How to split a String by space
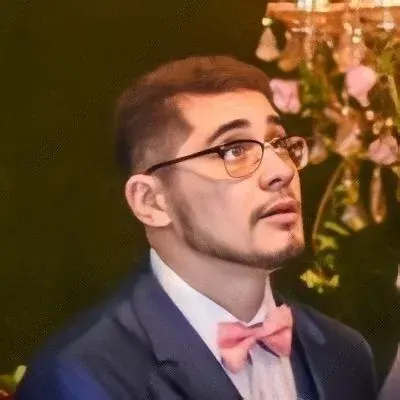
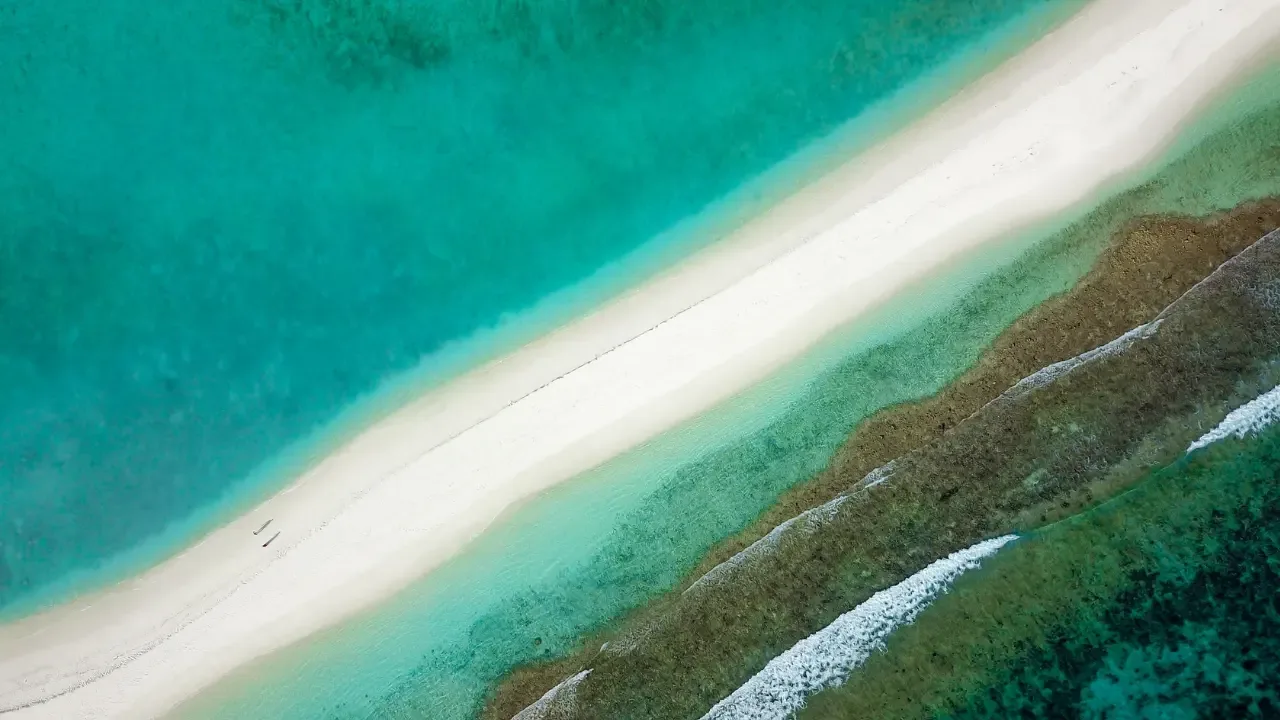
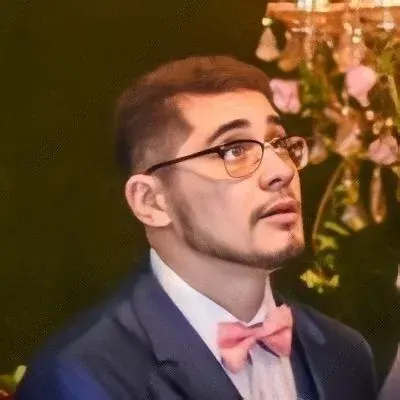
How to Split a String by Space: Decode the Mystery
š Welcome, fellow coders! Today, we are going to decode the ā”ļø elusive mystery of splitting a string by space ā”ļø. You've come to the right place if you're tired of scratching your head š¤ and want an easy solution, without compromising on the coolness factor. Let's dive in! š»š
The Enigma: String Splitting by Space
So, you have this string that you want to break into smaller pieces every time you encounter a space. Seems like a piece of cake, right? At first glance, you might even think you've got it covered with the following code snippet:
str = "Hello I'm your String";
String[] splited = str.split(" ");
The Conundrum: It Doesn't Work ā
But alas! Reality hits and you discover that your code isn't working š. The resulting splited
array doesn't hold the expected pieces of your string. š¢
The Solution: Decode the Riddle
Fear not! We are here to guide you towards the light. Let's dissect the problem and resolve it step by step.
Unraveling the Mystery: Double-Check the Whitespaces
Before diving deeper, double-check your string to ensure that it actually contains spaces. It seems obvious, but hey, it's always good to be thorough.
System.out.println(str.contains(" ")); // Check if string contains a space
The Split Method: Beware of Regex
The split
method in Java uses regular expressions as the separator. š§āāļø So when you pass a space as a separator, the split
method will consider any number of consecutive spaces as a single separator. This design might not be intuitive, but that's just how it works.
The Escaped Space: Unleash the Power of Regex
To split your string by each space individually, you'll have to use the power of regex. šŖ Here's the secret sauce:
String[] splited = str.split("\\s");
By using \\s
as the separator, we are explicitly telling Java to split the string at every whitespace character (including spaces, tabs, and line breaks). š¤©
The Conclusion: String Splitting Unveiled
Congratulations, you've cracked the code! š Now you can split your string into smaller parts by just using the magic snippet below:
String[] splited = str.split("\\s");
Remember, regex can be like a š©ļø thunderstorm, but once you understand its power, you can harness it to solve all sorts of string splitting puzzles.
Take It to the Next Level: Share Your Experience
Now that you've mastered the art of splitting a string by space, it's time to share your excitement with fellow coders. Comment below š and let us know how this tip helped you. Do you have any mind-boggling string manipulation challenges you'd like us to tackle? We're all ears! š
Until next time, happy coding! š»āļø