How to parse JSON in Java
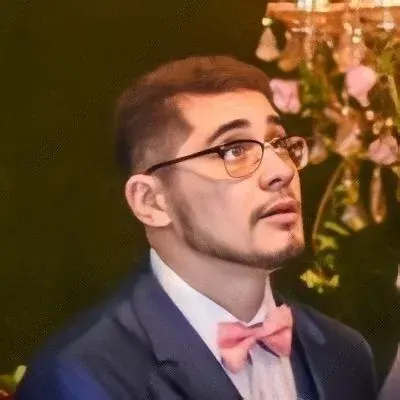
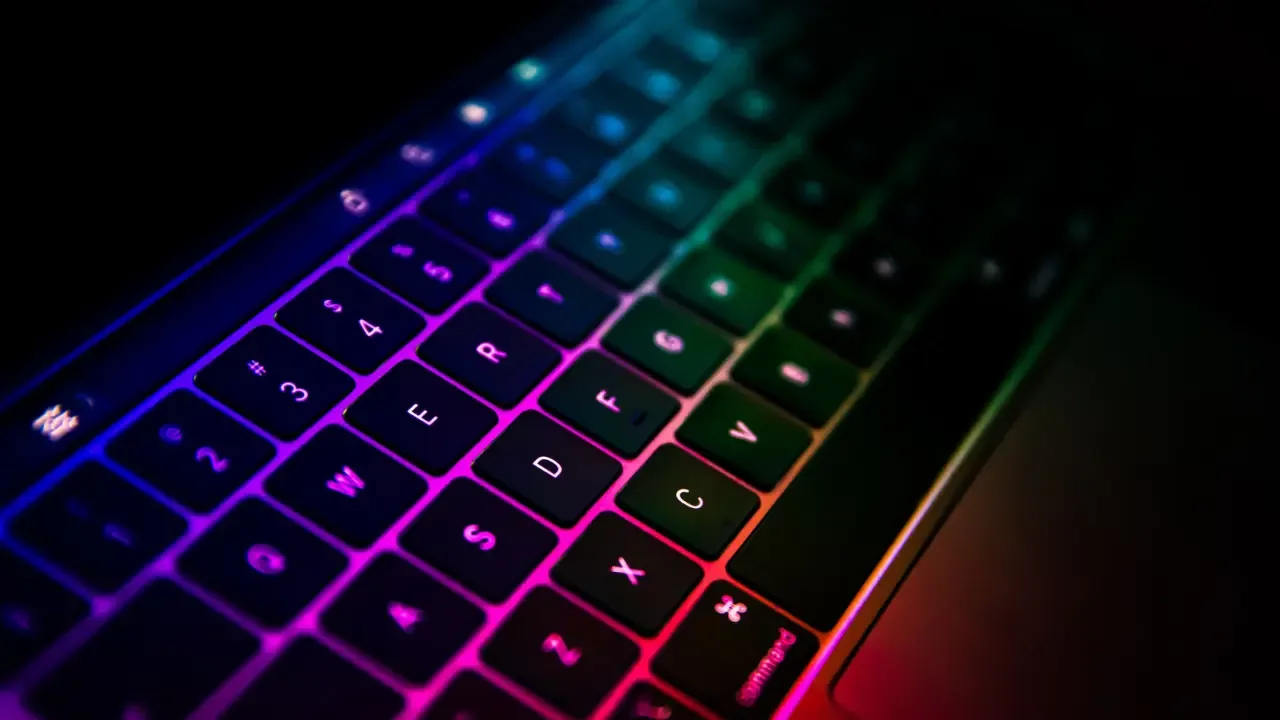
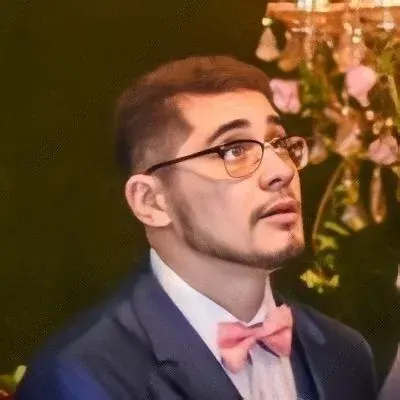
How to Parse JSON in Java: A Beginnerβs Guide π
Are you wondering how to extract specific values from a JSON text using Java? You're in the right place! Parsing JSON in Java might seem a bit challenging at first, but fear not. In this guide, we'll walk you through the process step by step. By the end, you'll be a JSON parsing pro! π
Understanding the Problem π€
Let's start by understanding the context. You have a JSON text that looks like this:
{
"pageInfo": {
"pageName": "abc",
"pagePic": "http://example.com/content.jpg"
},
"posts": [
{
"post_id": "123456789012_123456789012",
"actor_id": "1234567890",
"picOfPersonWhoPosted": "http://example.com/photo.jpg",
"nameOfPersonWhoPosted": "Jane Doe",
"message": "Sounds cool. Can't wait to see it!",
"likesCount": "2",
"comments": [],
"timeOfPost": "1234567890"
}
]
}
And you want to extract the values of pageName
, pagePic
, post_id
, and other relevant properties. Let's dive into the solutions! π‘
Solution 1: Using a JSON Library π
One of the easiest ways to parse JSON in Java is by using a JSON library. There are several popular libraries available, such as:
In this example, we'll use the Gson library. First, you need to include the Gson library in your project:
implementation 'com.google.code.gson:gson:2.8.8'
Once you have Gson added to your project, follow these steps to parse the JSON text and extract the desired values:
Import the necessary Gson classes:
import com.google.gson.Gson;
import com.google.gson.JsonObject;
Parse the JSON text and convert it into a JsonObject:
String jsonText = "{...}" // Replace with your JSON text
JsonObject jsonObject = new Gson().fromJson(jsonText, JsonObject.class);
Retrieve the values of the desired properties using the
get()
method:
String pageName = jsonObject.getAsJsonObject("pageInfo").get("pageName").getAsString();
String pagePic = jsonObject.getAsJsonObject("pageInfo").get("pagePic").getAsString();
String postId = jsonObject.getAsJsonArray("posts").get(0).getAsJsonObject().get("post_id").getAsString();
// Add more properties as needed
And that's it! You have successfully parsed the JSON and extracted the required values. Nice job! π
Solution 2: Using the Native JSON Library in Java 11+ π
If you're using Java 11 or later, parsing JSON became even easier with the introduction of the native JSON library, javax.json
. Here's how you can use it:
Import the necessary classes:
import javax.json.Json;
import javax.json.JsonObject;
Parse the JSON text and convert it into a JsonObject:
String jsonText = "{...}" // Replace with your JSON text
JsonObject jsonObject = Json.createReader(new StringReader(jsonText)).readObject();
Retrieve the values of the desired properties similar to Solution 1:
String pageName = jsonObject.getJsonObject("pageInfo").getString("pageName");
String pagePic = jsonObject.getJsonObject("pageInfo").getString("pagePic");
String postId = jsonObject.getJsonArray("posts").getJsonObject(0).getString("post_id");
// Add more properties as needed
This solution provides a simpler and more streamlined approach if you're using Java 11 or later. π
Conclusion and Call-to-Action π£
Parsing JSON in Java doesn't have to be complicated. By leveraging JSON libraries or the native JSON library in Java 11+, you can easily extract values from a JSON text. Now, it's your turn to give it a try! β¨
If you found this guide helpful, share it with your fellow developers. And, if you have any questions or need further assistance, leave a comment below. We're here to help! π