How to update a value, given a key in a hashmap?
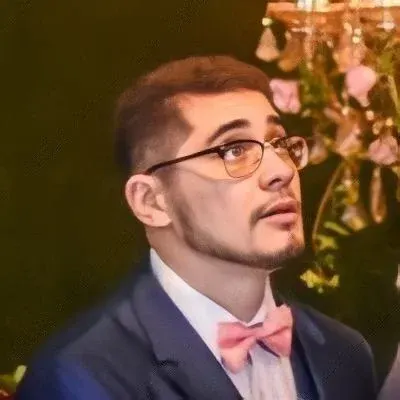
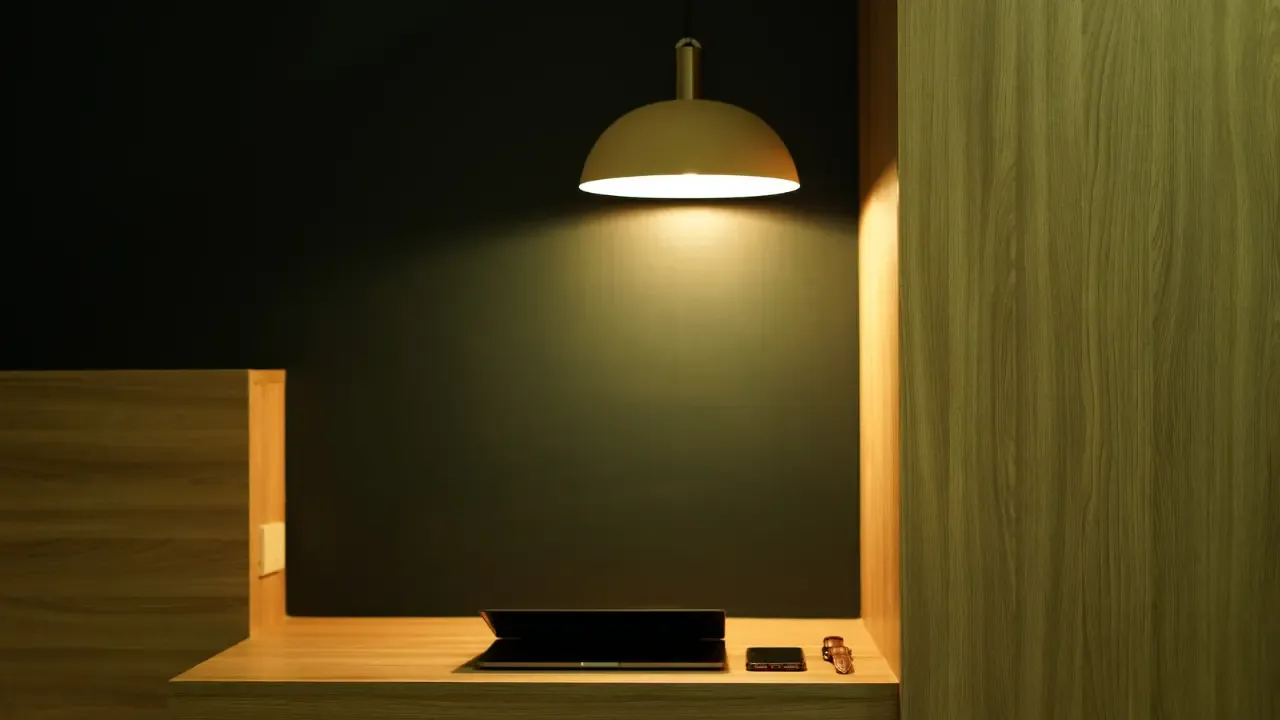
💡 Updating a Value in a Hashmap: Easy Solutions and Common Issues
Introduction
Welcome to my blog! In today's post, we'll discuss a common question: how to update a value in a hashmap given a key. We'll explore easy solutions to this problem and address common issues that may arise along the way. So, let's dive in and find out how to conquer this challenge!
Understanding the Scenario
Let's set the context first. Suppose we have a HashMap<String, Integer>
in Java, where the keys are strings and the values are integers. Our goal is to update (increment) the integer value for a specific string key whenever we find multiple occurrences of that key. But how can we achieve this without unnecessary overhead?
