How to remove the last character from a string?
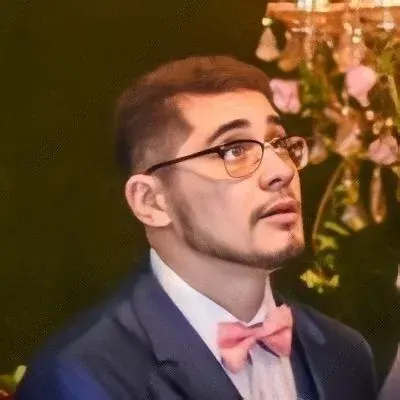
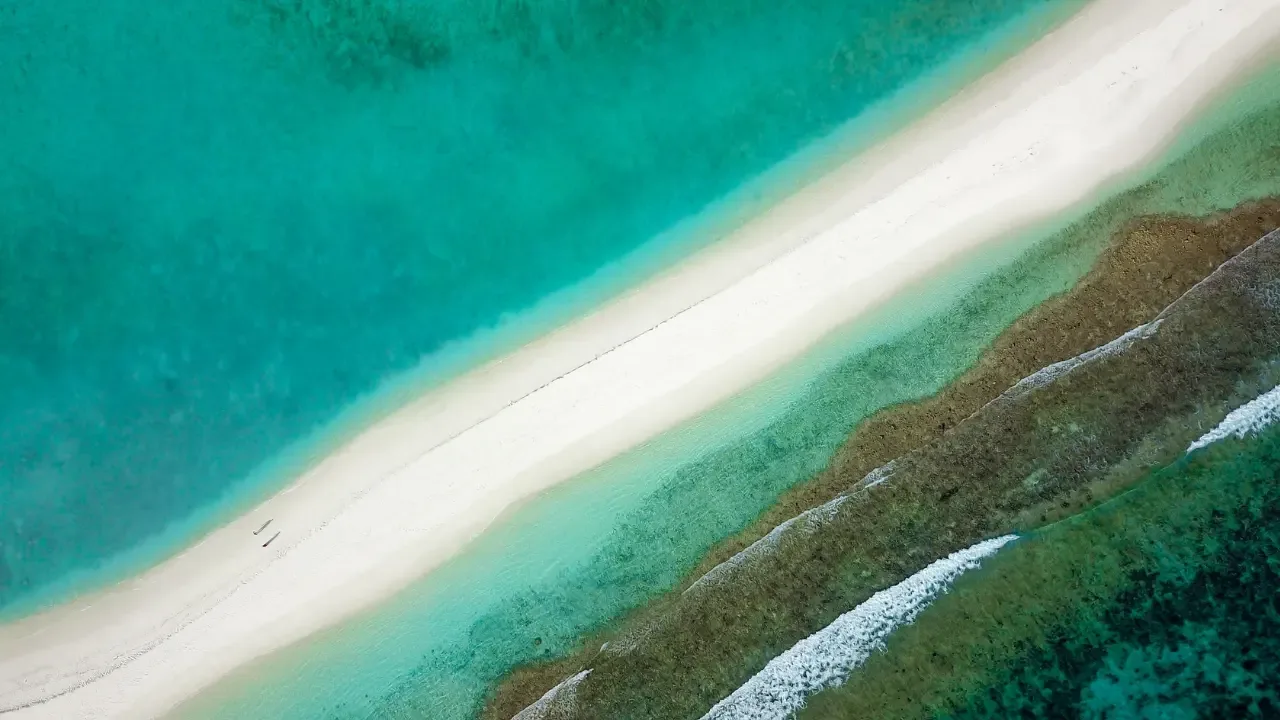
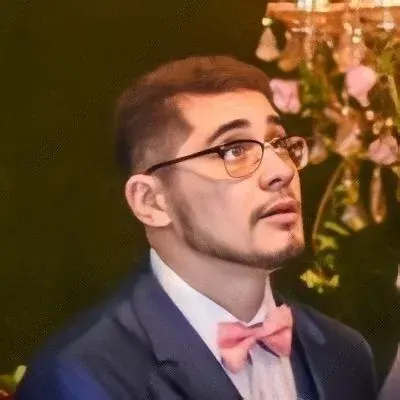
How to Remove the Last Character from a String 💥
Are you tired of getting unexpected results when trying to remove the last character from a string? You're not alone! Many developers face this issue and end up deleting more than just the last character.
In this guide, we will address this common problem and provide you with easy solutions to remove the last character from a string without any hassle. Let's dive in! 🚀
The Wrong Approach 😫
Before we look at the correct solutions, let's understand why the code snippet you provided doesn't work as expected.
public String method(String str) {
if (str.charAt(str.length()-1)=='x'){
str = str.replace(str.substring(str.length()-1), "");
return str;
} else{
return str;
}
}
The issue with this code is that it replaces all occurrences of the last character in the string, instead of just removing the last character. This causes unexpected behavior when there are other occurrences of the same character within the string.
Solution 1: Using Substring Method 👾
One way to correctly remove the last character from a string is by utilizing the substring
method. Here's how you can do it:
public String removeLastCharacter(String str) {
if (!str.isEmpty()) {
str = str.substring(0, str.length() - 1);
}
return str;
}
By using substring(0, str.length() - 1)
, we can extract the substring from index 0
to the second-to-last character, effectively removing the last character from the string.
To avoid potential errors when the string is empty, we added a check using the isEmpty
method to return the original string without any modifications. Safety first! 🔒
Solution 2: Using StringBuilder 💪
Another approach to removing the last character is by utilizing the StringBuilder
class. Here's how you can do it:
public String removeLastCharacter(String str) {
if (!str.isEmpty()) {
StringBuilder sb = new StringBuilder(str);
sb.deleteCharAt(str.length() - 1);
str = sb.toString();
}
return str;
}
Using a StringBuilder
allows us to manipulate the string efficiently. We create a StringBuilder
object with the input string, then use deleteCharAt
to remove the last character at index str.length() - 1
. Finally, we convert the modified StringBuilder
back to a string using toString()
.
Feel free to choose the solution that suits your coding style and requirements! 🤓
Engage and Share! 🌟
Now that you know how to remove the last character from a string, it's time to put your knowledge into action. Try out the provided solutions in your code, and let us know how it goes in the comments below.
If you found this guide helpful, don't forget to share it with your fellow developers. Together, we can save them from the frustrating pitfalls of removing the last character from a string!
Happy coding! 💻