How to return a custom object from a Spring Data JPA GROUP BY query
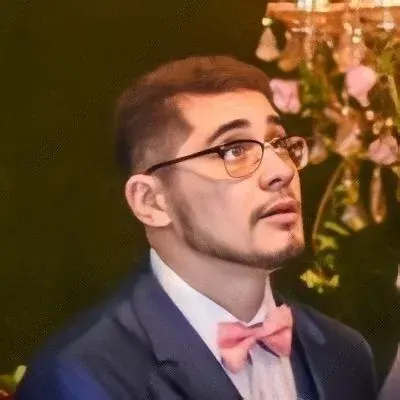
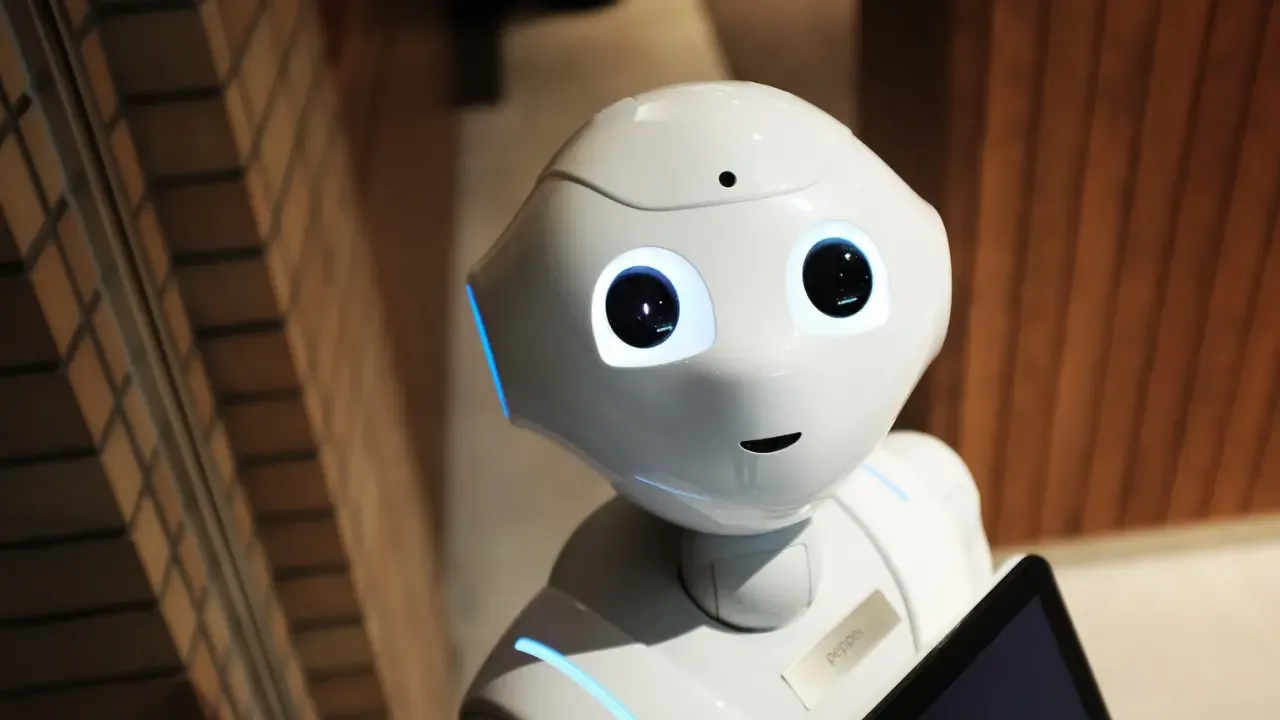
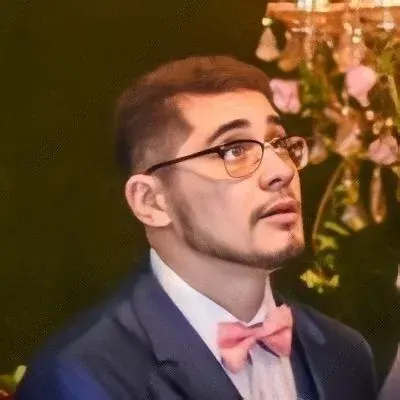
How to return a custom object from a Spring Data JPA GROUP BY query 😎💪
So you're working on a Spring Boot application, utilizing the power of Spring Data JPA for your data access needs. You've come across a situation where you need to perform a GROUP BY query and return a custom object instead of the default result set. 🤔
In your case, you have successfully written a custom JPQL query to group by a field and get the count. However, the result you obtain is not exactly what you're looking for. You want to transform the result into a list of objects containing the count and the answer. 📊
Here's the repository method you are currently using:
@Query(value = "select count(v) as cnt, v.answer from Survey v group by v.answer")
public List<?> findSurveyCount();
And the current result you obtain:
[
[1, "a1"],
[2, "a2"]
]
But you desire a result like this:
[
{ "cnt": 1, "answer": "a1" },
{ "cnt": 2, "answer": "a2" }
]
The good news is that achieving this is actually quite straightforward! 😀
What you need to do is create a custom class to represent your desired result and modify your repository method accordingly. Let's call this class SurveyCountDto
. Here's an example:
public class SurveyCountDto {
private Long cnt; // Count
private String answer;
public SurveyCountDto(Long cnt, String answer) {
this.cnt = cnt;
this.answer = answer;
}
// Getters and setters
}
Now, update your repository method to return a list of SurveyCountDto
objects:
@Query(value = "select new com.example.SurveyCountDto(count(v) as cnt, v.answer) from Survey v group by v.answer")
public List<SurveyCountDto> findSurveyCount();
With this modification, the result you'll obtain will be exactly what you desire. 🎉
Remember to replace com.example
with the actual package name where SurveyCountDto
resides.
That's it! You've successfully returned a custom object from your Spring Data JPA GROUP BY query. Now you can further process and utilize the result in your application logic.
If you found this guide helpful, share it with your fellow developers who might also be struggling with returning custom objects from GROUP BY queries. 👥 Spread the knowledge!
Do you have any questions or suggestions? Share your thoughts in the comments below! Let's keep the conversation going! 💬😊