How to nicely format floating numbers to string without unnecessary decimal 0"s
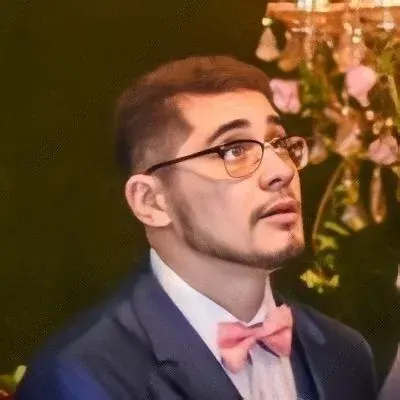
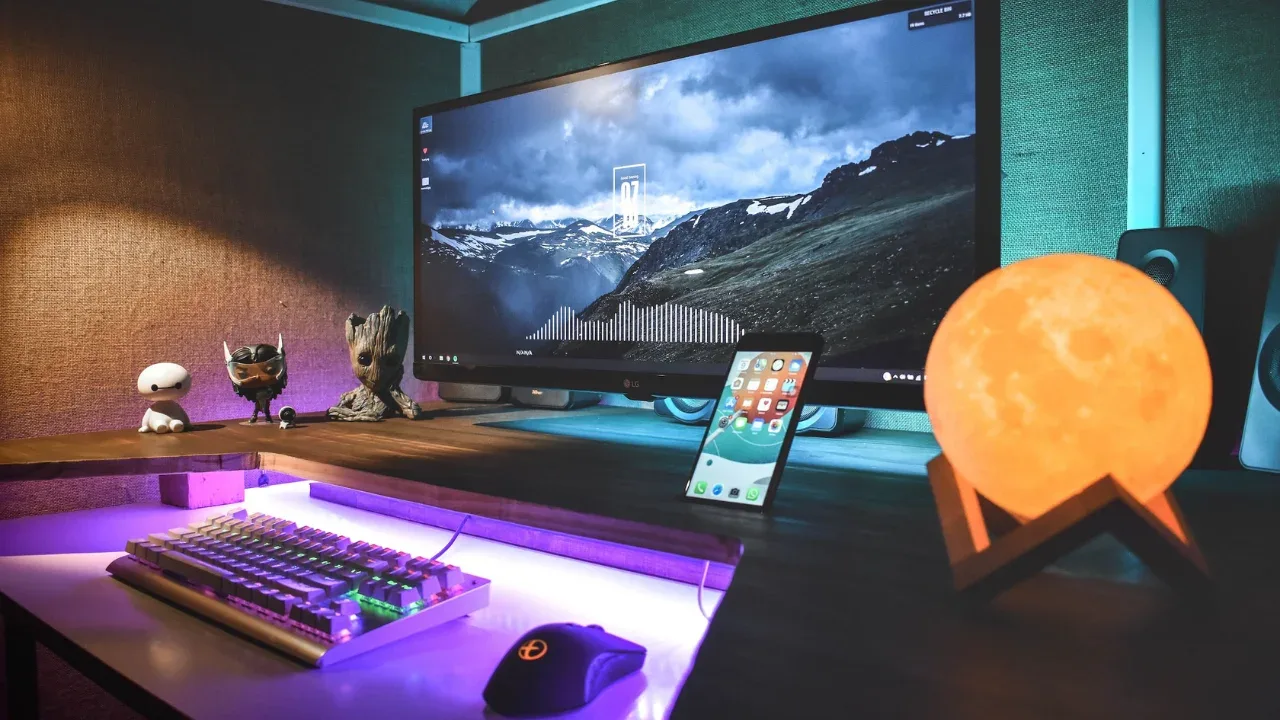
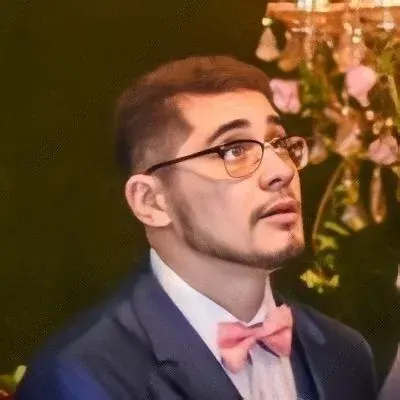
Nice Formatting for Floating Numbers in Java: Say Goodbye to Unnecessary Decimal 0's! ๐๐ฏ
Are you tired of dealing with pesky trailing zeros when you print floating numbers in Java? Don't worry, I've got your back! In this guide, I'll show you how to nicely format floating numbers to strings without those annoying decimal 0's. No more compromising on performance โ we'll find a smart solution for you! ๐ช๐
The Double Dilemma: Mixing Pseudo Integers with Actual Doubles ๐ฑ
Let's set the stage for our problem. We have a situation where we are working with 64-bit doubles to represent integers. Since a 64-bit double can accurately represent integer values up to +/- 2^53, it seems like a good fit for our needs. However, we encounter a challenge when we need to print these numbers. Our pseudointegers are mixed with actual doubles, creating confusion. ๐ซ
String Formatting to the Rescue! Format It Right! โ๏ธ๐ก
To tackle this problem, we initially try using String.format("%f", value)
, which seems like an appropriate solution. But alas, we face another annoyance โ trailing zeros for small values! Here's an example of the unwanted output we get:
232.00000000
0.18000000000
1237875192.0
4.5800000000
0.00000000
1.23450000
I Want My Numbers Clean: Removing Unnecessary Decimal 0's โ๏ธ๐ฏ
The desired output looks much cleaner, doesn't it? Here's what we want:
232
0.18
1237875192
4.58
0
1.2345
While we could write a function to trim those trailing zeros, it could lead to performance loss due to unnecessary string manipulation. So, rather than taking that route, let's explore other format codes that could be more efficient. Is there a better way? You bet! ๐
Introducing DecimalFormat: The Precision Cleanup Crew! ๐งนโจ
Say hello to DecimalFormat
โ our precision cleanup crew! With DecimalFormat
, we can easily format our numbers without worrying about performance overhead. Here's how we can use it:
import java.text.DecimalFormat;
public class CleanNumberPrinter {
public static void main(String[] args) {
DecimalFormat formatter = new DecimalFormat("#.##");
double value = 1.2345;
System.out.println(formatter.format(value));
}
}
Output:
1.23
But What About Locale Dependency? ๐๐
Hold on a second! Before you start using DecimalFormat
everywhere, it's vital to consider its locale dependency. Keep in mind that String.format(format, args...)
is locale-dependent. So, if you're dealing with internationalization and localization, you might need to make additional adjustments.
Let's Hear from the Experts! ๐จโ๐ฌ๐ฉโ๐ฌ
In the comments section, there were a couple of answers that suggested rounding to two decimal places, but they failed to address the problem correctly. It's crucial to understand the issue before jumping to conclusions. So, please use our solution using DecimalFormat
for accurate and clean results. ๐
Engage with Our Tech Community! ๐๐ข
We hope this guide helped you conquer the challenges of formatting floating numbers in Java! Feel free to share your thoughts, experiences, or alternative solutions in the comments below. Join our tech community and be part of the conversation! Don't forget to smash that like and share button to spread the knowledge! ๐๐ฅ
Together, we can code brilliantly! Happy formatting! ๐๐ป