How to POST form data with Spring RestTemplate?
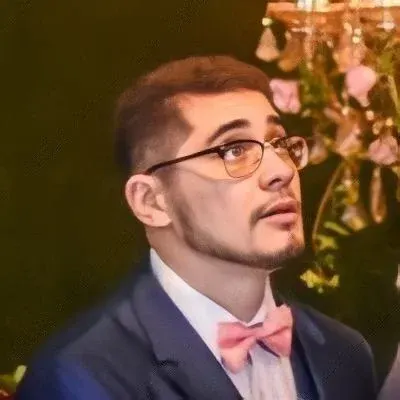
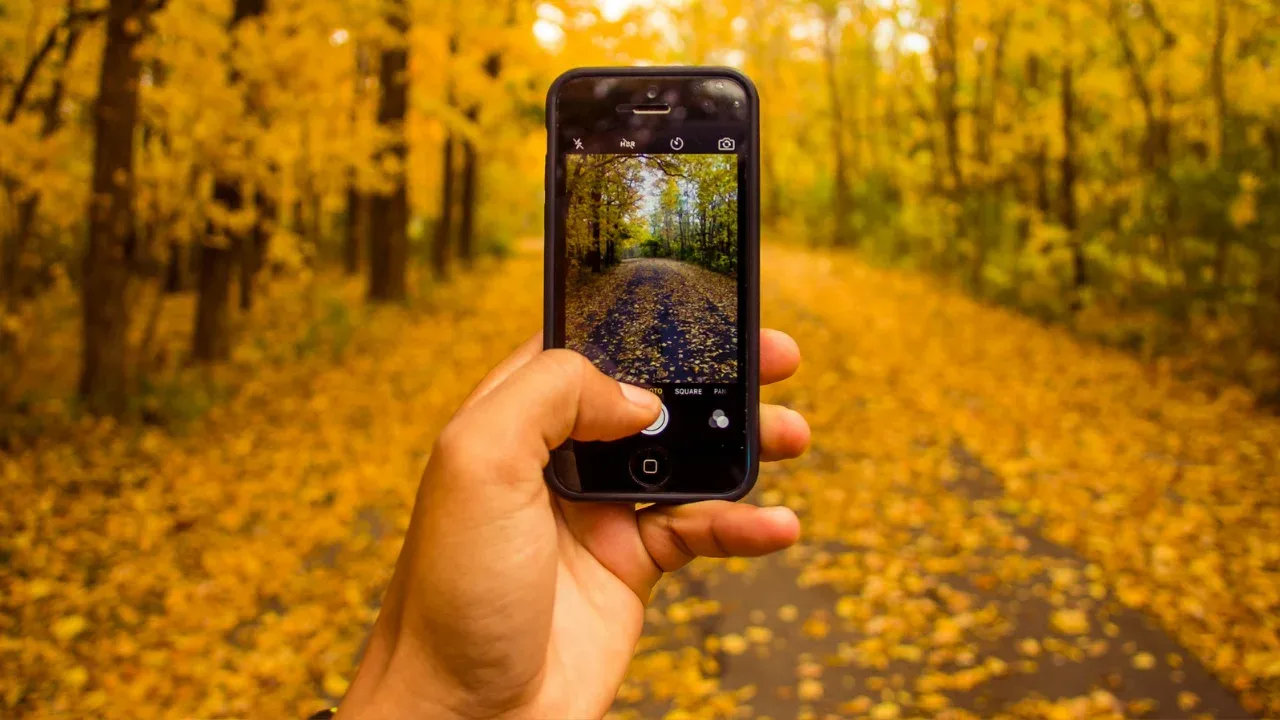
šš» Tech Blog
š„š„ Title: How to POST form data with Spring RestTemplate: Easy Solutions for Common Issues š„š„
Hey there tech enthusiasts! š Are you struggling with how to properly pass form data using Spring RestTemplate? Look no further! In this guide, we will address a common issue faced by many developers and provide you with easy and effective solutions. Let's dive in! šŖ
š” The Problem:
Let's start off by understanding the problem at hand. One of our awesome readers, let's call them "Tech Guru," had successfully used the following curl command to post form data to a specific URL:
curl -i -X POST -d "email=first.last@example.com" https://app.example.com/hr/email
But, when they tried to convert this curl snippet into a RestTemplate call in their Spring application, it resulted in a frustrating 404 Not Found response. š«
Here's the code snippet Tech Guru tried:
String url = "https://app.example.com/hr/email";
Map<String, String> params = new HashMap<String, String>();
params.put("email", "first.last@example.com");
RestTemplate restTemplate = new RestTemplate();
ResponseEntity<String> response = restTemplate.postForEntity(url, params, String.class);
Tech Guru also tried formulating the correct call in Postman, where they managed to get it working by specifying the email parameter as a "form-data" parameter in the body. But how can we achieve this functionality using RestTemplate? š¤
š The Solution:
To properly pass form data using RestTemplate, we need to make a few adjustments. Instead of sending the entire map of parameters, we need to use the MultiValueMap
class provided by Spring. This class is designed specifically for HTTP form parameters and headers. Let's update our code snippet:
String url = "https://app.example.com/hr/email";
