How to use Jackson to deserialise an array of objects
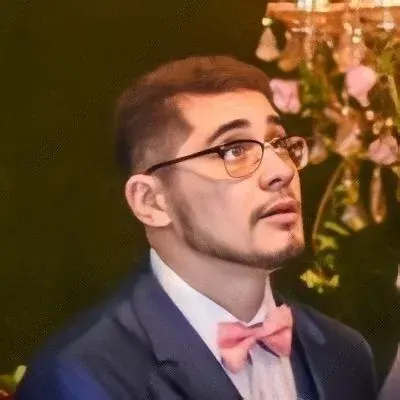
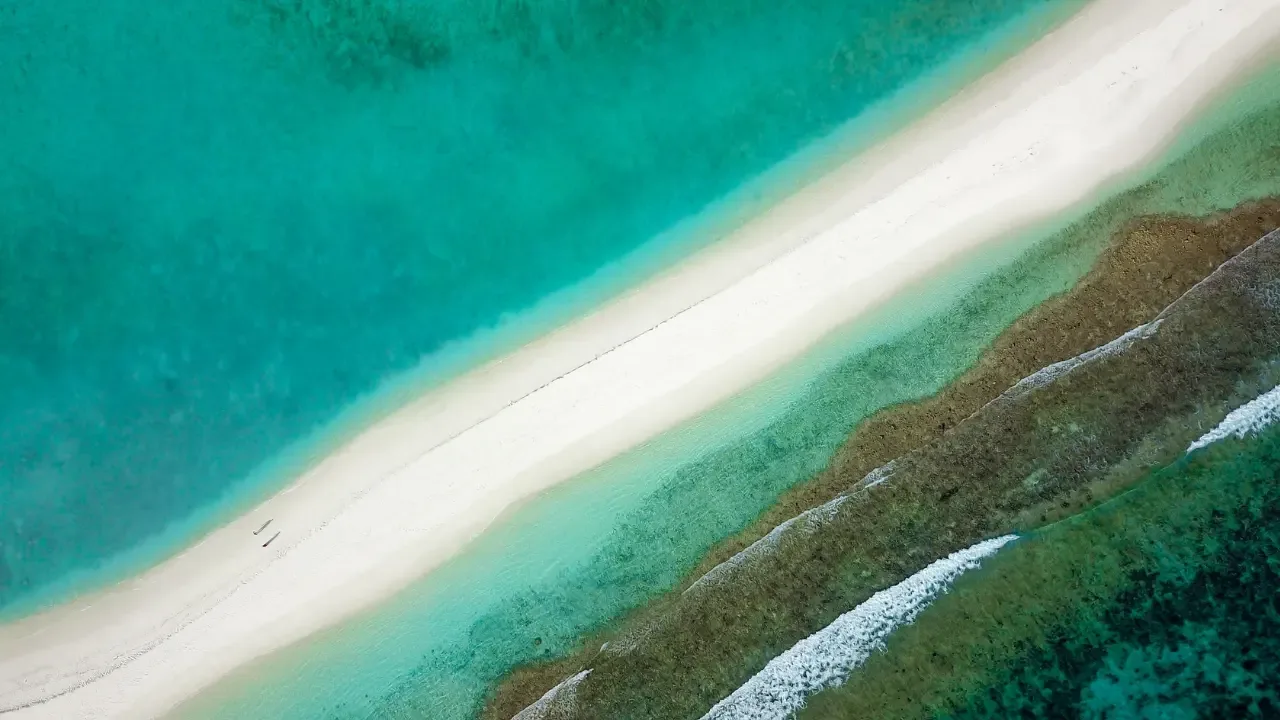
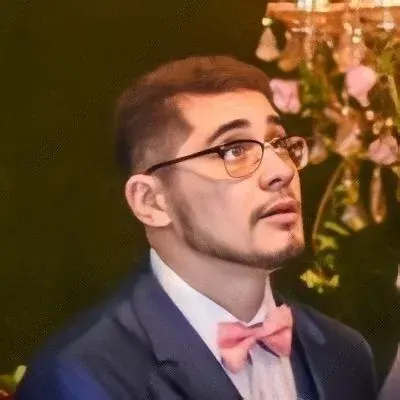
🚀 A Comprehensive Guide to Deserializing an Array of Objects using Jackson
Do you find yourself struggling to deserialize an array of objects using Jackson? Look no further! In this guide, we'll walk you through the steps to deserialize JSON arrays into Java objects using the powerful Jackson library. Let's dive in! 💪
The Challenge: Deserializing an Array of Objects
So, you've managed to deserialize a single JSON object using Jackson, but now you want to level up and handle arrays. Let's take a look at how we can achieve this in an efficient and elegant way. 🤔
The Solution: Utilizing Jackson's ObjectMapper
To deserialize an array of objects, we need to leverage Jackson's ObjectMapper
class. Here's how you can approach it:
Create an instance of the
ObjectMapper
class, which is responsible for handling the JSON-to-Java conversion.
ObjectMapper objectMapper = new ObjectMapper();
To deserialize a JSON array into a Java List, we can use the
readValue()
method with a combination of theTypeReference
class to preserve the generic type information. Here's an example:
String json = "[{\"id\" : \"junk\", \"stuff\" : \"things\"}, {\"id\" : \"spam\", \"stuff\" : \"eggs\"}]";
List<MyClass> entries = objectMapper.readValue(json, new TypeReference<List<MyClass>>(){});
In the above code snippet, we define the desired generic type List<MyClass>
inside the TypeReference
. This ensures that Jackson correctly converts the JSON array into a list of MyClass
objects.
Handling Common Issues
Issue 1: Dealing with an Empty Array
What if the JSON array is empty? 🤷♂️ Don't worry, Jackson has you covered. The readValue()
method returns an empty list if the JSON array is empty, ensuring our code handles this scenario gracefully.
Issue 2: Handling Nested Objects in the Array
What if your JSON array contains nested objects or complex structures? 🤔 Fortunately, Jackson's flexibility shines here too! You can define a class hierarchy that matches your JSON structure, allowing Jackson to handle the deserialization effortlessly. Jackson can even handle nested arrays within your objects!
Want to Become a Jackson Ninja?
Now that you have a clear understanding of how to deserialize JSON arrays using Jackson, it's time to put your skills to the test! 🥋 Experiment with different JSON array structures, try nesting arrays, and explore the advanced features of Jackson. The possibilities are endless!
Remember, practice makes perfect, so go ahead and code your way to becoming a Jackson ninja! 💪🔥
Conclusion
Deserializing an array of objects using Jackson doesn't have to be a daunting task. By utilizing Jackson's powerful ObjectMapper
class and the readValue()
method, you can effortlessly convert JSON arrays into Java objects.
Next time you encounter this challenge, refer back to this guide, and you'll be deserializing arrays like a pro! 🎉
So, what are you waiting for? Start exploring the amazing world of Jackson and share your experiences with us in the comments below! 😊
💌 Don't forget to share this post with your fellow developers and help them conquer the art of deserializing JSON arrays using Jackson! 🚀