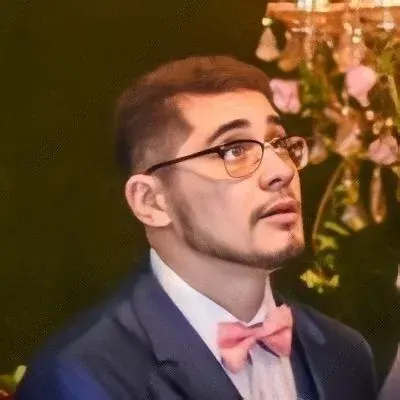
📝 Empty RequestParam values and defaultValue in Spring MVC
Are you encountering an error when trying to handle empty request parameter values in your Spring MVC application? 🤔
Imagine you have a request mapping similar to the following:
@RequestMapping(value = "/test", method = RequestMethod.POST)
@ResponseBody
public void test(@RequestParam(value = "i", defaultValue = "10") int i) {
}
And then you call this request with:
http://example.com/test?i=
You might encounter the following error message:
Failed to convert value of type 'java.lang.String' to type 'int'; nested exception is java.lang.NumberFormatException: For input string: ""
The Problem 😫
The reason for this error is that Spring tries to convert the empty string value to an integer directly and fails in the process. It expects a valid integer value, but an empty string doesn't fit the bill. 😕
The Solution 💡
Luckily, there are a couple of easy solutions to handle this issue:
Solution 1: Stop the Client from Sending Empty Parameters
One solution is to ensure that the JavaScript client stops sending empty parameters in the first place. By validating the input before sending the request, you can prevent this error from occurring altogether. ✋
Solution 2: Accept String Values and Check for Blankness
Another solution involves accepting string values as request parameters and parsing them only if they are not blank. This way, you can explicitly handle the empty or null values and set the default value manually. 👌
Here's an example of how you can modify your request mapping to accommodate this solution:
@RequestMapping(value = "/test", method = RequestMethod.POST)
@ResponseBody
public void test(@RequestParam(value = "i") String i) {
int parsedValue = StringUtils.isBlank(i) ? 10 : Integer.parseInt(i);
// your logic here
}
In this example, we are using the StringUtils.isBlank()
method from Apache Commons Lang to check if the value is blank (i.e., empty or null). If it is, we set a default value of 10. Otherwise, we parse the string value as an integer.
Update 🔄
Please note that in later versions of Spring (from 4.3.5 onwards), the framework implemented the originally desired behavior. This means that the null value will be automatically converted to the default value, without triggering a NumberFormatException
. So, if you are using a newer version of Spring, the original mapping you shared should work fine without modifications. 😄
Unfortunately, the exact version in which this behavioral change was made remains unknown. However, it's always a good idea to keep your dependencies up to date and consult the Spring documentation for more information.
Conclusion 🎉
Handling empty request parameter values in Spring MVC can lead to errors if not properly handled. By using one of the solutions presented above, you can overcome this issue and ensure smooth functioning of your application. Remember to validate input on the client-side and handle empty or null values on the server-side, using string values as necessary.
Have you encountered this issue before and found a different solution? Or do you have any other questions regarding Spring MVC? Let us know in the comments below! We'd love to hear from you and help you out. 👇