In Java, how do I parse XML as a String instead of a file?
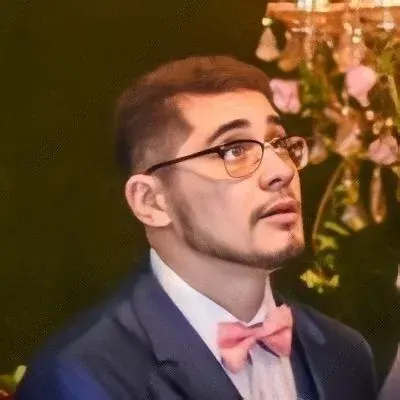
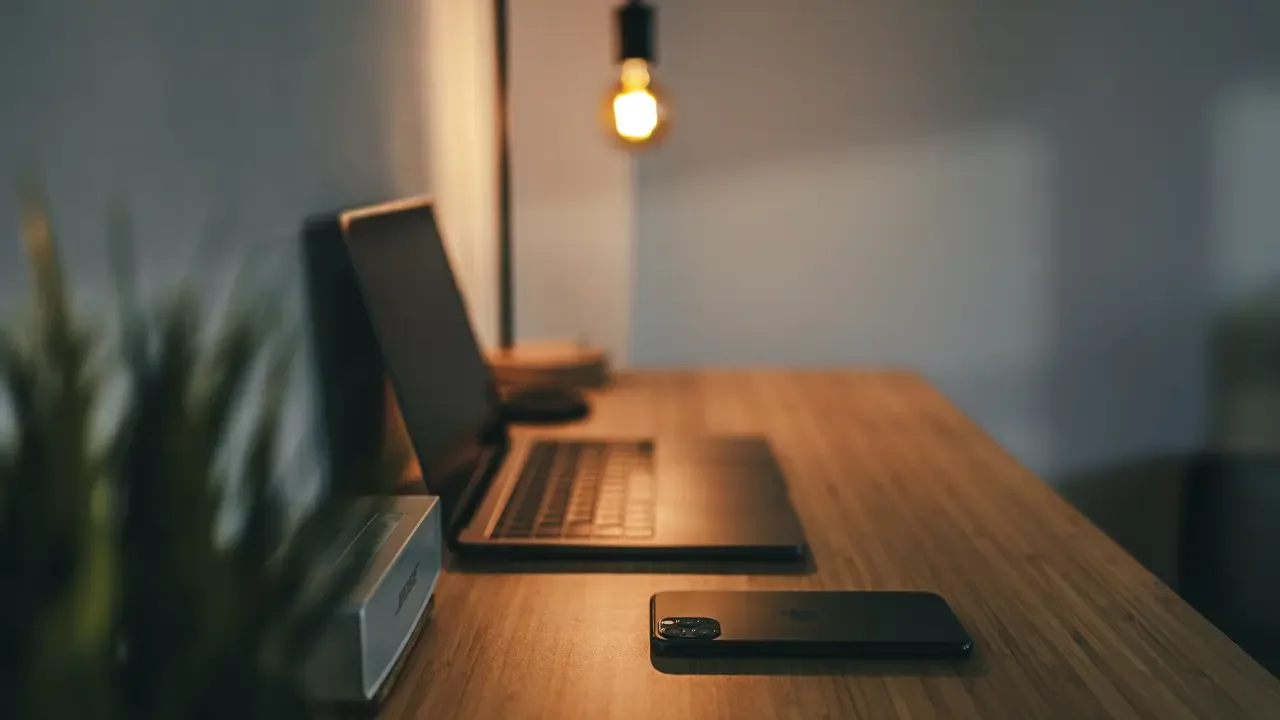
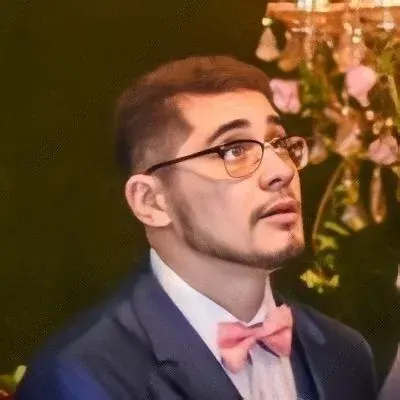
How to Parse XML as a String in Java 📝
Are you tired of dealing with XML files in Java and wish there was an easier way to work with XML data? Well, you're in luck! In this guide, we'll show you how to parse XML as a String instead of a file, so you can kiss those file reading headaches goodbye. 😌
The Problem 🤔
Let's start by addressing the specific problem at hand. You have the following code snippet:
DocumentBuilderFactory.newInstance().newDocumentBuilder().parse(xmlFile);
The parse()
method expects an InputStream
or a File
as its parameter, but you want to parse XML data that is stored within a String variable. So, how can you achieve this?
The Solution 💡
Fortunately, Java provides a way to parse XML from a String using a StringReader
and an InputSource
. Here's how you can modify your code to parse XML as a String:
String xmlString = "<root><element>Some XML data</element></root>";
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
InputSource inputSource = new InputSource(new StringReader(xmlString));
Document xmlDoc = builder.parse(inputSource);
In the above code snippet, we create a StringReader
to read the XML data stored in the xmlString
variable. Then, we create an InputSource
using the StringReader
. Finally, the parse()
method is called with the InputSource
to obtain the parsed XML document.
A Simple Example 🌟
Let's dive deeper into an example to solidify the understanding.
import org.w3c.dom.Document;
import org.xml.sax.InputSource;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import java.io.StringReader;
public class XMLParser {
public static void main(String[] args) {
String xmlString = "<root><element>Some XML data</element></root>";
try {
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
InputSource inputSource = new InputSource(new StringReader(xmlString));
Document xmlDoc = builder.parse(inputSource);
// Process the parsed XML document here
// For example, you can traverse the document, extract nodes, etc.
} catch (Exception e) {
e.printStackTrace();
}
}
}
Simply replace the xmlString
with your desired XML, and you're good to go!
Keep Parsing XML Without a File 🚀
Now that you know how to parse XML as a String in Java, you can handle XML data with ease! No more struggling with file reading and unnecessary complexities. 😁
Feel free to experiment with different XML data and build powerful applications that process XML efficiently.
If you found this guide helpful, share it with your fellow developers and spread the knowledge! If you have any questions or suggestions, leave a comment below.
Happy XML parsing! 🎉