How to respond with an HTTP 400 error in a Spring MVC @ResponseBody method returning String
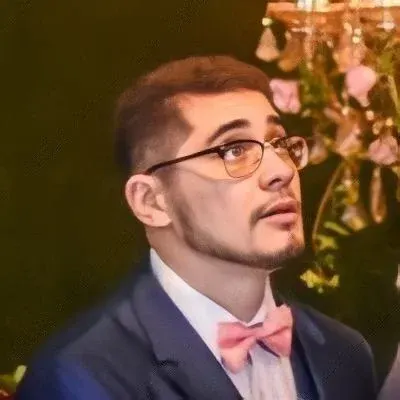
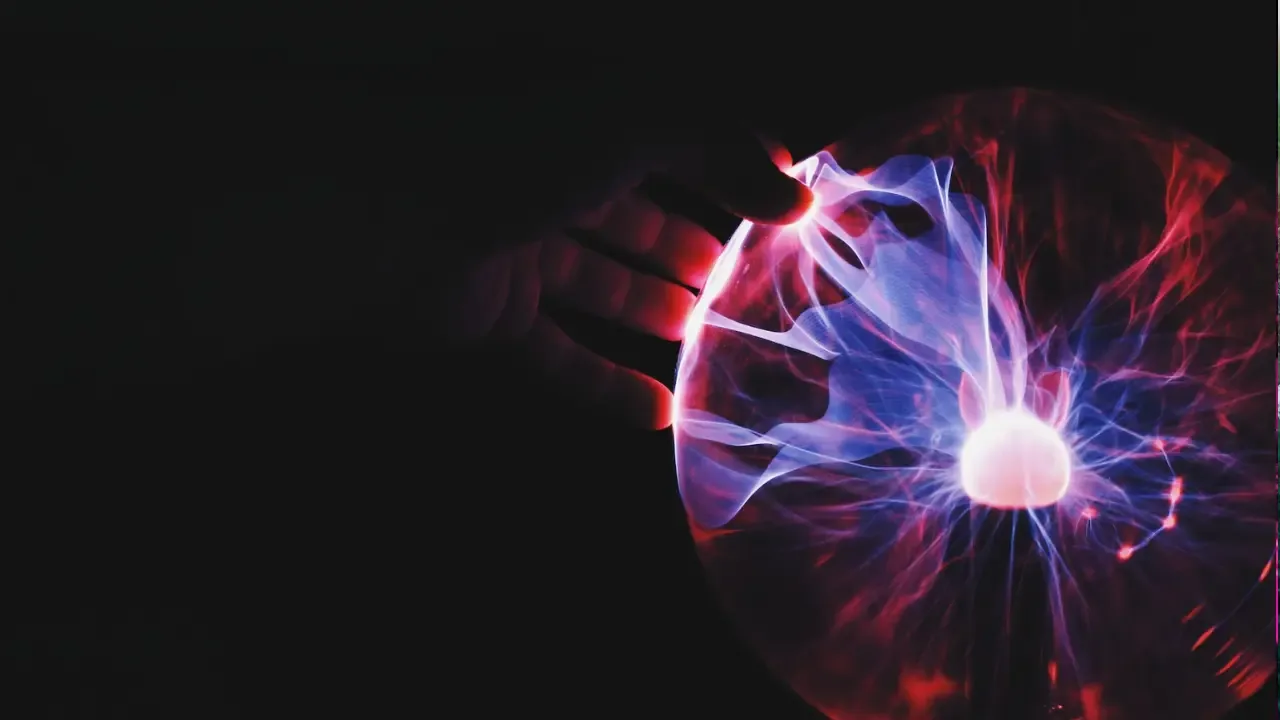
How to Respond with an HTTP 400 Error in a Spring MVC @ResponseBody Method Returning String 🤔
So you're using Spring MVC for a simple JSON API, and you want to know how to respond with an HTTP 400 error in a Spring MVC @ResponseBody
method when the method's return type is String
. Don't worry, we've got you covered! In this blog post, we'll address the common issues surrounding this problem and provide you with easy solutions. Let's dive right in! 💪
The Problem 🤷♂️
In the given scenario, you have a @RequestMapping
method that produces JSON and returns a String
. You want to respond with an HTTP 400 error when the json
variable is null
.
@RequestMapping(value = "/matches/{matchId}", produces = "application/json")
@ResponseBody
public String match(@PathVariable String matchId) {
String json = matchService.getMatchJson(matchId);
if (json == null) {
// TODO: how to respond with e.g. 400 "bad request"?
