Infinite Recursion with Jackson JSON and Hibernate JPA issue
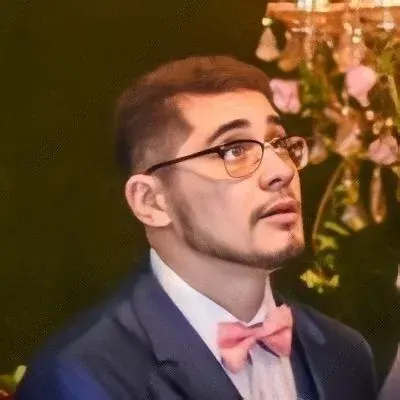
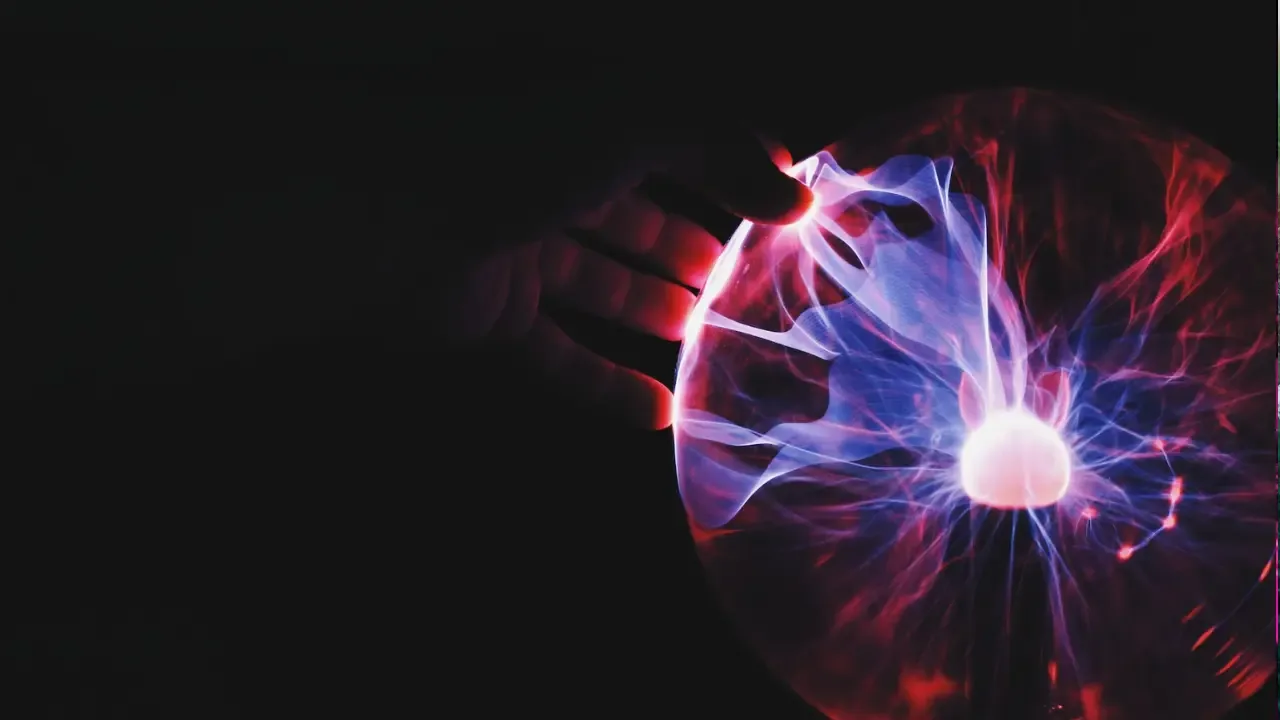
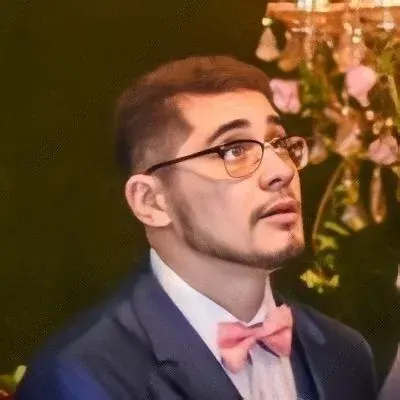
Infinite Recursion with Jackson JSON and Hibernate JPA issue: A Simple Guide 🔄
Have you ever encountered the dreaded "Infinite recursion" error when converting a JPA object with bi-directional associations into JSON using Jackson JSON and Hibernate JPA? 😱 If you've been scratching your head trying to find a solution, look no further. In this blog post, we will unravel this issue, provide easy-to-implement solutions, and empower you to overcome this challenge once and for all! 🎉
The Problem 🤔
So, what is this "Infinite recursion" error all about? 🔄 Well, let's say you have two business objects: Trainee
and BodyStat
. Trainee
has a set of BodyStat
objects, and BodyStat
has a reference back to the parent Trainee
. When you try to convert a Trainee
object into JSON using Jackson JSON and Hibernate JPA, you may encounter the following error: "org.codehaus.jackson.map.JsonMappingException: Infinite recursion (StackOverflowError)". 😭
The Solution 💡
Fear not, for there is a light at the end of the tunnel! Here are three easy solutions to tackle the "Infinite recursion" issue:
1. Use @JsonIgnore
annotation 🙅♀️
One way to break the recursion cycle is by using the @JsonIgnore
annotation provided by the Jackson library. Apply this annotation to the bi-directional relationship in your JPA entity classes. In our case, you would add @JsonIgnore
to the trainee
field in the BodyStat
class.
