How to set base url for rest in spring boot?
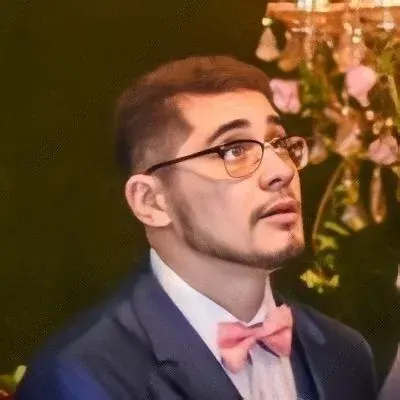
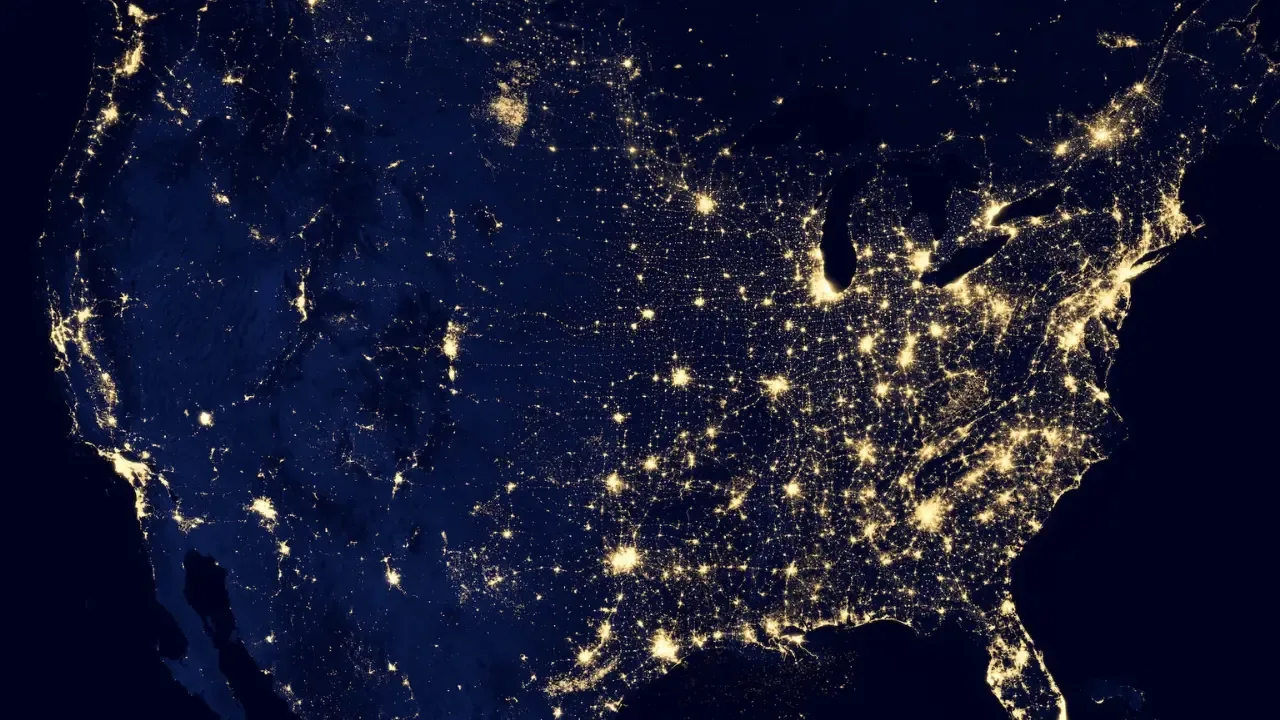
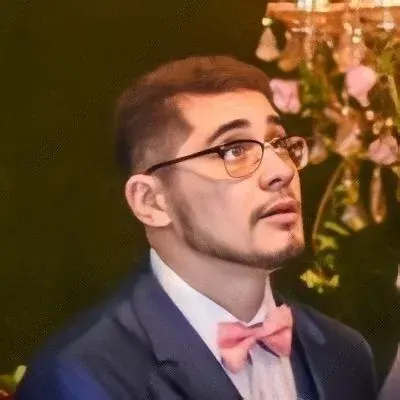
🌐 How to Set Base URL for REST in Spring Boot? 🚀
So, you want to mix MVC and REST in a single Spring Boot project, eh? And you want to set a base path for all your REST controllers in a single place? No worries, mate! I gotchu covered! 😎
One common approach to achieving this is by utilizing the @RequestMapping
annotation in Spring Boot. This nifty little annotation allows you to specify the base path for your REST controllers.
🛠️ Solution
Here's a step-by-step guide to help you set the base URL for REST in your Spring Boot project:
Open your
application.properties
orapplication.yml
file.In this file, add the following line:
server.servlet.context-path=/api
or
server: servlet: context-path: /api
In the above code,
/api
represents the base path you want to set for your REST controllers. Feel free to replace it with any path of your choice.Save the file and restart your Spring Boot application. 🔄
Voila! 🎉 Your REST controllers are now accessible at the base path /api
.
Now, if you want to access your MVC controllers at a different base path (let's say /whatever
), here's what you need to do:
Create a new class, let's call it
MvcConfig
.Annotate this class with
@Configuration
.Implement the
WebMvcConfigurer
interface in yourMvcConfig
class.Override the
addViewControllers
method like this:@Configuration public class MvcConfig implements WebMvcConfigurer { @Override public void addViewControllers(ViewControllerRegistry registry) { registry.addViewController("/whatever/**").setViewName("forward:/"); } }
In the above code,
/whatever/**
represents the base path for your MVC controllers. You can replace it with any path of your choice.Save the file and restart your Spring Boot application. 🔄
Fantastic! Now your MVC controllers should be easily accessible at the base path /whatever
. 🎉
📣 Call-to-Action
There you have it, folks! 💪 Setting the base URL for REST in your Spring Boot project can be as easy as pie. With these simple steps, you'll be able to streamline your REST and MVC controllers without any hassle.
So, why not give it a try and level up your Spring Boot game? 👩💻👨💻
If you found this guide helpful, don't forget to share it with your fellow developers and spread the knowledge! 🚀
Got any questions or suggestions? Leave a comment below and let's discuss! Happy coding! 😉✨