How to verify that a specific method was not called using Mockito?
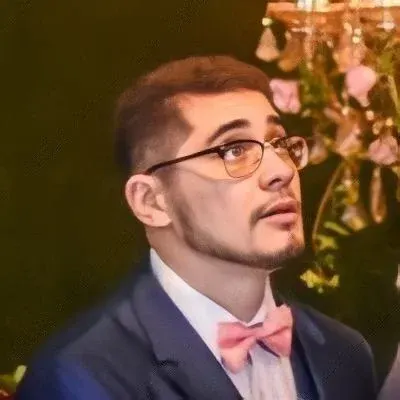
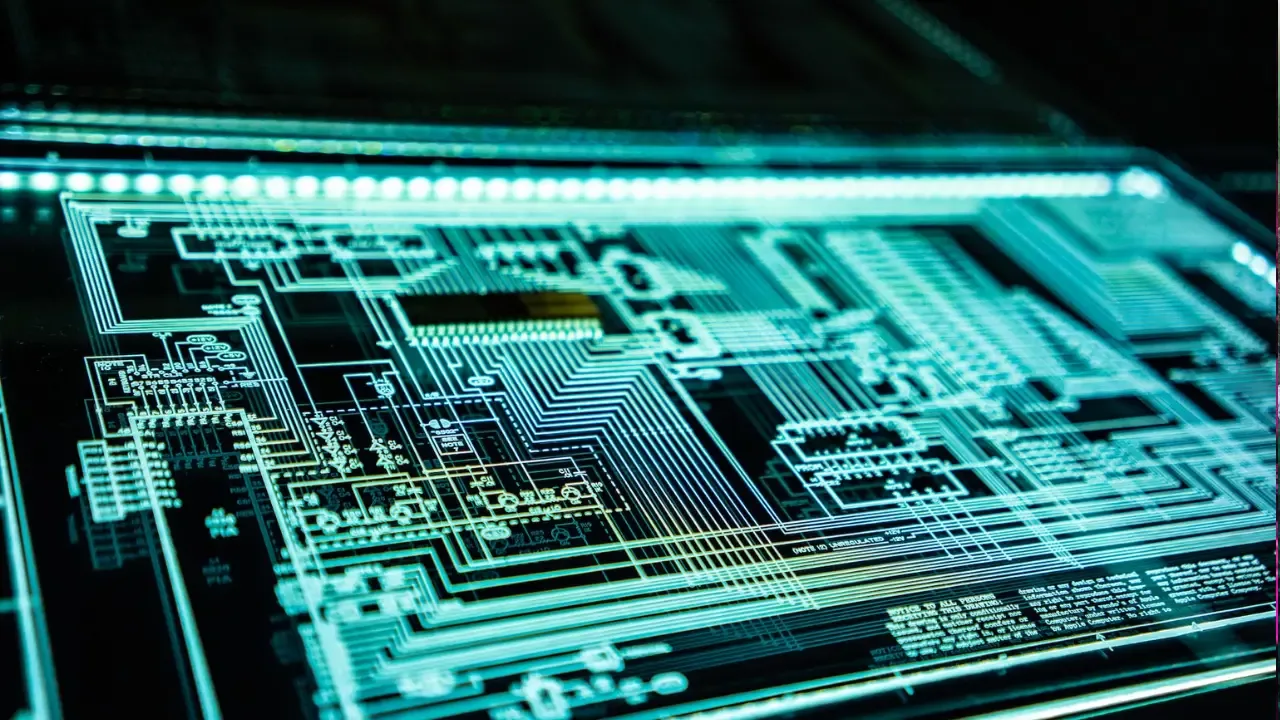
š Title: How to Verify that a Specific Method was not Called using Mockito
š Hey there, tech enthusiasts! Welcome back to our blog. Today, we have an interesting topic to discuss - how to verify that a specific method was not called using Mockito. š
So, you're working on a project and you've stumbled upon a scenario where you need to make sure that a particular method is not called on an object's dependency. Let's dive into the details and understand how to tackle this situation effortlessly. šŖ
š Understanding the Problem:
To understand the problem better, let's consider a sample implementation. We have an interface called Dependency
with a method named someMethod()
. We also have a class called Foo
, which accepts an instance of Dependency
as a parameter in its method bar()
. Our goal is to verify that the someMethod()
of Dependency
is not called when executing the bar()
method of Foo
.