Here's an example of how it would look in your code:
@ManyToOne(fetch = FetchType.EAGER, cascade = CascadeType.ALL)
@JoinColumn(name="trainee_fk")
@JsonIgnore
private Trainee trainee;
By ignoring the trainee
field during JSON serialization, you effectively break the recursive loop and prevent the "Infinite recursion" error. 🎯
2. Use DTOs (Data Transfer Objects) 👩💼
Another approach is to use Data Transfer Objects (DTOs) to decouple your JPA entities from your JSON representation. Create separate DTOs for Trainee
and BodyStat
that contain only the necessary fields for JSON serialization. Then, map your JPA entities to these DTOs before converting them to JSON.
For example, you could create a TraineeDto
and a BodyStatDto
class with only the required fields:
public class TraineeDto {
private String name;
private String surname;
// Getters and setters
}
public class BodyStatDto {
private Float height;
private Date measureTime;
// Getters and setters
}
In your controller, you would map your JPA entities to DTOs before returning them as JSON:
@RequestMapping(value = "/getAllTrainees", method = RequestMethod.GET)
@ResponseBody
public Collection<TraineeDto> getAllTrainees() {
Collection<Trainee> allTrainees = this.traineeDAO.getAll();
List<TraineeDto> traineeDtos = allTrainees.stream()
.map(trainee -> {
TraineeDto traineeDto = new TraineeDto();
traineeDto.setName(trainee.getName());
traineeDto.setSurname(trainee.getSurname());
return traineeDto;
})
.collect(Collectors.toList());
return traineeDtos;
}
Using DTOs allows you to control the JSON serialization process explicitly and avoid any unwanted recursion issues. 🧰
3. Customize Jackson's ObjectMapper 🖌️
The third solution involves customizing Jackson's ObjectMapper
to handle the recursion issue automatically. By configuring the ObjectMapper
to handle bi-directional associations more gracefully, you can avoid the "Infinite recursion" error.
Here's an example of how you can customize the ObjectMapper
:
@Configuration
public class JacksonConfig {
@Bean
public ObjectMapper objectMapper() {
ObjectMapper objectMapper = new ObjectMapper();
// Configure your ObjectMapper here
objectMapper.configure(DeserializationFeature.FAIL_ON_IGNORED_PROPERTIES, false);
objectMapper.configure(SerializationFeature.FAIL_ON_EMPTY_BEANS, false);
objectMapper.configure(SerializationFeature.WRITE_NULL_MAP_VALUES, false);
objectMapper.configure(SerializationFeature.WRITE_EMPTY_JSON_ARRAYS, false);
// Add more customization if needed
return objectMapper;
}
}
By providing custom configuration to the ObjectMapper
, you can fine-tune its behavior and handle bi-directional associations without running into the "Infinite recursion" problem. 🎨
Conclusion 🎓
In summary, dealing with the "Infinite recursion" issue when using Jackson JSON and Hibernate JPA is no longer an insurmountable challenge. With the solutions we've discussed – using @JsonIgnore
annotation, employing DTOs, or customizing Jackson's ObjectMapper
– you can overcome this problem and continue converting your JPA objects into JSON effortlessly.
Have you encountered this issue before? How did you solve it? Let us know in the comments below! 💬 And don't forget to share this post with your fellow developers who might be struggling with the "Infinite recursion" problem. Together, we can conquer any code-related hurdles! 🚀