How to mock void methods with Mockito
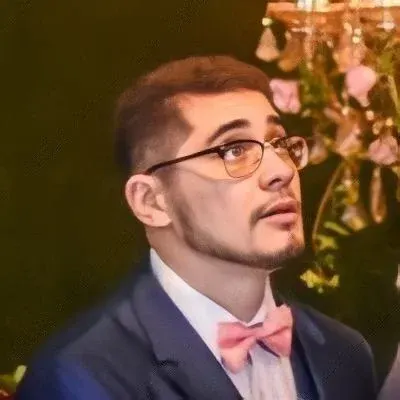
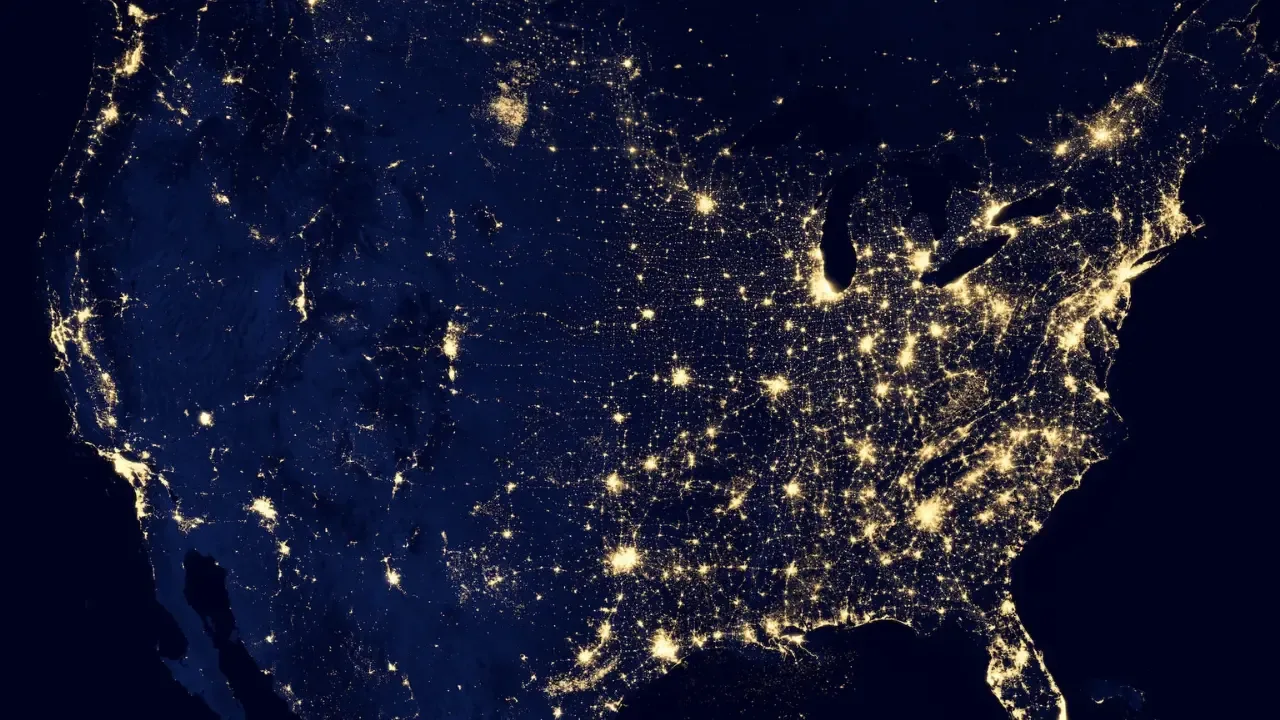
📝🤔 How to mock void methods with Mockito? Let's solve this tricky problem! 💪
Hey there tech enthusiasts! 😎 Are you struggling with mocking void methods 🔇 using Mockito? 🤔 Don't worry, you're not alone! Many developers face this challenge, but worry not, because in this blog post, we'll guide you on how to overcome this hurdle and mock void methods like a pro! 💻🔥
So here's the story: A fellow developer was trying to implement an observer pattern but faced difficulties in mocking it with Mockito. The class structure looked like this:
public class World {
List<Listener> listeners;
void addListener(Listener item) {
listeners.add(item);
}
void doAction(Action goal, Object obj) {
setState("I received");
goal.doAction(obj);
setState("I finished");
}
private String state;
// Setter and getter for state
}
public class WorldTest implements Listener {
@Test
public void word() {
World w = mock(World.class);
w.addListener(this);
// ...
