How to use java.net.URLConnection to fire and handle HTTP requests
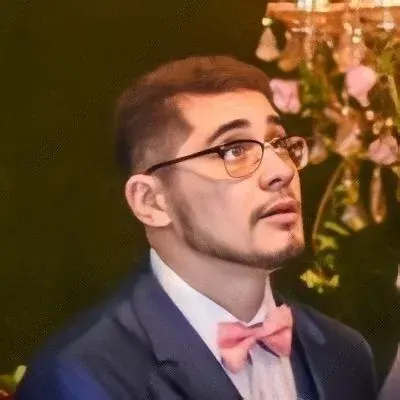
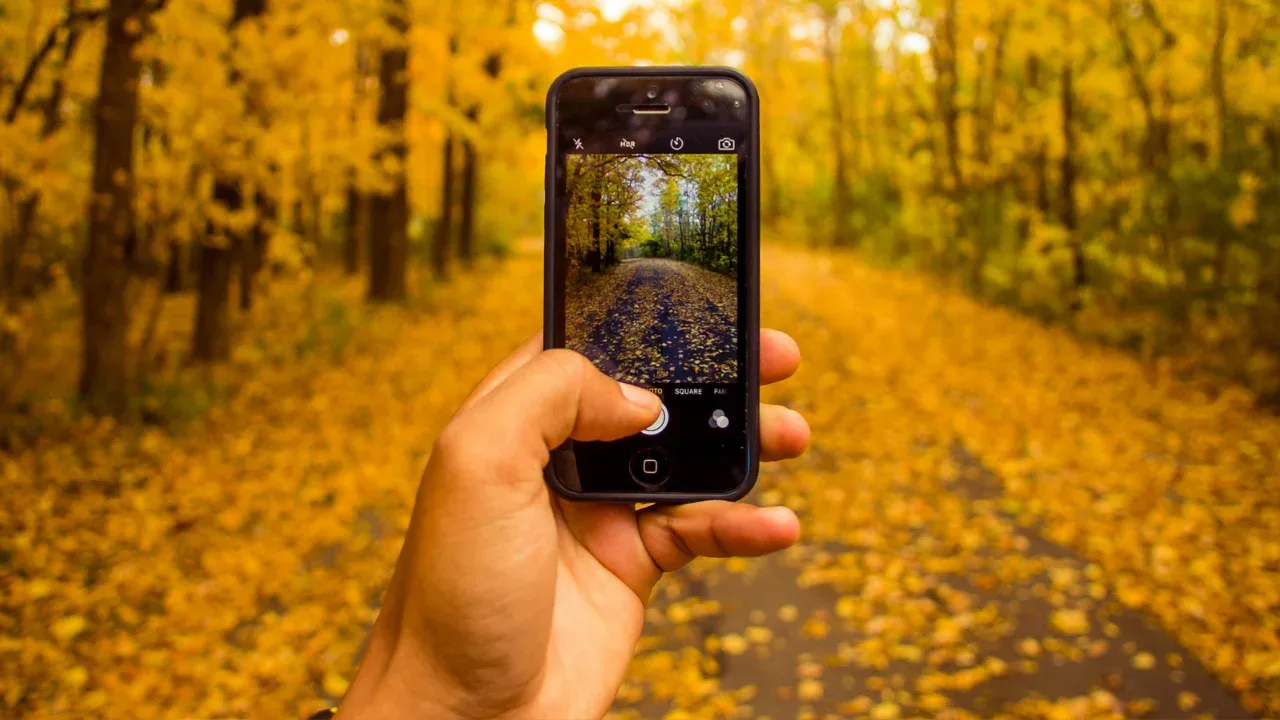
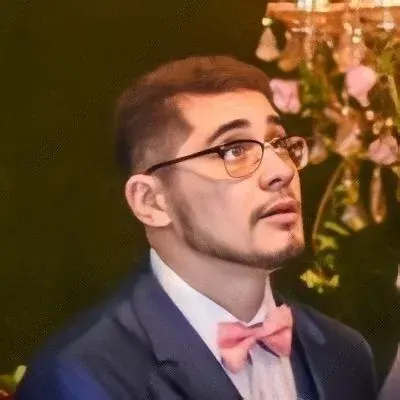
π₯ππ‘ Mastering Advanced HTTP Requests with java.net.URLConnection π
Are you tired of feeling stuck when trying to fire and handle "advanced" HTTP requests using java.net.URLConnection? Don't worry, because I've got you covered! πͺ
π The Problem Explained: When delving into the documentation for java.net.URLConnection, it becomes clear that the provided tutorial is not enough to fully unleash its potential. It only scratches the surface by demonstrating how to fire a simple GET request and read the response. For tasks like POST requests, setting request headers, working with cookies, form submissions, and file uploads, we're left wondering where to turn next. π
π‘ The Easy Solutions: 1οΈβ£ Performing POST Requests: To perform a POST request, you need to do a few extra steps compared to a GET request. Here's a simplified example:
URL url = new URL("https://example.com");
URLConnection connection = url.openConnection();
connection.setDoOutput(true);
connection.setRequestMethod("POST");
OutputStreamWriter out = new OutputStreamWriter(connection.getOutputStream());
out.write("your_post_data_here");
out.close();
// Now, handle the response as usual
2οΈβ£ Setting Request Headers: Want to customize the headers of your HTTP request? We can do that too! Here's how you can add a custom header:
URLConnection connection = new URL("https://example.com").openConnection();
connection.setRequestProperty("Header-Name", "Header-Value");
// Continue with firing the request
3οΈβ£ Reading Response Headers:
To retrieve the response headers, you can use the getHeaderField()
method. Here's a quick example of how to get the value of the "Content-Type" response header:
URLConnection connection = new URL("https://example.com").openConnection();
String contentType = connection.getHeaderField("Content-Type");
// Use the retrieved header value as needed
4οΈβ£ Dealing with Cookies: Handling cookies is essential in web development. With java.net.URLConnection, you can both send and receive cookies. Check out this simplified illustration:
URLConnection connection = new URL("https://example.com").openConnection();
connection.setDoOutput(true);
// Set the cookie value to be sent
connection.setRequestProperty("Cookie", "your_cookie_value");
// Continue with the request handling
5οΈβ£ Submitting an HTML Form: To submit an HTML form using java.net.URLConnection, you need to encode the form data and send it as the request body. Here's a snippet to get you started:
URLConnection connection = new URL("https://example.com").openConnection();
connection.setDoOutput(true);
connection.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
String formData = "name1=value1&name2=value2";
OutputStreamWriter out = new OutputStreamWriter(connection.getOutputStream());
out.write(formData);
out.close();
// Deal with the response as required
6οΈβ£ Uploading a File: If you need to upload a file, java.net.URLConnection has got your back! Here's an example of how to achieve that:
URL url = new URL("https://example.com/upload");
URLConnection connection = url.openConnection();
connection.setDoOutput(true);
connection.setRequestProperty("Content-Type", "multipart/form-data; boundary=YOUR_BOUNDARY");
// Write the file data to the request body as needed
// Handle the response as per your requirements
Now, with these easy solutions up your sleeve, you can confidently fire and handle those "advanced" HTTP requests with java.net.URLConnection! π
π Your Call-to-Action: Share your experiences with using java.net.URLConnection to fire "advanced" requests in the comments below. Have you encountered any hurdles? Do you have additional tips to share? Let's spark a productive discussion! β¨π£οΈ
Remember, internet superheroes unite! Save this post and share it with your tech-savvy friends who could use some HTTP request mastery in their lives! π¦ΈββοΈπ¦ΈββοΈ
Happy coding! π»π₯πͺ