MultiValueMap<String, String> params = new LinkedMultiValueMap<>();
params.add("email", "first.last@example.com");
RestTemplate restTemplate = new RestTemplate();
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_FORM_URLENCODED);
HttpEntity<MultiValueMap<String, String>> requestEntity = new HttpEntity<>(params, headers);
ResponseEntity<String> response = restTemplate.postForEntity(url, requestEntity, String.class);
Here's what we did differently:
We replaced the regular
HashMap
with aLinkedMultiValueMap
fromorg.springframework.util
package. This allows us to properly handle form parameters.We initialized
HttpHeaders
to set the Content-Type asapplication/x-www-form-urlencoded
. This tells the server that we are sending form data.We created an
HttpEntity
with theparams
andheaders
, representing the request body and headers respectively.Finally, we use
restTemplate.postForEntity
to perform the POST request, passing theurl
,requestEntity
, and the expected response type.
š You Did It!
Congratulations, you've successfully learned how to POST form data with Spring RestTemplate! š Now you can confidently integrate your Spring application with APIs that require form data submission. š
We hope this guide was helpful in solving your problem. If you have any more questions or face any other issues, feel free to drop a comment below. We'd love to assist you further! š
š£š£ Your Turn!
Now it's your turn to put your newfound knowledge into practice. Try implementing the updated code snippet in your project and let us know how it worked for you. Feel free to share your success stories or any roadblocks you encountered. We can't wait to hear from you! š
Keep exploring, keep learning, and stay tuned for more exciting tech tips and tricks!
Happy coding! š»š
--
āļø Tech Blogger | š YourTechBlog.com | āļø contact@yourtechblog.com
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
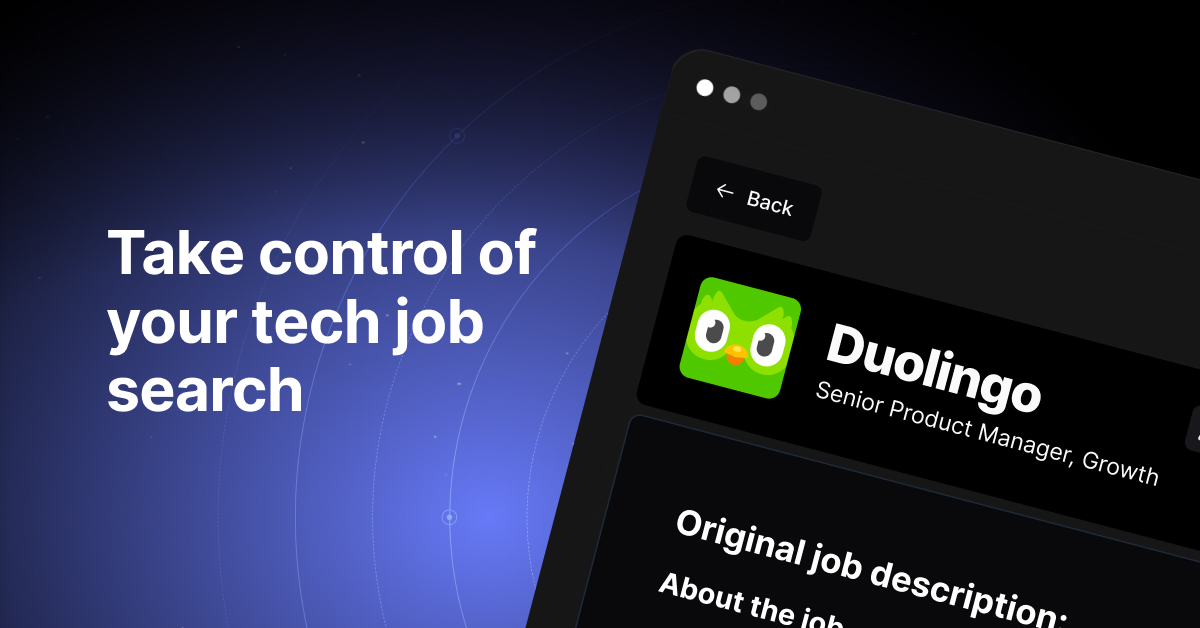