How to use JNDI DataSource provided by Tomcat in Spring?
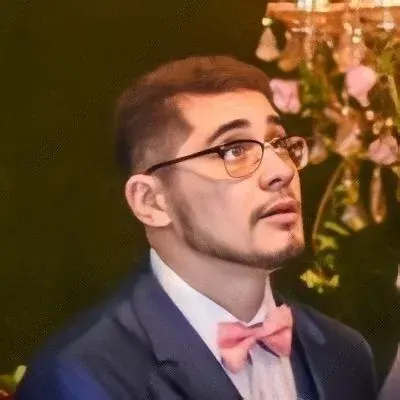
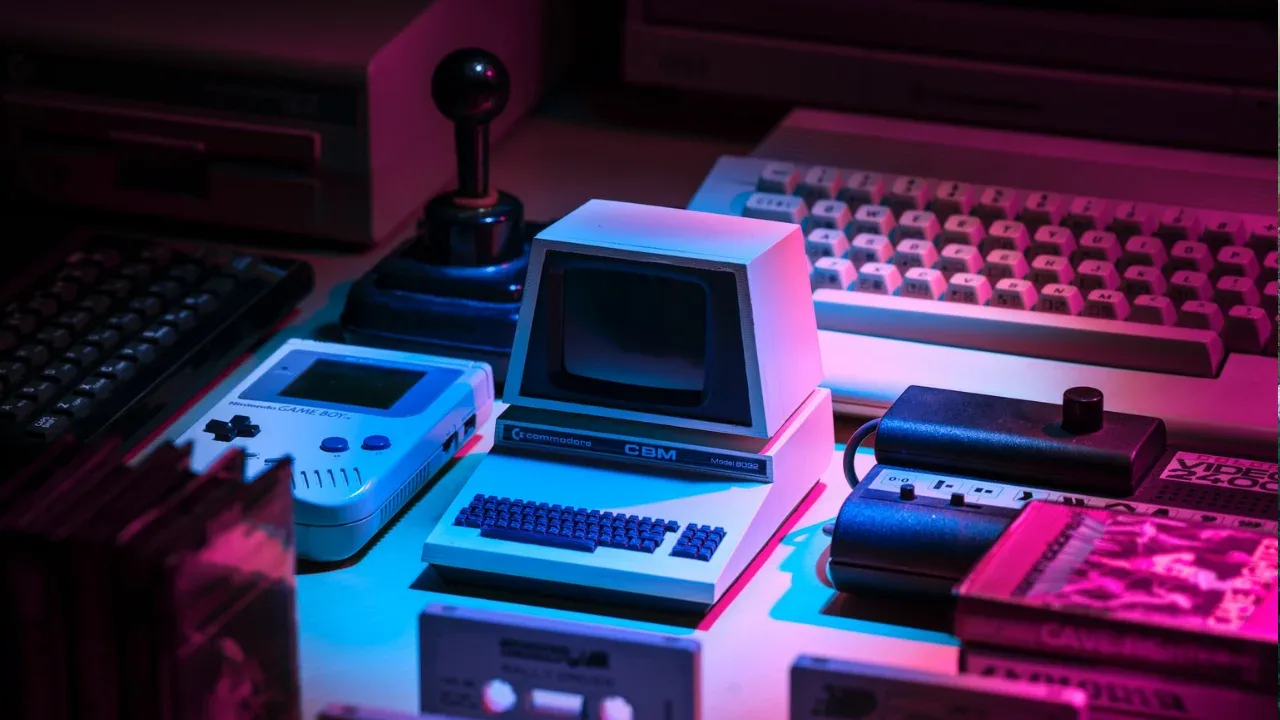
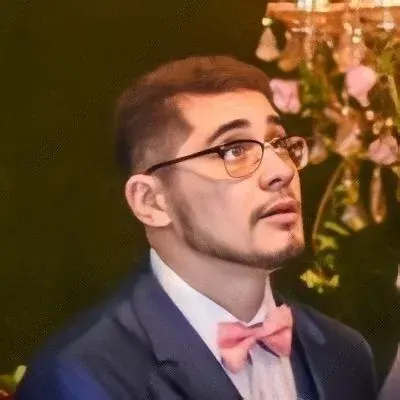
How to Use JNDI DataSource Provided by Tomcat in Spring 👩💻🚀
Have you ever wondered how to efficiently utilize a JNDI DataSource provided by Tomcat in Spring? Look no further! We're here to demystify this process and provide you with easy-to-follow steps and solutions. Let's dive in! 💦
The Background Story 📚
In the Spring javadoc article about the DriverManagerDataSource
class, it's mentioned that using a JNDI DataSource provided by the container is highly recommended. This not only simplifies your code but also ensures a more efficient connection to your database. Great, right? But how do we accomplish this? 🤔
The Burning Question 🔥
So, you may be asking yourself, "How can I create a DataSource
bean to access my custom MySQL database using the provided JNDI DataSource in Tomcat?" Well, fret not! We've got you covered with an easy-to-understand solution. 🙌
Step 1: Configure Tomcat's server.xml 🛠️
First, we need to set up the JNDI resource in Tomcat's server.xml
configuration file. Open up the file, and locate the <GlobalNamingResources>
element. Here's an example:
<GlobalNamingResources>
<!-- Other resources -->
<Resource name="jdbc/myCustomDataSource"
auth="Container"
type="javax.sql.DataSource"
driverClassName="com.mysql.jdbc.Driver"
url="jdbc:mysql://localhost:3306/my_database"
username="your_username"
password="your_password"/>
</GlobalNamingResources>
In this example, we've defined a resource with the name jdbc/myCustomDataSource
. Feel free to change the name according to your preference. ✏️
Step 2: Update context.xml ☑️
Next, navigate to your Tomcat's web application's context.xml
file and add the following:
<ResourceLink name="jdbc/myCustomDataSource"
global="jdbc/myCustomDataSource"
type="javax.sql.DataSource" />