š Solution:
To achieve this verification, we can utilize the power of Mockito, a popular testing framework that provides a simple and elegant way to mock objects and verify their behavior. Mockito offers a variety of methods to verify method calls, but we need one that verifies the absence of a specific method call. Let's see how it's done:
Mock the Dependency:
First, we need to create a mock instance of the Dependency
interface. In our test, we can achieve this by using the mock()
static method provided by the Mockito framework. This will create a mock object of the Dependency
interface, allowing us to control its behavior and verify method calls.
final Dependency dependency = mock(Dependency.class);
Invoke the Method under Test:
Next, we need to execute the method that we want to test. In our case, this is the bar()
method of the Foo
class, passing the mocked Dependency
object as a parameter.
foo.bar(dependency);
Verify the Absence of Method Call:
Finally, we can use the verifyNoInteractions()
method of Mockito to ensure that the someMethod()
of the Dependency
object was not called during the execution of our test. This method verifies that there were no interactions with the specified mock object.
verifyNoInteractions(dependency);
And that's it! You have successfully verified that the someMethod()
of the Dependency
object was not called. š
š Example Test:
To give you a complete picture of how this verification works, let's take a look at an example test using the sample code provided earlier.
public class FooTest {
@Test
public void dependencyIsNotCalled() {
// Arrange
final Foo foo = new Foo(...);
final Dependency dependency = mock(Dependency.class);
// Act
foo.bar(dependency);
// Assert
verifyNoInteractions(dependency);
}
}
In this test, we create an instance of Foo
and a mock Dependency
object. We then invoke the bar()
method of Foo
, passing the mock Dependency
object. Finally, we verify that there were no interactions with the Dependency
mock using verifyNoInteractions(dependency)
.
š£ Call to Action:
Congratulations! You've learned a neat trick to verify that a specific method was not called using Mockito. This knowledge will come handy in your future tests, ensuring the correctness of your code.
Now, it's your turn to put this technique into practice. Try it out in your own tests and let us know your experience. Did it help you uncover unexpected interactions? Share your feedback and thoughts in the comments section below. We'd love to hear from you! š¤
Remember, sharing is caring! If you found this article helpful, don't forget to hit the share button and spread the word. Until next time, happy testing! āļø
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
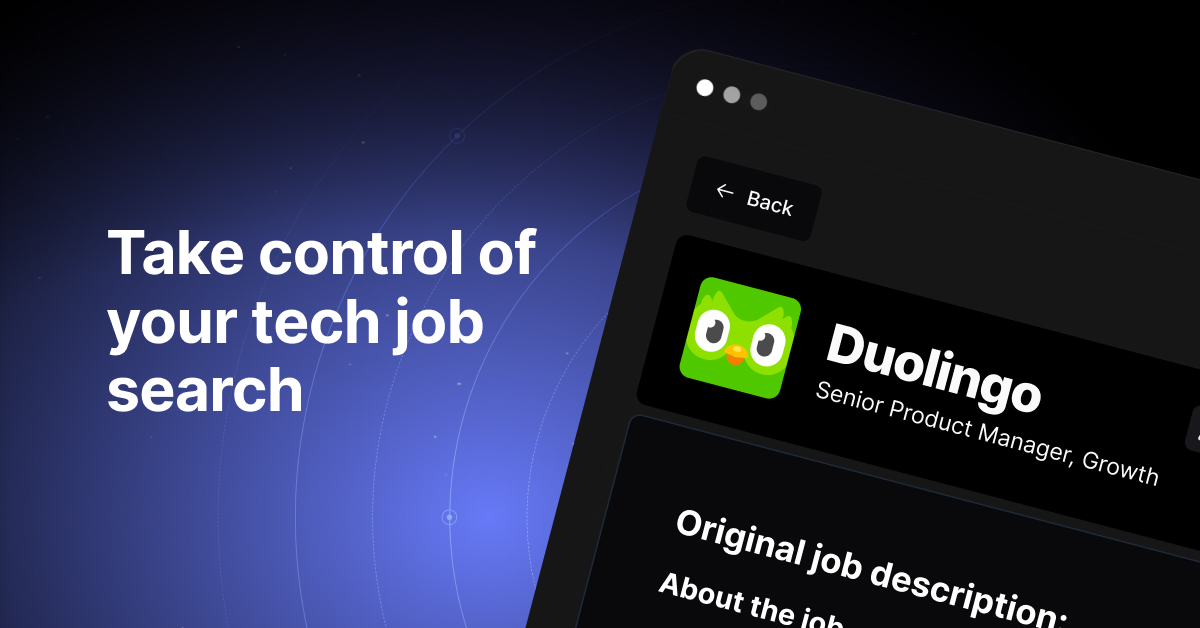