How to tell Jackson to ignore a field during serialization if its value is null?
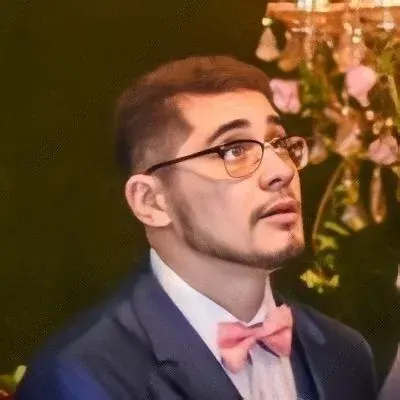
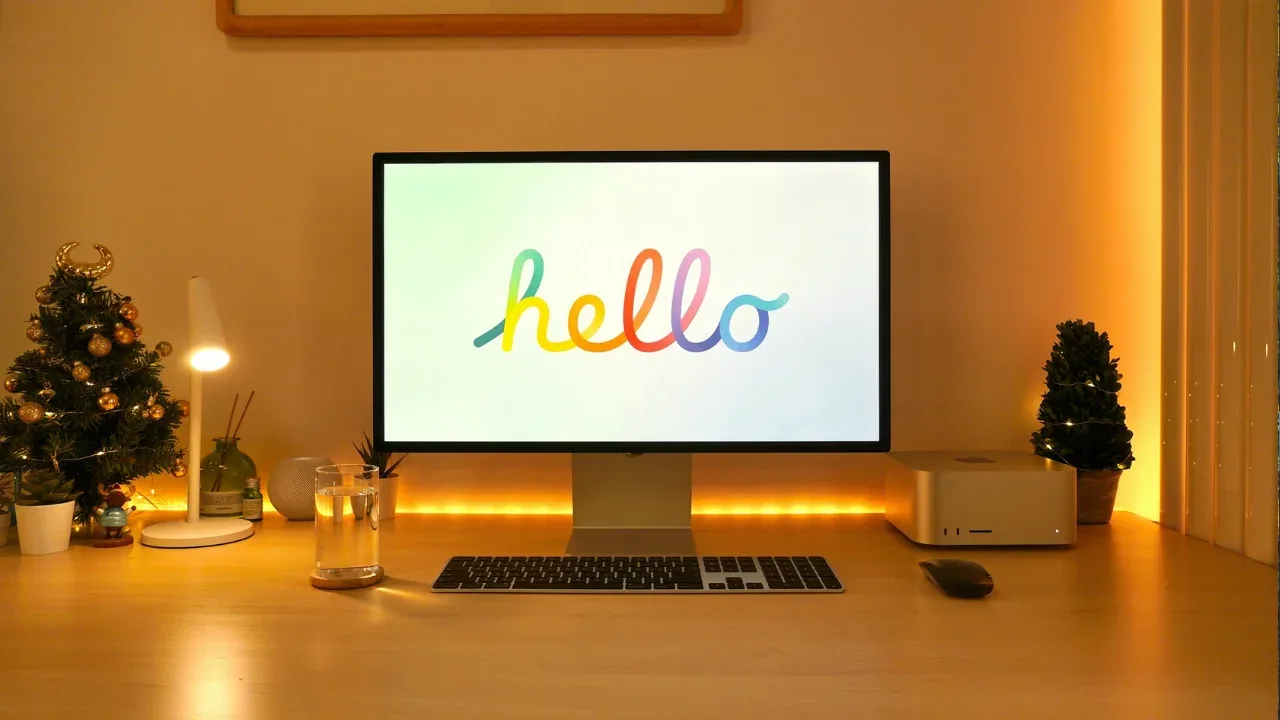
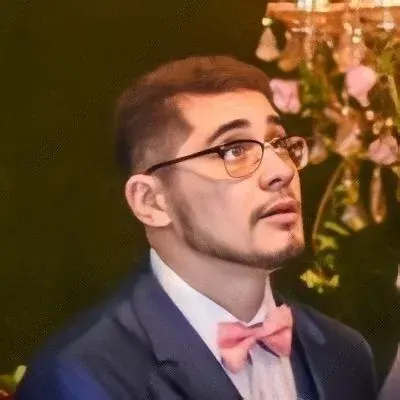
๐๐ Hey there, tech enthusiasts! ๐ Are you struggling with how to make Jackson ignore a field during serialization if its value is null? No worries, I've got you covered! In this blog post, we'll dive into this common issue and explore easy solutions to ensure smooth sailing with Jackson. So, let's get started! ๐๐
The Problem: How to Ignore a Null Field during Serialization?
Imagine you have a Java class SomeClass
and it has a field called someValue
. Now, you want Jackson to skip serializing this field if its value is null. But if it contains a value, you want it to be serialized. Sounds familiar? Let me show you an example:
public class SomeClass {
private String someValue;
}
So, the challenge here is: How do we configure Jackson to ignore the someValue
field during serialization if its value is null? ๐ค
Solution: The Magic of Jackson Annotations โจ
To achieve this behavior, we can make use of Jackson annotations. โ๏ธ These annotations provide configuration options to customize the serialization and deserialization process. In our case, we'll use the @JsonInclude
annotation.
Here's how you can modify the SomeClass
to achieve the desired behavior:
public class SomeClass {
@JsonInclude(JsonInclude.Include.NON_NULL)
private String someValue;
}
By adding @JsonInclude(JsonInclude.Include.NON_NULL)
above the someValue
field, we're instructing Jackson to exclude the field during serialization if its value is null. But if the someValue
field has a non-null value, Jackson will include it in the serialized output.
Let's Test It Out! ๐งช
Now that we know how to solve the problem, let's validate our solution with a simple test. ๐ Assume we have an instance of SomeClass
where someValue
is null:
SomeClass obj = new SomeClass();
obj.setSomeValue(null);
If we serialize this object using Jackson, we should observe that the field someValue
is not present in the resulting JSON output. Let's see the code snippet to serialize this object and the expected output:
ObjectMapper objectMapper = new ObjectMapper();
String json = objectMapper.writeValueAsString(obj);
System.out.println(json);
// Output: {}
Voila! The someValue
field is successfully ignored during serialization since its value is null. ๐
You're Ready to Go! ๐
Congratulations! ๐ฅณ You have learned how to tell Jackson to ignore a field during serialization if its value is null. And remember, all it takes is a little annotation magic with @JsonInclude(JsonInclude.Include.NON_NULL)
.
Now go ahead and implement this trick in your code to make your serialization process a breeze. Enjoy the flexibility and control offered by Jackson annotations. ๐
But wait, don't keep this knowledge to yourself! Share this blog post with your fellow developers who might be facing the same issue. Let's spread the word and help others master the art of Jackson serialization. Click that share button now! ๐ข
As always, if you have any questions or want to share your thoughts, feel free to leave a comment below. Let's engage in a fruitful discussion. Happy coding! ๐ป๐ก