How to round a number to n decimal places in Java
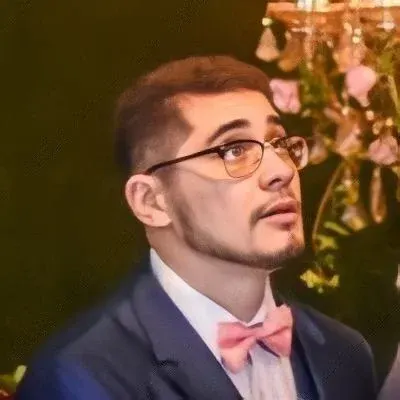
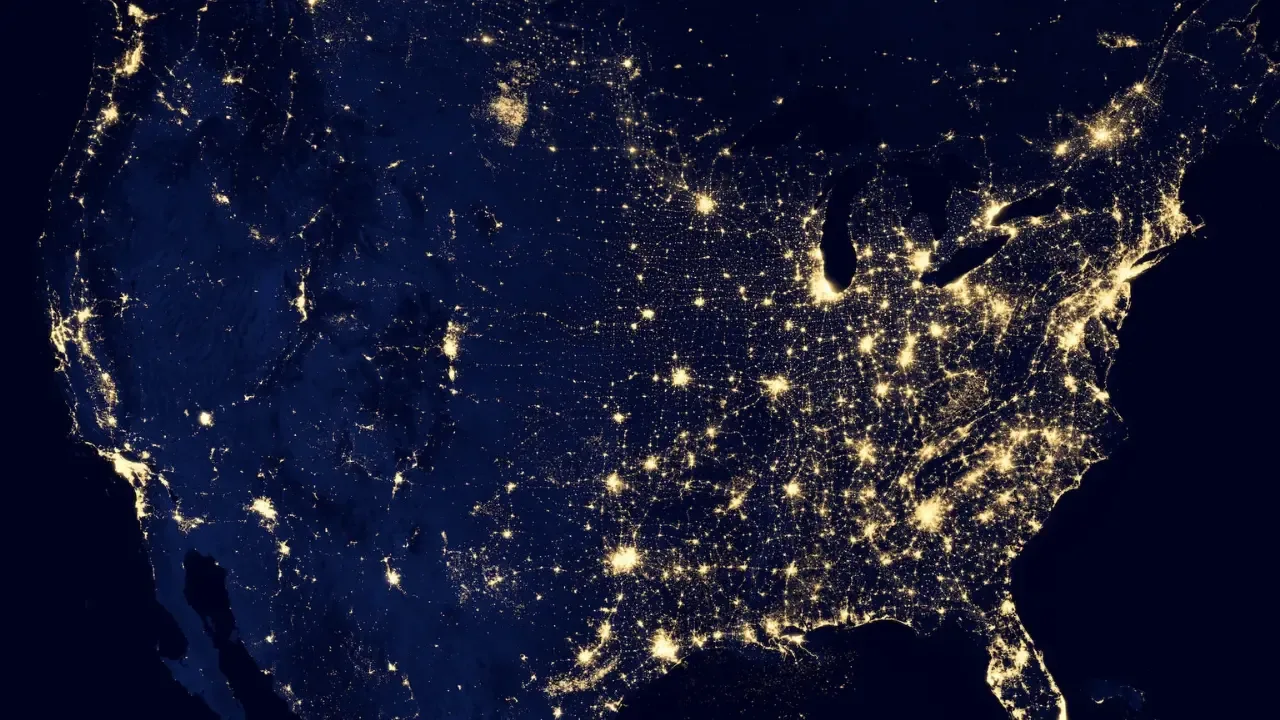
How to Round a Number to n Decimal Places in Java 😃💻
So you want to round a number in Java to a specific number of decimal places, huh? Don't worry, I've got your back. In this guide, I'll show you a couple of methods to accomplish this, addressing common issues and providing easy solutions. Let's dive in! 🏊♂️
The Problem 🤔
The user wants to round a number using the "half-up" method, which means rounding up if the decimal to be rounded is 5 or above. Additionally, they only want to display significant digits without any trailing zeros. They've already tried using String.format
and DecimalFormat
, but none of them fully satisfy their requirements.
