How to split a string, but also keep the delimiters?
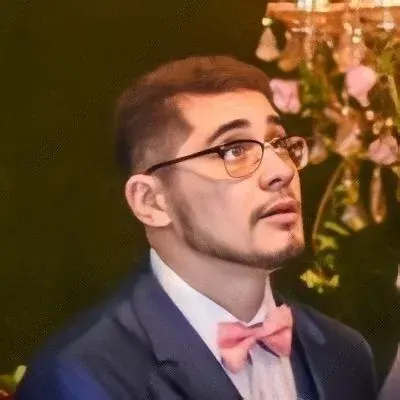
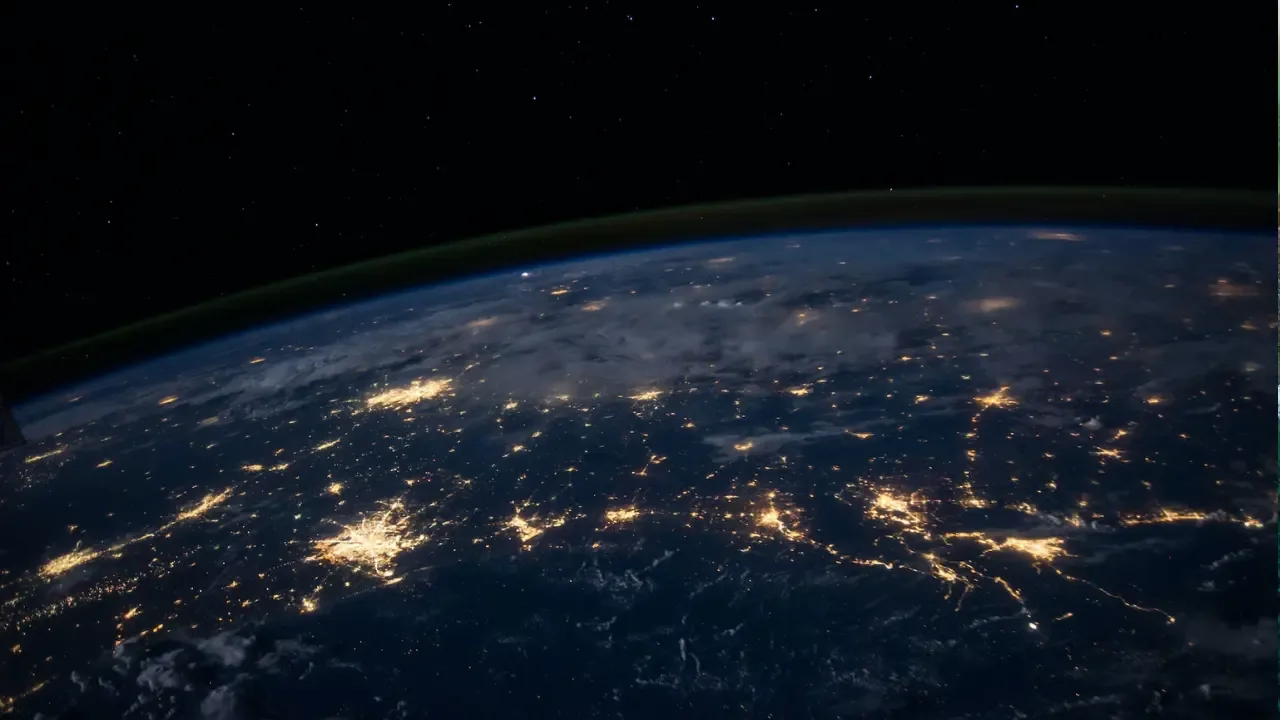
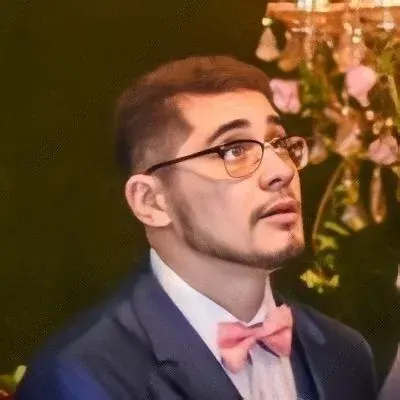
How to Split a String and Keep the Delimiters: A Complete Guide
Are you stuck trying to split a string into parts while also keeping the delimiters intact? 😩 Don't worry, we've got you covered! In this guide, we'll walk you through the common issues and provide easy solutions to this problem. So let's dive right in! 💪
Understanding the Problem
You may have come across a multiline string that is delimited by different delimiters. But when you try to split it using String.split(regex)
, you only get the parts of the string and lose the actual delimiters. 😓 Let's consider an example:
(Text1)(DelimiterA)(Text2)(DelimiterC)(Text3)(DelimiterB)(Text4)
Using String.split
, you would get the following result:
Text1
Text2
Text3
Text4
But what you actually want is to keep the delimiters and get the following result:
Text1
DelimiterA
Text2
DelimiterC
Text3
DelimiterB
Text4
Solution: Lookaround Assertions
To achieve the desired result, we can leverage the power of lookaround assertions in regular expressions. Lookaround assertions allow us to match patterns based on what comes before or after them, without consuming the characters.
In our case, we want to split the string at the positions where delimiters occur, but we also want to include the delimiters themselves in the result. Here's how you can do it:
String input = "(Text1)(DelimiterA)(Text2)(DelimiterC)(Text3)(DelimiterB)(Text4)";
String regex = "(?<=\\))(?=\\()";
String[] result = input.split(regex);
Let's break down the regular expression (?<=\\))(?=\\()
(?<=\\))
is a positive lookbehind assertion that matches the position right after a closing parenthesis ')' without including it in the match.(?=\\()
is a positive lookahead assertion that matches the position right before an opening parenthesis '(' without including it in the match.
By splitting the input string using this regular expression, we achieve the desired result.
Example Code
Here's an example code snippet that you can try:
public class StringSplitExample {
public static void main(String[] args) {
String input = "(Text1)(DelimiterA)(Text2)(DelimiterC)(Text3)(DelimiterB)(Text4)";
String regex = "(?<=\\))(?=\\()";
String[] result = input.split(regex);
for (String part : result) {
System.out.println(part);
}
}
}
Conclusion
Splitting a string while keeping the delimiters may seem tricky, but with the power of lookaround assertions, it becomes a breeze. By using a regular expression with lookbehind and lookahead assertions, you can easily achieve the desired result.
So the next time you find yourself in a similar situation, remember to leverage lookaround assertions to split your string and keep those delimiters intact! 🎉
Now it's your turn! Have you ever encountered this problem? How did you solve it? Comment below and let's discuss! 💬