Ignoring new fields on JSON objects using Jackson
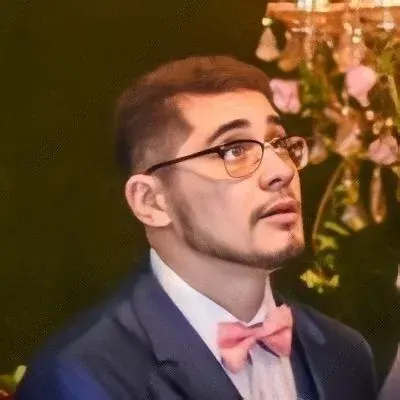
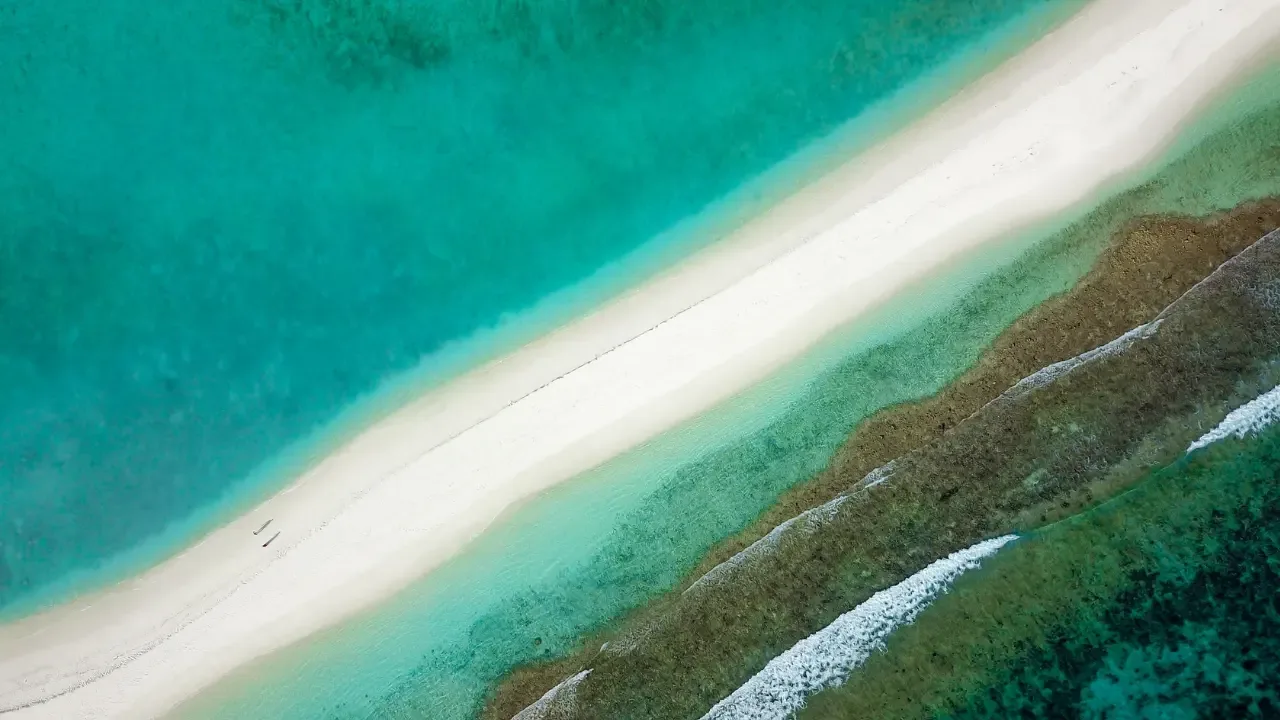
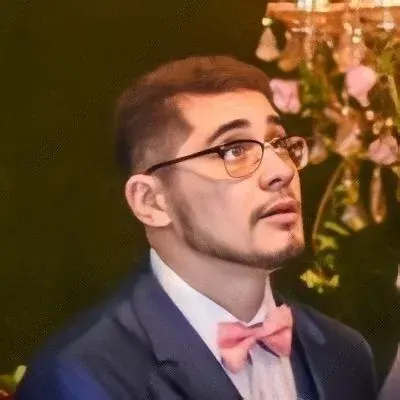
Ignoring new fields on JSON objects using Jackson 😎🔥
So, you're using the Jackson JSON library to convert JSON objects to POJO classes in your Android application. But here's the catch: the JSON objects might change and have new fields added while your application is published, leading to potential breaking changes. 😱
But fear not! We've got a solution for you. In this blog post, we will address this common issue and provide you with easy solutions to ignore newly added fields using Jackson. Let's dive right in! 💪
The problem at hand 🤔
Imagine this scenario: you have a JSON object with a set structure, and your goal is to convert it into a corresponding Java object using Jackson. However, the JSON object could evolve over time, with new fields being added. And when that happens, your code crashes because it doesn't recognize these new fields. Talk about a headache! 😫
So, how can we tell Jackson to gracefully ignore these new fields? Let's find out! 🕵️♀️
Solution 1: Using @JsonIgnoreProperties(ignoreUnknown = true)
🙅♂️
One simple solution is to utilize the @JsonIgnoreProperties
annotation provided by Jackson. By adding this annotation on your POJO class, you can tell Jackson to ignore any unknown properties found in the JSON object.
Here's how you can use it:
@JsonIgnoreProperties(ignoreUnknown = true)
public class MyPojo {
// Your class properties...
}
With this annotation, Jackson will quietly skip any unknown fields found in the JSON object without causing your code to break. 🎉
Solution 2: Configuring ObjectMapper
at the global level 🌍
If you want to globally ignore unknown properties for every mapping, you can configure your ObjectMapper
to do so. This means you won't have to add the @JsonIgnoreProperties
annotation to every POJO class individually.
Here's how you can achieve this:
ObjectMapper objectMapper = new ObjectMapper();
objectMapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
By calling configure()
on your ObjectMapper
instance and passing DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES
as the feature to configure with false
, you're effectively telling Jackson to ignore unknown properties on a global level.
A compelling call-to-action! 📢
There you have it, folks! Two simple solutions to ignore newly added fields on JSON objects using Jackson. Say goodbye to breaking changes and hello to smooth sailing! 🌊⛵️
Now it's your turn to take action. Implement these solutions in your code and save yourself from potential headaches caused by changing JSON objects.
Have you ever encountered issues with unknown properties in JSON objects? How did you handle them? Share your experiences and thoughts in the comments below! Let's learn from each other and make our coding lives easier. 👇
Happy coding! 💻🚀