How to pass an object from one activity to another on Android
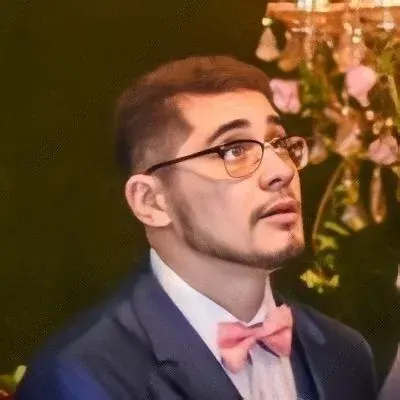
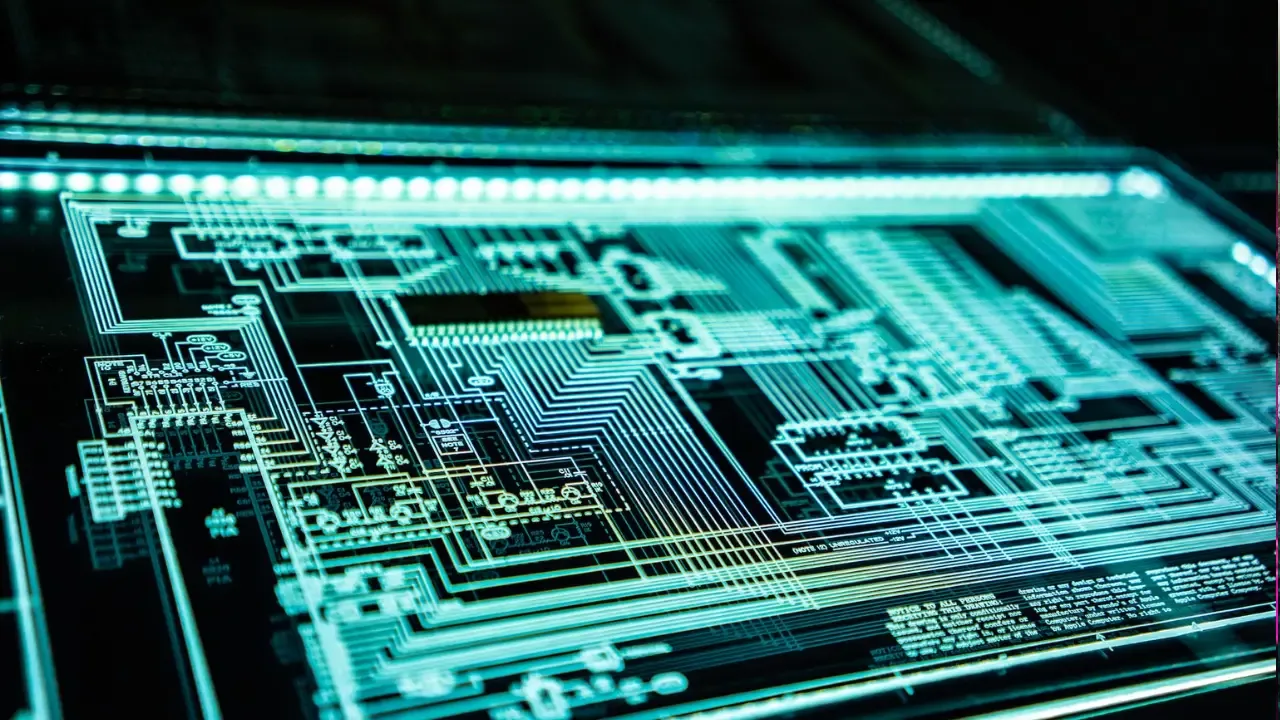
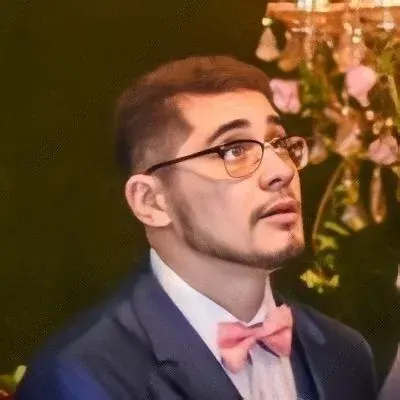
How to Pass an Object from One Activity to Another on Android 📱
Have you ever wondered how to send an object from one activity to another in your Android app? 🤔 It can be quite a common requirement when you want to share data between different screens or components of your application. In this blog post, we'll explore an easy solution to this challenge and help you achieve your goal effortlessly! 🚀
The Scenario 🌴
Let's consider a scenario where you want to pass an object of your Customer
class from Activity A to Activity B. The Customer
class has properties like firstName
, lastName
, age
, and address
, and a method called printValues()
that returns a formatted string with all the customer details. 🏢
public class Customer {
private String firstName;
private String lastName;
private String address;
private int age;
public Customer(String fname, String lname, int age, String address) {
firstName = fname;
lastName = lname;
age = age;
address = address;
}
public String printValues() {
String data = "First Name: " + firstName + " Last Name: " + lastName +
" Age: " + age + " Address: " + address;
return data;
}
}
Your goal is to send the Customer
object from Activity A to Activity B and display the customer details on the second screen. 📝
Solution: Using Intent Extras 📦
To pass objects between activities in Android, you can use the putExtra()
method of the Intent
class. This method allows you to add additional data to the intent and transfer it to the destination activity. 📲
Here's how you can achieve this:
In Activity A:
// Create a new Intent
Intent intent = new Intent(ActivityA.this, ActivityB.class);
// Create a Customer object
Customer customer = new Customer("John", "Doe", 25, "123 Main St");
// Pass the Customer object as an extra
intent.putExtra("customer", customer);
// Start Activity B
startActivity(intent);
In Activity B:
// Retrieve the Customer object from the intent extras
Customer customer = (Customer) getIntent().getSerializableExtra("customer");
// Display the customer details using the printValues() method
String customerDetails = customer.printValues();
textView.setText(customerDetails);
That's it! 🎉 By following these simple steps, you can now effortlessly pass an object from one activity to another and display the data on the receiving activity.
Common Issues and Troubleshooting 🛠️
Issue 1: Serialization
Make sure your Customer
class implements the Serializable
interface. This is required for objects to be passed through intents.
public class Customer implements Serializable {
// Your class code
}
Issue 2: Null Objects
Double-check that the object you're passing is not null. If it is null, you may encounter a NullPointerException in the receiving activity.
Issue 3: Different Packages
If your activities are in different packages or modules, make sure both activities are in the same import statement or provide the fully qualified class name when creating the intent.
Your Turn to Shine! 🌟
Now that you have learned how to pass objects between activities in Android, it's time for you to give it a try! Implement this feature in your own app and see how smoothly the data flows between screens. Don't forget to share your experience with us in the comments section below. We would love to hear from you! 😄
Feel free to reach out if you have any questions or encounter any issues along the way. We're here to assist you. Happy coding! 💻
\uD83D\uDC49 Don't forget to share this blog post with your fellow Android developers and spread the knowledge!