By linking the resource in the context.xml
, we make it accessible to our Spring application. 🌐
Step 3: Spring Application Configuration 🌸
Now we move on to our Spring application's configuration. Create or open your Spring application's context configuration file (often applicationContext.xml
), and add the following:
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd">
<!-- Other configuration beans -->
<bean id="myCustomDataSource" class="org.springframework.jndi.JndiObjectFactoryBean">
<property name="jndiName" value="java:comp/env/jdbc/myCustomDataSource" />
</bean>
<bean id="myCustomJdbcTemplate" class="org.springframework.jdbc.core.JdbcTemplate">
<constructor-arg ref="myCustomDataSource" />
</bean>
<!-- Other beans utilizing the JdbcTemplate -->
</beans>
In this configuration, we define the myCustomDataSource
bean using JndiObjectFactoryBean
, providing the JNDI name we linked in the previous step. We also configure a myCustomJdbcTemplate
bean to utilize the myCustomDataSource
for database operations, such as executing queries. 🗃️
🌟 Test the Magic! 🌟
You're all set! 🎉 Now, you can freely utilize your custom MySQL database just by autowiring the myCustomJdbcTemplate
bean into your services:
@Service
public class MyCustomService {
private final JdbcTemplate jdbcTemplate;
@Autowired
public MyCustomService(JdbcTemplate jdbcTemplate) {
this.jdbcTemplate = jdbcTemplate;
}
// ... Rest of your service methods ...
}
No need to worry about establishing database connections manually! Thanks to the JNDI DataSource provided by Tomcat, Spring will handle everything seamlessly behind the scenes. 🪄
🙌 Your Turn! 🗣️
Now that you've learned how to utilize a JNDI DataSource provided by Tomcat in Spring, it's time for you to put this knowledge into practice. Share your experiences, ask questions, or let us know if you faced any obstacles while following these steps. We'd love to hear from you! 😊💬
✨ Keep calm and code with Spring! ✨