How to pretty print XML from Java?
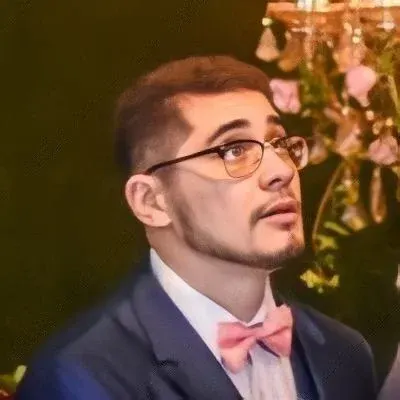
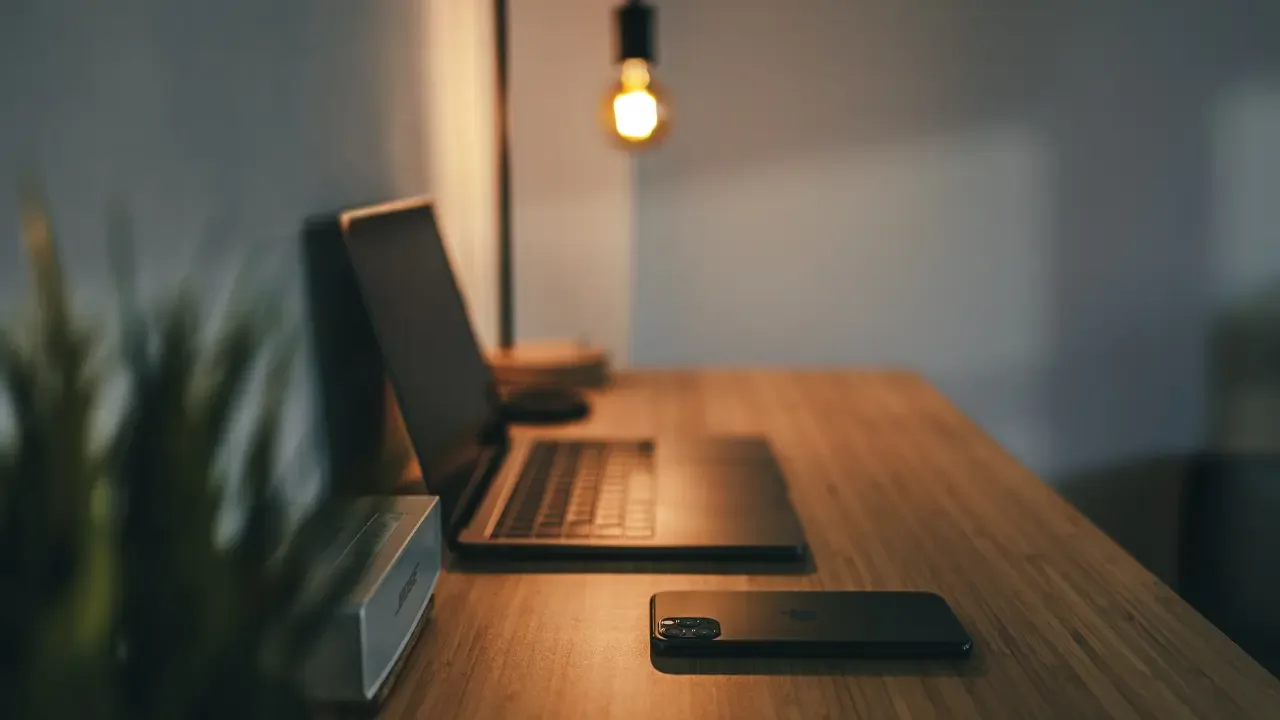
How to Pretty Print XML from Java?
Are you struggling with unformatted XML in your Java code? Do you want to transform it into nicely formatted XML with proper line feeds and indentations? Look no further! In this guide, we'll dive into the common issues faced when trying to pretty print XML from Java and provide you with easy solutions to get your XML looking clean and organized.
The Problem
Let's start with the problem statement. You have a Java String that contains XML but lacks line feeds and indentations. Your goal is to convert this unformatted XML into a well-structured and readable String.