Solution 1: String.format
🎯
The first method they tried, String.format
, gets pretty close to what they want. Here's an example:
String formattedNumber = String.format("%.5g%n", 0.912385);
This would return 0.91239
, which is rounded to 5 decimal places as desired. However, the problem arises when we have numbers like 0.912300
, which would be displayed as 0.91230
with trailing zeros. 😕
Solution 2: DecimalFormat
🎯
The user also attempted to use DecimalFormat
, which is another option. Here's an example:
DecimalFormat df = new DecimalFormat("#.#####");
String formattedNumber = df.format(0.912385);
This time, the rounding doesn't have trailing zeros, which is great. However, the rounding behavior is slightly different from what they expect. It uses the "half-even" rounding, where it rounds down if the previous digit is even. What they want is straightforward: round up whenever the decimal to be rounded is 5 or above. 😟
Solution 3: Custom Method 💡
To achieve the desired rounding behavior, we can create a custom method that takes care of both the rounding and trailing zeros. Here's an example implementation:
public static String roundToNDecimalPlaces(double number, int decimalPlaces) {
double powerOfTen = Math.pow(10, decimalPlaces);
double roundedNumber = Math.round(number * powerOfTen) / powerOfTen;
if (decimalPlaces == 0) {
return String.valueOf(Math.round(roundedNumber));
} else {
return String.valueOf(roundedNumber);
}
}
Now, let's see this method in action:
String formattedNumber = roundToNDecimalPlaces(0.912385, 5);
This would return 0.91239
, and for 0.912300
, it would return 0.9123
without any trailing zeros. Perfect! 🎉
Wrapping Up and Your Turn! 🌟🗣️
There you have it! Three different approaches to round a number to a specific number of decimal places in Java. While String.format
and DecimalFormat
provide a good starting point, our custom method allows for greater control over rounding behavior and trailing zeros.
Now it's your turn to try it out! Implement these methods in your code and see which one fits your requirements best. Do you have any other cool ways to achieve this? Let us know in the comments below! Let's all make our Java programs more precise and polished! 💪💯
Note: Don't forget to import the necessary classes and methods before using them in your code.
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
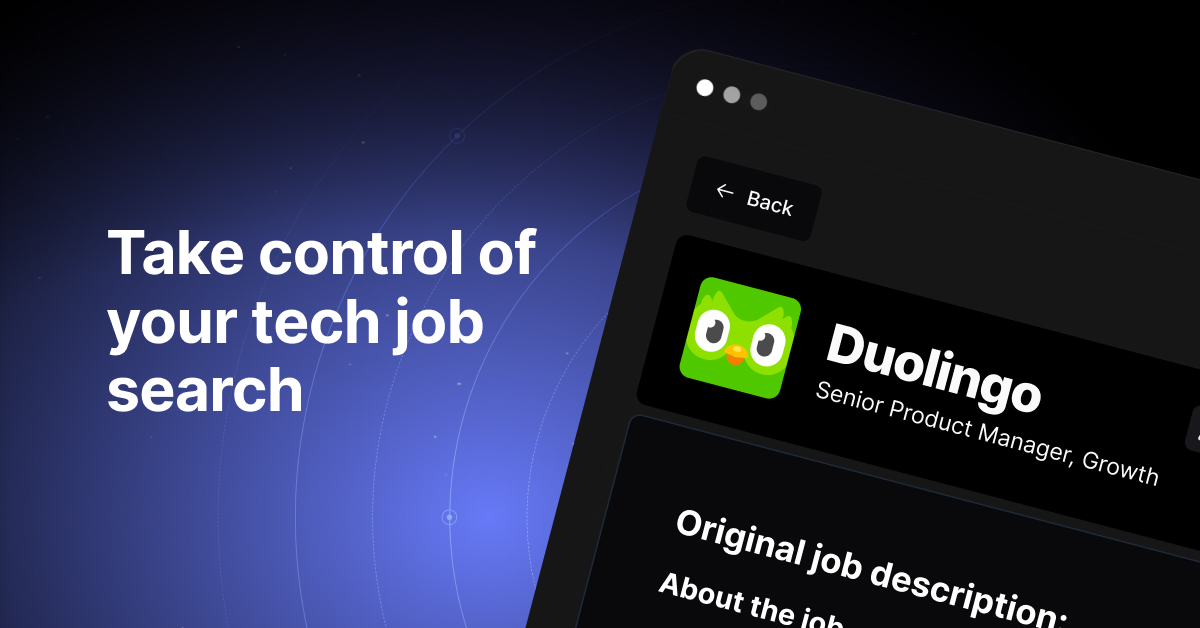