How to parameterize @Scheduled(fixedDelay) with Spring 3.0 expression language?
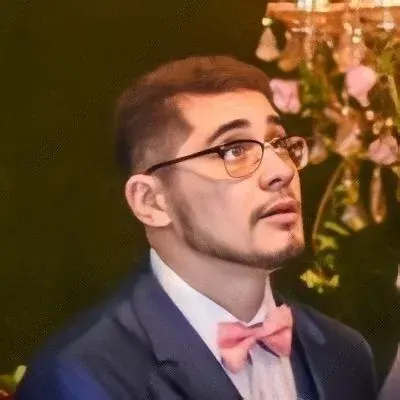
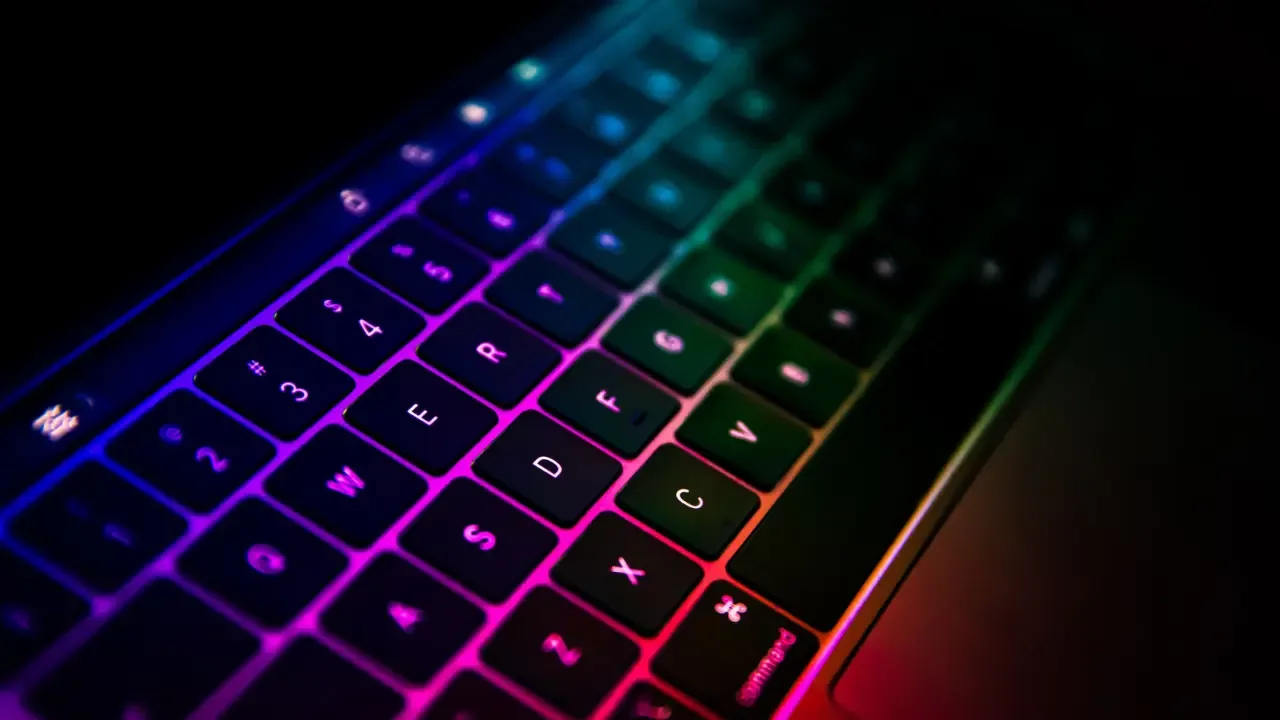
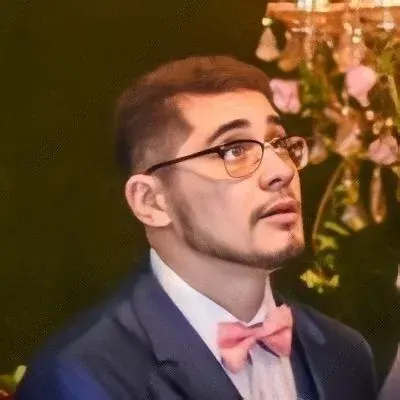
📝 How to Parameterize @Scheduled(fixedDelay) with Spring 3.0 Expression Language?
Do you want to set the fixedDelay
parameter of the @Scheduled
annotation in Spring 3.0 from your configuration file instead of hard-wiring it into your task class? 🤔
Currently, the fixedDelay
parameter requires a long value, but when using the Spring Expression Language (SpEL), the @Value
annotation returns a String object, which cannot be directly auto-boxed to a long value. 😕
But don't worry! I'm here to guide you through the process of parameterizing @Scheduled(fixedDelay)
using SpEL in Spring 3.0. Let's dive in! 💪
First, to parameterize the fixedDelay
value, you need to create a properties file in your Spring application's configuration folder. Let's name it app.properties
. 📄
In app.properties
, define your desired fixedDelay
value as a long integer. For example:
fixed.delay=5000
Next, let's configure Spring to load this properties file. In your Spring configuration file (usually applicationContext.xml
), add the following lines:
<context:property-placeholder location="classpath:app.properties" />
This configuration allows Spring to load the app.properties
file from the classpath.
Now, we can use the parameterized value in the @Scheduled
annotation using SpEL and the @Value
annotation. Update your task class as follows:
@Scheduled(fixedDelayString = "${fixed.delay}")
public void readLog() {
// Your task logic goes here
}
Here, we use the fixedDelayString
attribute instead of fixedDelay
. The fixedDelayString
attribute expects a String value, which allows us to use SpEL with the @Value
annotation.
By specifying ${fixed.delay}
as the value for fixedDelayString
, Spring will resolve the value from the app.properties
file and use it as the delay for your scheduled task.
🔥 Pro Tip: You can also use the fixedDelayString
attribute to specify an expression using SpEL. For example, if you want the delay to be calculated dynamically at runtime, you can use ${#{T(java.lang.Math).random() * 5000}}
, which generates a random delay between 0 and 5000 milliseconds.
And that's it! 🎉 You have successfully parameterized the @Scheduled(fixedDelay)
by leveraging the power of SpEL and the @Value
annotation in Spring 3.0. Now you can easily configure your scheduled tasks from a properties file, making your application more flexible and maintainable. 🚀
If you found this guide helpful, make sure to share it with your fellow developers, and let me know in the comments if you have any questions or other Spring-related topics you'd like to learn more about. Happy coding! 💻💡
📣 CALL TO ACTION: Want to learn more about Spring and explore other advanced topics? Check out our blog for more insightful articles and tutorials! Join our community of passionate developers and stay up-to-date with the latest trends and technologies. Don't miss out, subscribe now! 💌👇