Here's an example of what you might be working with:
String unformattedXml = "<tag><nested>hello</nested></tag>";
The Solution
To achieve pretty printed XML from Java, we'll use a library called org.apache.xml.serializer.OutputPropertiesFactory
. Follow the steps below to format your XML:
Import the required classes:
import org.apache.xml.serializer.OutputPropertiesFactory;
import org.apache.xml.serializer.Serializer;
import org.apache.xml.serializer.SerializerFactory;
Create a method to format the XML string:
public String formatXml(String unformattedXml) throws Exception {
// Create a Serializer instance
Serializer serializer = SerializerFactory.getSerializer(
OutputPropertiesFactory.getDefaultMethodProperties("xml"));
// Prepare the output properties
Properties outputProperties = OutputPropertiesFactory.getDefaultMethodProperties("xml");
outputProperties.setProperty("indent", "yes");
// Set the output properties
serializer.setOutputProperties(outputProperties);
// Create a StringWriter to store the formatted XML
StringWriter stringWriter = new StringWriter();
// Serialize the input XML string into the StringWriter
serializer.setWriter(stringWriter);
serializer.transform(new StreamSource(new StringReader(unformattedXml)), null);
// Return the formatted XML as a String
return stringWriter.toString();
}
Call the
formatXml
method with your unformatted XML:
String unformattedXml = "<tag><nested>hello</nested></tag>";
String formattedXml = formatXml(unformattedXml);
Voila! You now have a
formattedXml
String with nicely formatted XML ready to use.
The Result
Applying the steps mentioned above to our example XML, here's the formatted XML you'll get:
<?xml version="1.0" encoding="UTF-8"?>
<root>
<tag>
<nested>hello</nested>
</tag>
</root>
Take It Further
Once you have successfully formatted your XML, you might want to explore additional enhancements:
Externalize XML: If you are frequently working with XML, it might be beneficial to externalize it into separate XML files. This can improve code readability, maintainability, and enable easy updates to the XML content without modifying your Java code.
Validate XML: If you want to ensure that the XML you are working with is valid, you can use XML Schema Definition (XSD) files to validate the structure and data of your XML.
XML Manipulation: If you need to perform more complex operations on your XML, such as parsing, modifying, or extracting data, consider using XML parsing libraries like DOM or SAX provided by Java. These libraries offer powerful features for XML manipulation.
Your Turn!
Now that you have learned how to pretty print XML from Java, it's time to put your skills to the test. Take a moment to apply this knowledge to your own projects and share your experience in the comments below. If you have any questions or suggestions, we'd love to hear from you!
Happy coding! 🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
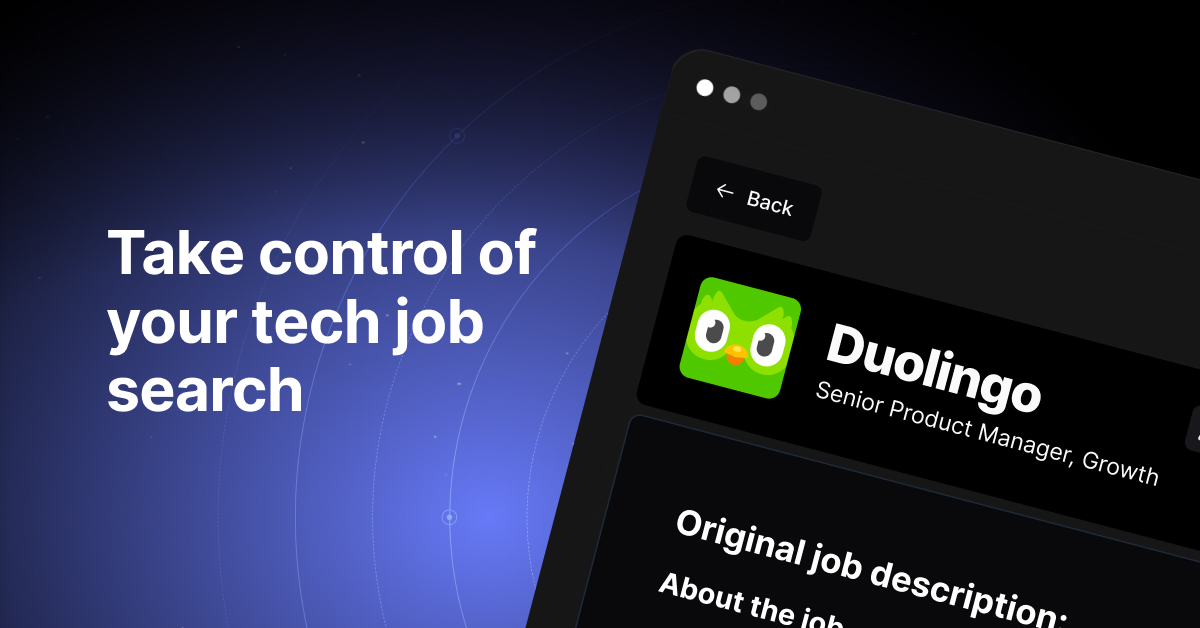