// ...
}
}
interface Listener {
void doAction();
}
As you can see, the developer was unable to trigger the system with mock. The goal was to show the system state mentioned above and make assertions based on them. So, let's dive in and find a solution! 💡
The Solution: Void Methods and Mockito
To mock void methods with Mockito, we can make use of the doAnswer()
method provided by the Mockito framework. This method allows us to define custom behavior when a mocked void method is called.
In our example, let's say we want to mock the doAction()
method in the World
class. We can do it like this:
@Test
public void testDoAction() {
World world = mock(World.class);
doAnswer(invocation -> {
Object arg0 = invocation.getArgument(0);
Object arg1 = invocation.getArgument(1);
// Custom logic here
// Perform assertions based on the arguments
return null; // Since the method is void, we just return null
}).when(world).doAction(any(Action.class), any(Object.class));
// ... Perform test and assertions
}
By using the doAnswer()
method, we can capture the arguments passed to the doAction()
method and perform our custom logic, such as making assertions or updating the system state.
Wrap-up and Share Your Thoughts!
And there you have it! Now you know how to mock void methods with Mockito! 🙌 We leveraged the doAnswer()
method to define custom behavior for void methods within Mockito, allowing us to make assertions and capture the arguments passed.
We hope this guide was helpful and solved your problem. If you still have any questions or want to explore another topic, feel free to leave a comment below! Let's support and learn from each other and make the tech community stronger! 👏💬
[Insert your compelling call-to-action here, encouraging readers to share their thoughts, provide feedback, or suggest more topics to cover in future blog posts.]
So, until next time, happy coding! 🚀✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
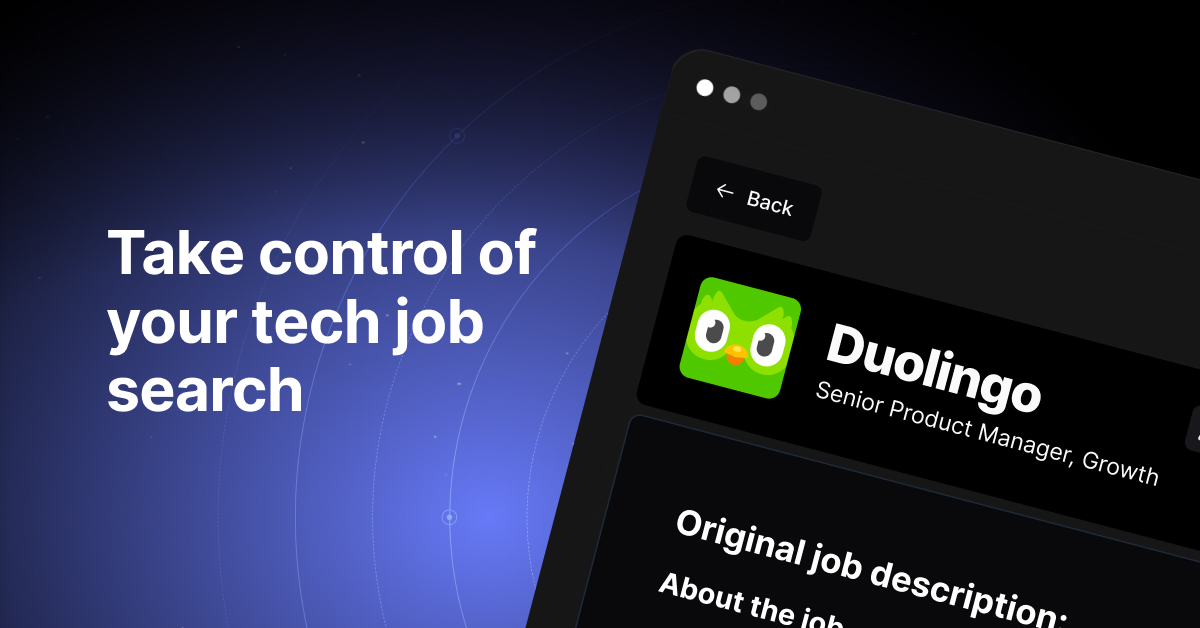