How to split a string with any whitespace chars as delimiters
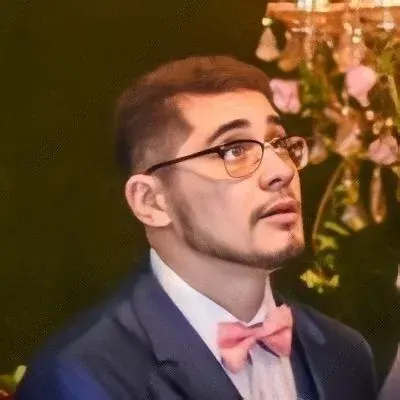
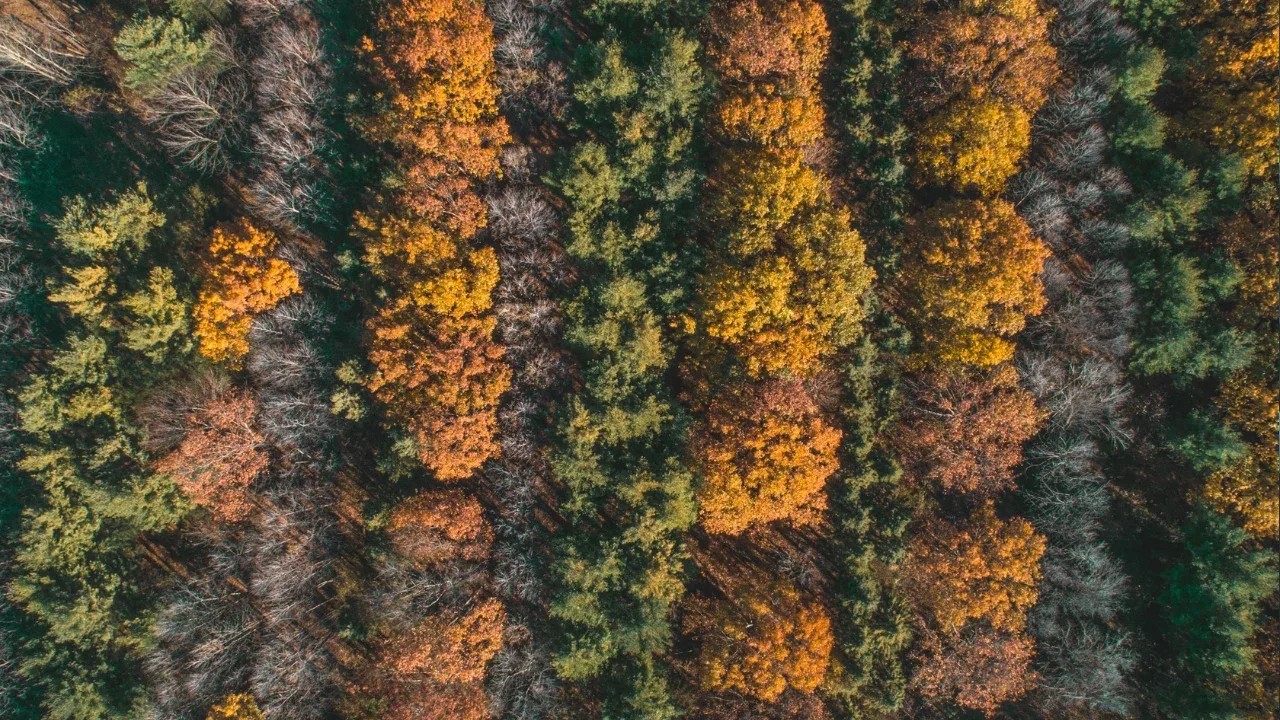
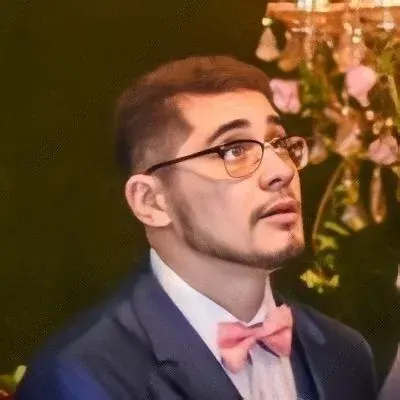
Splitting Strings with Whitespace Characters: A Complete Guide
Welcome to another tech blog post! Today, we're going to address a common issue faced by developers when it comes to splitting strings with any whitespace characters as delimiters. It's a tricky problem, but fear not! We have easy solutions for you. 😄
The Problem
So you have a string that you want to split into an array of substrings, using all whitespace characters (spaces, tabs, newlines, etc.) as delimiters. You want to achieve this using the java.lang.String.split()
method. But what regex pattern should you pass as the argument?
The Solution
Here's the magic regex pattern you need: \\s+
. 😎
Let's break it down:
The double backslash,
\\
, is required because we need to escape the backslash character in Java strings.The
s
is the metacharacter for matching whitespace characters.The
+
matches one or more occurrences of the preceding pattern, in this case, one or more whitespace characters.
By passing this regex pattern to the split()
method, you'll achieve the desired result!
Example Code
String str = "Hello\tworld! How\nare you?";
String[] substrings = str.split("\\s+");
In this code snippet, the string str
contains a mixture of spaces, tabs, and newlines. By invoking the split()
method with the regex pattern \\s+
, we get an array substrings
that contains all the individual words: ["Hello", "world!", "How", "are", "you?"]
. Awesome, right? 😄
Common Issues
Issue #1: Including leading/trailing empty substrings
By default, the split()
method eliminates any leading or trailing empty substrings. However, in some cases, you might want to preserve them. To achieve this, you can use the overloaded split()
method with a second argument: the maximum number of substrings you want to return.
For example:
String str = "Hello World";
String[] substrings = str.split("\\s+", -1);
In this code snippet, the resulting array will include the leading and trailing empty substrings: ["Hello", "", "", "World"]
.
Issue #2: Handling multiple consecutive whitespace characters
The regex pattern \\s+
matches one or more whitespace characters. It treats consecutive whitespace characters as a single delimiter. If you want to treat consecutive whitespace characters as separate delimiters, you need to modify the regex pattern.
For example:
String str = "Hello World";
String[] substrings = str.split("(?<=\\s)|(?=\\s+)");
In this code snippet, the resulting array will have individual substrings for each whitespace character: ["Hello", "", "", "", "World"]
.
Reader Engagement
We hope you found this guide helpful in tackling the challenge of splitting strings with whitespace characters as delimiters. Try implementing the solutions in your next project and see the magic happen! If you have any questions or alternative solutions to share, we'd love to hear from you in the comments below. Let's level up our regex skills together! 💪🚀