Solution: Putting the New Pair
One simple approach to updating a value in a hashmap is to put the new pair, replacing the old one. In Java, the put()
method allows us to achieve this without much hassle. Here's a code snippet to illustrate this solution:
HashMap<String, Integer> hashMap = new HashMap<>();
String key = "example";
Integer oldValue = hashMap.get(key);
if (oldValue != null) {
hashMap.put(key, oldValue + 1);
} else {
hashMap.put(key, 1);
}
In the code snippet above, we first retrieve the current value associated with the key using the get()
method. If the value is not null
, it means the key already exists in the hashmap. We then update the value by incrementing it, and put the new pair back into the hashmap using the put()
method. If the value is null
, it means the key is new, so we simply put the key and assign the initial value of 1.
Hashcode Collision: Handling New Key Insertion
Now, let's address a potential concern when inserting a new key into the hashmap. What if there is a hashcode collision with a recently inserted key? How should the hashmap behave in this situation?
Hashcode collisions occur when different objects generate the same hashcode, which can lead to a collision in the hashmap's internal data structure. In such cases, the correct behavior for a hashmap would be to either assign a different location for the new key or create a list (known as a bucket) to handle multiple entries with the same hashcode.
Fortunately, Java's HashMap
implementation handles hashcode collisions efficiently. It internally uses linked lists (buckets) to store multiple entries with the same hashcode. When a collision occurs, the new key-value pair is simply appended to the existing bucket, ensuring all values are stored correctly.
Conclusion
Updating a value in a hashmap may seem daunting at first, but with the right approach, it becomes a straightforward task. Remember, you can update a value by putting a new pair with the desired key, making sure to handle collisions gracefully. Java's HashMap
implementation takes care of collision handling, so you can focus on updating values efficiently.
I hope this guide has provided you with easy solutions and insights into common issues related to updating values in a hashmap. Now it's your turn to put this knowledge into action! Share your experiences, questions, and feedback in the comments section below. Happy coding! 💻🎉
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
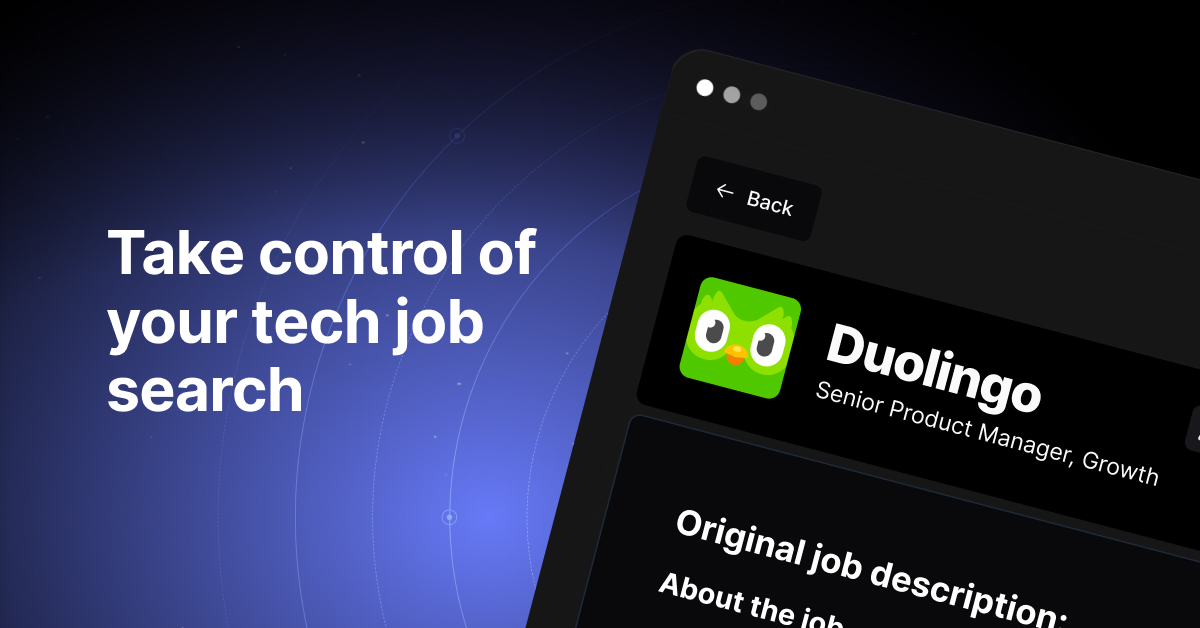