}
return json;
}
The Solution 💡
To respond with an HTTP 400 error in this scenario, we can make use of Spring's HttpServletResponse
object. Here's how we can accomplish that:
@RequestMapping(value = "/matches/{matchId}", produces = "application/json")
@ResponseBody
public void match(@PathVariable String matchId, HttpServletResponse response) {
String json = matchService.getMatchJson(matchId);
if (json == null) {
response.setStatus(HttpStatus.BAD_REQUEST.value());
return;
}
response.getWriter().write(json);
}
In the above code snippet, we're injecting the HttpServletResponse
object as a parameter in the match
method. We then set the response status to HTTP 400 using response.setStatus(HttpStatus.BAD_REQUEST.value())
.
Alternative Solution 🔄
If modifying the method signature to include HttpServletResponse
is not feasible, you can implement a custom exception handler to handle the HTTP 400 errors. Here's an example of how you can achieve this:
@ControllerAdvice
public class CustomExceptionHandler extends ResponseEntityExceptionHandler {
@ExceptionHandler(value = { IllegalArgumentException.class })
protected ResponseEntity<Object> handleBadRequest(RuntimeException ex, WebRequest request) {
String bodyOfResponse = "Bad request";
return handleExceptionInternal(ex, bodyOfResponse, new HttpHeaders(), HttpStatus.BAD_REQUEST, request);
}
}
With this approach, you throw an IllegalArgumentException
when you encounter a bad request. The exception is then caught by the handleBadRequest
method in the CustomExceptionHandler
class, which returns an HTTP 400 response with the message "Bad request".
Wrapping Up 🎉
Responding with an HTTP 400 error in a Spring MVC @ResponseBody
method returning a String
may seem tricky at first, but it doesn't have to be. By using the HttpServletResponse
object or implementing a custom exception handler, you can easily handle bad requests and provide meaningful responses to your API consumers.
Now it's your turn to try out these solutions! Let us know in the comments which approach worked best for you or if you have any other questions. Happy coding! 😄
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
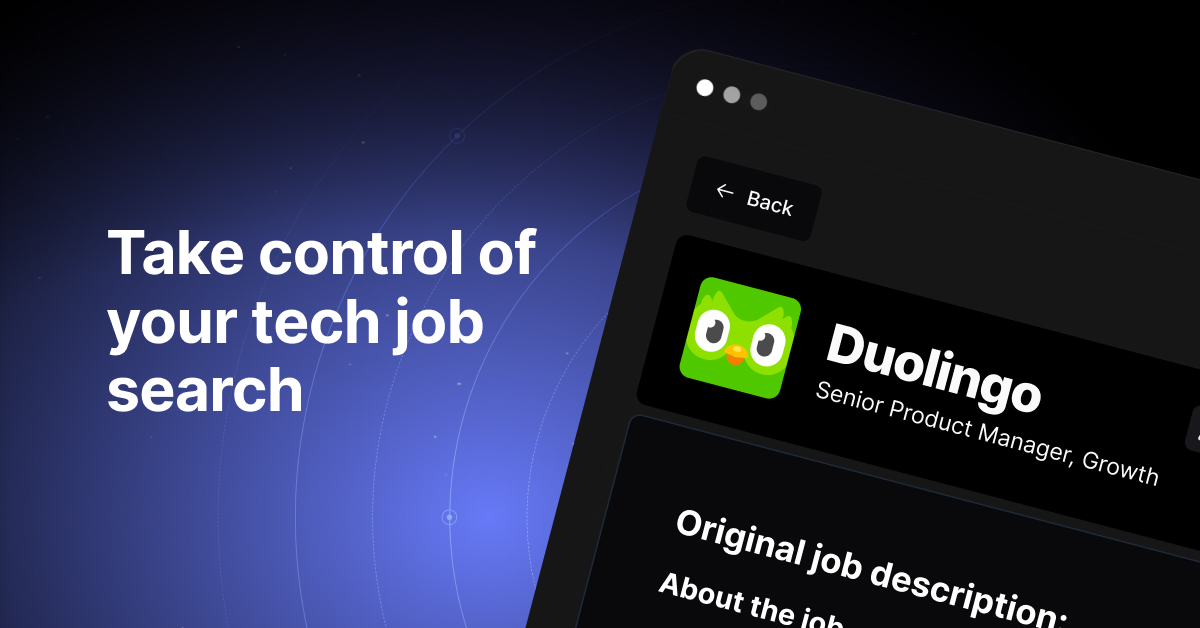