How to manage REST API versioning with spring?
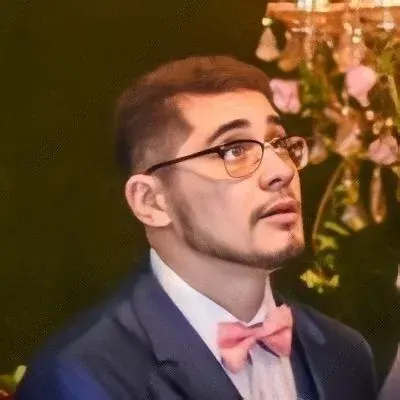
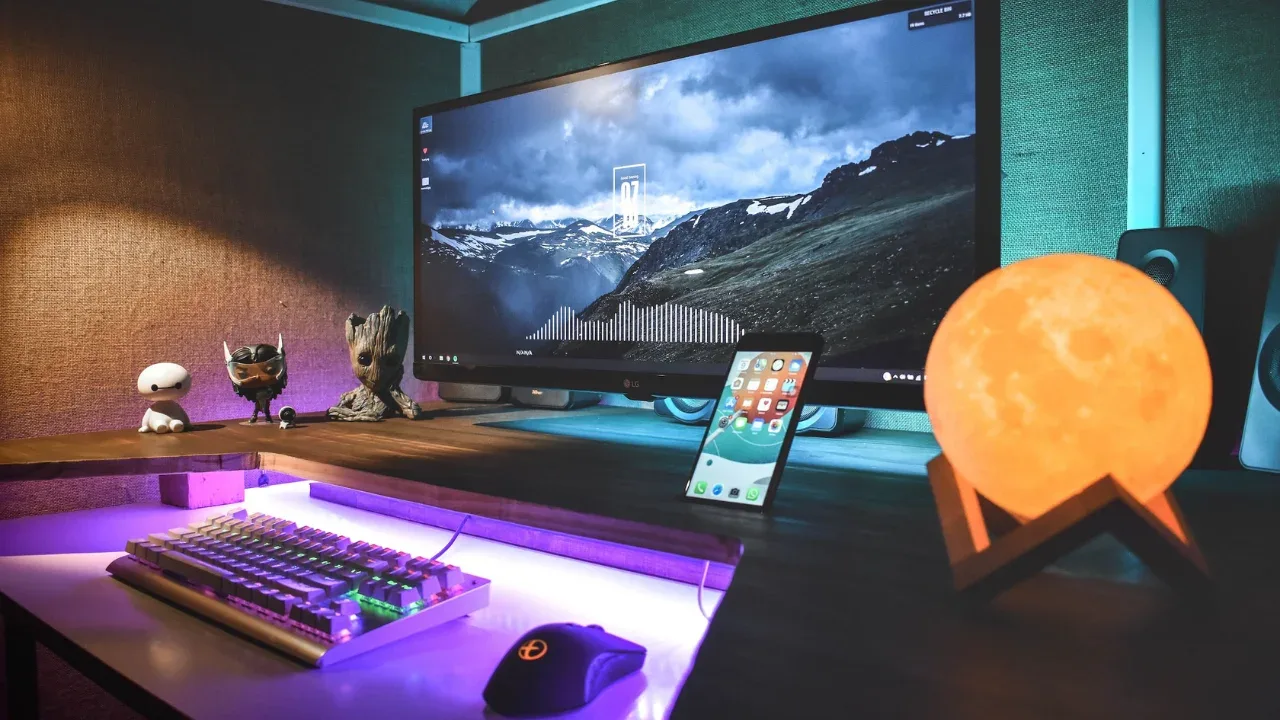
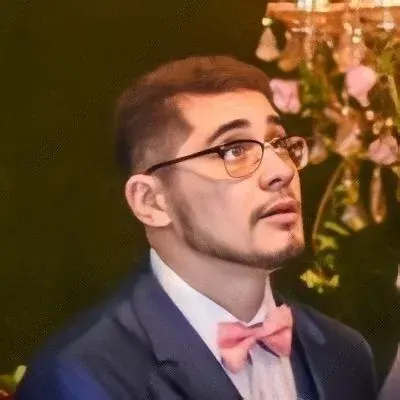
How to Manage REST API Versioning with Spring
Are you struggling to find an easy and maintainable way to manage REST API versioning using Spring? Don't worry, we've got you covered! In this blog post, we'll walk you through a common issue faced by developers and provide you with an easy solution. Let's dive in! 💪🌟
The Problem: Versioning Based on the Accept Header
Imagine you have a REST API with multiple versions, and you want to handle each version based on the Accept header. For example, if a request has the Accept header application/vnd.company.app-1.1+json
, you want Spring MVC to forward this request to the corresponding version-specific method.
The challenge here is that not all methods in an API change in the same release. Therefore, you don't want to modify each controller for handlers that haven't changed between versions. Additionally, you don't want to clutter your controllers with logic to determine the appropriate version, as Spring already handles method selection based on request mapping.
The Solution: Custom Annotation with Version Ranges
To address this problem, we can create a custom annotation to define version ranges for each method. Let's call this annotation @VersionRange
.
🔹 Step 1: Define the Custom Annotation
Create a custom annotation, @VersionRange
, which can define an open or closed version range. For example:
@Target(ElementType.METHOD)
@Retention(RetentionPolicy.RUNTIME)
public @interface VersionRange {
double from() default 0.0;
double to() default Double.POSITIVE_INFINITY;
}
In the above code, we define the @VersionRange
annotation with from()
and to()
attributes to specify the version range.
🔹 Step 2: Apply the Custom Annotation
Apply the @VersionRange
annotation to your version-specific methods in your controller. For example:
@RequestMapping(...)
@VersionRange(from = 1.0, to = 1.6)
@ResponseBody
public Object method1() {
// do something
return object;
}
@RequestMapping(...)
@VersionRange(from = 1.7)
@ResponseBody
public Object method2() {
// do something
return object;
}
In the code snippet above, we have applied the @VersionRange
annotation to two methods. The first method is valid for versions 1.0 to 1.6, while the second method is valid for version 1.7 onwards.
Implementation Approach
Now, let's discuss how we can implement this approach in Spring.
🔸 Option 1: Tinkering with Request Mapping Types
You can write your own ProducesRequestCondition
to match handlers to requests based on the version range defined in the @VersionRange
annotation. This approach allows you to specify version ranges directly in the produces
part of the @RequestMapping
annotation.
However, this approach requires replacing some core Spring MVC classes like RequestMappingInfoHandlerMapping
, RequestMappingHandlerMapping
, and RequestMappingInfo
. This might create extra work whenever you upgrade to a newer version of Spring, which is not ideal.
🔸 Option 2: Using a Third-Party Library Alternatively, you can leverage existing third-party libraries, such as Spring Cloud Gateway or Spring Boot Actuator, that provide built-in support for API versioning. These libraries offer more flexibility and maintainability without the need for extensive customizations.
Conclusion and Call-to-Action
Managing REST API versioning with Spring can be challenging, but with the right approach, it becomes much simpler. By using a custom annotation like @VersionRange
and applying it to your version-specific methods, you can achieve version-based routing without cluttering your controllers.
Give this approach a try, and let us know your thoughts! Do you find it easier to maintain? Share your experience with us in the comments below. Don't forget to check out the GitHub repository for the POC (Proof of Concept) shared by the author here.
If you have any further questions or suggestions on how to handle API versioning in a simpler way, feel free to reach out and share your insights. Happy coding! 🚀👩💻👨💻